Webhooks
ChargeOver supports utilizing "web hooks" to notify external, 3rd-party applications of events/changes occurring within ChargeOver.
Whenever an event occurs in ChargeOver, a webhook can be triggered -- a HTTP/HTTPS request will be made to a user-specified URL with data about the event/change that occurred.
Webhooks Best Practices:
- DO return a
200 OK
HTTP response if you received and processed the data successfully - DO return a non-
200 OK
header if you did not receive the data successfully and need ChargeOver to retry delivery - DO log the webhooks on your server so you can debug/troubleshoot if required
Restrictions:
- Only
https://
URLs are allowed - Non-standard ports are not accepted (i.e. only ports 80 and ports 443 are allowed)
- Your webhook must use a resolvable public domain name (you may not use just an IP address)
- ChargeOver will send you a HTTP
POST
request - The Content-Type will be
application/json
Helpful Tools/Tips:
- If you return a non-
200 OK
response to ChargeOver, the payload will be logged and viewable in theWebhook Log
report in the Report Center in your ChargeOver account. This can be useful for debugging/to capture payloads for troubleshooting. - You may consider using ngrok, which provides you with a UI that makes it easy to inspect all of your HTTP responses and requests and replay webhook requests.
Enabling webhooks
There are two ways to enable webhooks in ChargeOver:
Enabling webhooks in the user interface
You can enable webhooks directly in the ChargeOver user interface.
- Log in to ChargeOver, and click the settings gear/cog in the top right
- Choose "Developer" from the left-side navigation menu
- Choose "Webhooks"
- Enable Webhooks
- Click the "New Webhook" button and enter the URL to send webhooks to
- Give your webhook a nickname (optional)
- Select the desired events to listen for
- Click the save button to save the configuration
Enabling webhooks programatically
If you need your application to automatically subscribe to webhooks, without depending on any human interaction, you can do that via our Rest Hooks implementation.
Filtering events
Tailor your webhooks to your specific needs by choosing the events that you want to be notified about.
For example, if you're using ChargeOver to manage subscriptions, selecting which events to listen for allows you to receive only the most important notifications, such as when a customer's subscription is created, updated, or canceled. This helps you stay informed without being overwhelmed by notifications that may not be as relevant to your business.
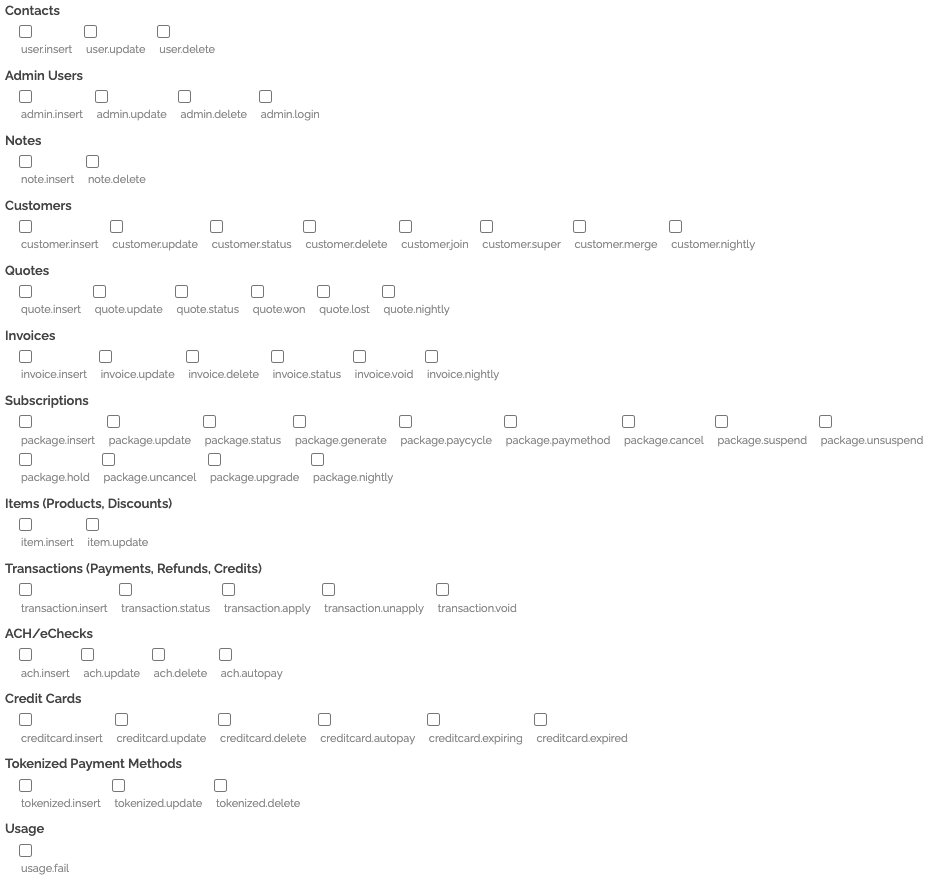
Learn more about specific webhook events supported by ChargeOver.
Dates and times
ChargeOver returns datetime
fields in ISO 8601
format.
For example, October 25th, 2022 at 8:50am Central Time
is represented as:
2022-10-25T08:50:45-05:00
Generally, the timestamps returned will be in America/Chicago
timezone.
When sending date
or datetime
values to ChargeOver, you send dates in a few formats:
YYYY-MM-DD
- a date, example:2022-10-25
YYYY-MM-DD HH:II:SS
- a date/time, example:2022-10-25 08:50:00
YYYY-MM-DDTHH:II:SS-Z
- a date/time with a timezone, example:2022-10-25T08:50:45-05:00
Note: The date/time format and timezone returned by ChargeOver is configurable, so your ChargeOver account may return dates/times in an alternative format or timezone.
Events
ChargeOver webhooks are documented below.
Customers
A customer is created
This event occurs when a new customer is created.
Note these fields:
context_str = customer
context_id = ...
(this will be thecustomer_id
of the new customer)event = insert
A customer is created
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: Ma4hWVUNLx0O5zfmJSjQ3AgwpRdY7qu1
X-Chargeover-Source: example.chargeover.com
{
"context_str": "customer",
"context_id": 160,
"event": "insert",
"data": {
"customer": {
"superuser_id": 370,
"external_key": null,
"token": "lrqtxpimw5vo",
"company": "John Doe's Company, LLC",
"bill_addr1": "72 E Blue Grass Road",
"bill_addr2": "Suite D",
"bill_addr3": null,
"bill_city": "Mt Pleasant",
"bill_state": "MI",
"bill_postcode": "48858",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"currency_id": 1,
"write_datetime": "2014-12-20 12:29:31",
"write_ipaddr": "172.16.4.176",
"mod_datetime": "2014-12-20 12:29:31",
"mod_ipaddr": "172.16.4.176",
"paid": 0,
"total": 0,
"balance": 0,
"url_paymethodlink": "http:\/\/dev.chargeover.com\/signup\/r\/paymethod\/i\/lrqtxpimw5vo",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "John Doe's Company, LLC",
"ship_block": "",
"bill_block": "72 E Blue Grass Road\nSuite D\nMt Pleasant MI 48858\nUnited States",
"superuser_name": "John Doe",
"superuser_first_name": "John",
"superuser_last_name": "Doe",
"superuser_phone": "860-634-1602",
"superuser_email": "johndoe@chargeover.com",
"customer_id": 160
},
"user": {
"user_id": 370,
"external_key": null,
"first_name": "John",
"middle_name_glob": null,
"last_name": "Doe",
"name_suffix": null,
"title": "",
"email": "johndoe@chargeover.com",
"phone": "860-634-1602",
"write_datetime": "2014-12-20 12:29:31",
"mod_datetime": "2014-12-20 12:29:31",
"name": "John Doe",
"display_as": "John Doe",
"username": "johndoe@chargeover.com"
}
},
"security_token": "Ma4hWVUNLx0O5zfmJSjQ3AgwpRdY7qu1"
}
A customer is updated
This event occurs when an existing customer is updated (e.g. the name or address is changed, etc.).
Note these fields:
context_str = customer
context_id = ...
(this will be thecustomer_id
of the customer)event = update
A customer is updated
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: 9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo
X-Chargeover-Source: example.chargeover.com
{
"context_str": "customer",
"context_id": "8",
"event": "update",
"data": {
"customer": {
"superuser_id": 354,
"external_key": null,
"token": "2ygprd9569t4",
"company": "John Doe's Company, LLC",
"bill_addr1": "20 Test Street",
"bill_addr2": "",
"bill_addr3": "",
"bill_city": "City",
"bill_state": "State",
"bill_postcode": "",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"terms_id": 4,
"class_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"admin_id": 3,
"campaign_id": null,
"currency_id": 1,
"language_id": 1,
"brand_id": 1,
"no_taxes": false,
"no_dunning": false,
"write_datetime": "2017-06-08 15:39:59",
"write_ipaddr": "127.0.0.1",
"mod_datetime": "2017-06-15 14:22:01",
"mod_ipaddr": "127.0.0.1",
"terms_name": "Net 45",
"terms_days": 45,
"paid": 10.95,
"total": 82.85,
"balance": 71.9,
"url_paymethodlink": "http:\/\/dev.chargeover.com\/r\/paymethod\/i\/2ygprd9569t4",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/customer\/view\/8",
"admin_name": "Karli Palmer",
"admin_email": "karli@chargeover.com",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "John Doe's Company, LLC",
"ship_block": "",
"bill_block": "20 Test Street\nCity State\nUnited States",
"superuser_name": "John Doe",
"superuser_first_name": "John",
"superuser_last_name": "Doe",
"superuser_phone": "",
"superuser_email": "john@example.com",
"superuser_token": "4e7t9r5a03fv",
"customer_id": 8,
"invoice_delivery": "print",
"dunning_delivery": "email",
"customer_status_id": 1,
"customer_status_name": "Current",
"customer_status_str": "active-current",
"customer_status_state": "a",
"superuser_username": "john@example.com"
},
"user": {
"user_id": 354,
"external_key": null,
"first_name": "John",
"middle_name_glob": null,
"last_name": "Doe",
"name_suffix": null,
"title": "",
"email": "john@example.com",
"token": "4e7t9r5a03fv",
"phone": "",
"user_type_id": 1,
"write_datetime": "2017-06-08 15:39:59",
"mod_datetime": "2017-06-08 15:39:59",
"name": "John Doe",
"display_as": "John Doe",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/contact\/view\/354",
"user_type_name": "Billing",
"username": "john@example.com",
"customer_id": 8
}
},
"security_token": "9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo"
}
A contact is added to the customer
This event occurs when a new contact/user is attached to a customer.
Note these fields:
context_str = customer
context_id = ...
(this will be thecustomer_id
of the customer)event = join
A contact is added to the customer
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: Ma4hWVUNLx0O5zfmJSjQ3AgwpRdY7qu1
X-Chargeover-Source: example.chargeover.com
{
"context_str": "customer",
"context_id": 160,
"event": "join",
"data": {
"user_id": null,
"customer": {
"superuser_id": 370,
"external_key": null,
"token": "lrqtxpimw5vo",
"company": "John Doe's Company, LLC",
"bill_addr1": "72 E Blue Grass Road",
"bill_addr2": "Suite D",
"bill_addr3": null,
"bill_city": "Mt Pleasant",
"bill_state": "MI",
"bill_postcode": "48858",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"currency_id": 1,
"write_datetime": "2014-12-20 12:29:31",
"write_ipaddr": "172.16.4.176",
"mod_datetime": "2014-12-20 12:29:31",
"mod_ipaddr": "172.16.4.176",
"paid": 0,
"total": 0,
"balance": 0,
"url_paymethodlink": "http:\/\/dev.chargeover.com\/signup\/r\/paymethod\/i\/lrqtxpimw5vo",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "John Doe's Company, LLC",
"ship_block": "",
"bill_block": "72 E Blue Grass Road\nSuite D\nMt Pleasant MI 48858\nUnited States",
"superuser_name": "John Doe",
"superuser_first_name": "John",
"superuser_last_name": "Doe",
"superuser_phone": "860-634-1602",
"superuser_email": "johndoe@chargeover.com",
"customer_id": 160
},
"user": {
"user_id": 370,
"external_key": null,
"first_name": "John",
"middle_name_glob": null,
"last_name": "Doe",
"name_suffix": null,
"title": "",
"email": "johndoe@chargeover.com",
"phone": "860-634-1602",
"write_datetime": "2014-12-20 12:29:31",
"mod_datetime": "2014-12-20 12:29:31",
"name": "John Doe",
"display_as": "John Doe",
"username": "johndoe@chargeover.com"
}
},
"security_token": "Ma4hWVUNLx0O5zfmJSjQ3AgwpRdY7qu1"
}
The main contact is changed
This event occurs when the main contact for a customer changes.
Note these fields:
context_str = customer
context_id = ...
(this will be thecustomer_id
of the customer)event = super
The main contact is changed
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: Ma4hWVUNLx0O5zfmJSjQ3AgwpRdY7qu1
X-Chargeover-Source: example.chargeover.com
{
"context_str": "customer",
"context_id": 160,
"event": "super",
"data": {
"user_id": null,
"customer": {
"superuser_id": 370,
"external_key": null,
"token": "lrqtxpimw5vo",
"company": "John Doe's Company, LLC",
"bill_addr1": "72 E Blue Grass Road",
"bill_addr2": "Suite D",
"bill_addr3": null,
"bill_city": "Mt Pleasant",
"bill_state": "MI",
"bill_postcode": "48858",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"currency_id": 1,
"write_datetime": "2014-12-20 12:29:31",
"write_ipaddr": "172.16.4.176",
"mod_datetime": "2014-12-20 12:29:31",
"mod_ipaddr": "172.16.4.176",
"paid": 0,
"total": 0,
"balance": 0,
"url_paymethodlink": "http:\/\/dev.chargeover.com\/signup\/r\/paymethod\/i\/lrqtxpimw5vo",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "John Doe's Company, LLC",
"ship_block": "",
"bill_block": "72 E Blue Grass Road\nSuite D\nMt Pleasant MI 48858\nUnited States",
"superuser_name": "John Doe",
"superuser_first_name": "John",
"superuser_last_name": "Doe",
"superuser_phone": "860-634-1602",
"superuser_email": "johndoe@chargeover.com",
"customer_id": 160
},
"user": {
"user_id": 370,
"external_key": null,
"first_name": "John",
"middle_name_glob": null,
"last_name": "Doe",
"name_suffix": null,
"title": "",
"email": "johndoe@chargeover.com",
"phone": "860-634-1602",
"write_datetime": "2014-12-20 12:29:31",
"mod_datetime": "2014-12-20 12:29:31",
"name": "John Doe",
"display_as": "John Doe",
"username": "johndoe@chargeover.com"
}
},
"security_token": "Ma4hWVUNLx0O5zfmJSjQ3AgwpRdY7qu1"
}
A customer is deleted
This event occurs when an existing customer is deleted.
Note these fields:
context_str = customer
context_id = ...
(this will be thecustomer_id
of the customer)event = delete
A customer is deleted
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: Jy5gsouFvDh3ZwS241xpHbPt0K7MkcTW
X-Chargeover-Source: example.chargeover.com
{
"context_str": "customer",
"context_id": "11",
"event": "delete",
"data": {
"customer": {
"superuser_id": 358,
"external_key": null,
"token": "fhejv4mux605",
"company": "Notable & Co., LLC",
"bill_addr1": "123 Main Street",
"bill_addr2": null,
"bill_addr3": null,
"bill_city": "Mt. Pleasant",
"bill_state": "MI",
"bill_postcode": "48858",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"terms_id": 2,
"class_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"admin_id": 3,
"campaign_id": null,
"currency_id": 1,
"no_taxes": false,
"no_dunning": false,
"write_datetime": "2015-03-13 20:03:49",
"write_ipaddr": "::1",
"mod_datetime": "2015-03-13 20:03:49",
"mod_ipaddr": "::1",
"terms_name": "Net 30",
"terms_days": 30,
"paid": 0,
"total": 0,
"balance": 0,
"url_paymethodlink": "http:\/\/dev.chargeover.com\/r\/paymethod\/i\/fhejv4mux605",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "Notable & Co., LLC",
"ship_block": "",
"bill_block": "123 Main Street\r\nMt. Pleasant MI 48858\r\nUnited States",
"superuser_name": "Jon Doe",
"superuser_first_name": "Jon",
"superuser_last_name": "Doe",
"superuser_phone": "",
"superuser_email": "jondoe@chargeover.com",
"customer_id": 11
},
"user": {
"user_id": 358,
"external_key": null,
"first_name": "Jon",
"middle_name_glob": null,
"last_name": "Doe",
"name_suffix": null,
"title": "",
"email": "jondoe@chargeover.com",
"token": "16czoktfpnm3",
"phone": "",
"write_datetime": "2015-03-13 20:03:49",
"mod_datetime": "2015-03-13 20:03:49",
"name": "Jon Doe",
"display_as": "Jon Doe",
"username": "jondoe@chargeover.com"
}
},
"security_token": "Jy5gsouFvDh3ZwS241xpHbPt0K7MkcTW"
}
The nightly event occurs
This is an event that is kicked off nightly for each customer.
This event only occurs for customer who have had an active subscription, an invoice, a transaction (payment, refund, etc.), or quote within the last 90 days. Customers with no recent activity will not fire this event.
Internally, this event is used for updating caches, consistency checks, scheduled events for customers, etc.
Externally, you can choose to hook into this event and use it for whatever you would like.
Note these fields:
context_str = customer
context_id = ...
(this will be thecustomer_id
of the customer)event = nightly
The nightly event occurs
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: 9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo
X-Chargeover-Source: example.chargeover.com
{
"context_str": "customer",
"context_id": "38",
"event": "nightly",
"data": {
"customer": {
"superuser_id": 387,
"external_key": null,
"token": "y10e3vbl3o9a",
"company": "Karli Marie ",
"bill_addr1": "56 Greene Avenue ",
"bill_addr2": null,
"bill_addr3": null,
"bill_city": "New Haven",
"bill_state": "CT ",
"bill_postcode": null,
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"terms_id": 2,
"class_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"admin_id": 3,
"campaign_id": null,
"currency_id": 1,
"language_id": 1,
"brand_id": 1,
"default_paymethod": null,
"default_creditcard_id": null,
"default_ach_id": null,
"tax_ident": "",
"no_taxes": false,
"no_dunning": false,
"write_datetime": "2018-02-23 13:01:53",
"write_ipaddr": "127.0.0.1",
"mod_datetime": "2018-02-23 13:01:53",
"mod_ipaddr": "127.0.0.1",
"terms_name": "Net 30",
"terms_days": 30,
"class_name": "",
"paid": 1996,
"total": 2096,
"balance": 100,
"url_statementlink": "http:\/\/dev.chargeover.com\/r\/statement\/view\/y10e3vbl3o9a",
"url_paymethodlink": "http:\/\/dev.chargeover.com\/r\/paymethod\/i\/y10e3vbl3o9a",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/customer\/view\/38",
"admin_name": "ChargeOver Support",
"admin_email": "support@chargeover.com",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "Karli Marie ",
"ship_block": "",
"bill_block": "56 Greene Avenue \nNew Haven CT\nUnited States",
"superuser_name": "Karli Marie",
"superuser_first_name": "Karli",
"superuser_last_name": "Marie",
"superuser_phone": null,
"superuser_email": "karli@example.com",
"superuser_token": "44yzf79u168x",
"customer_id": 38,
"parent_customer_id": null,
"invoice_delivery": "email",
"dunning_delivery": "email",
"customer_status_id": 1,
"customer_status_name": "Current",
"customer_status_str": "active-current",
"customer_status_state": "a",
"superuser_username": "karli@example.com"
},
"user": {
"user_id": 387,
"external_key": null,
"first_name": "Karli",
"middle_name_glob": null,
"last_name": "Marie",
"name_suffix": null,
"title": null,
"email": "karli@example.com",
"token": "44yzf79u168x",
"phone": null,
"user_type_id": 1,
"write_datetime": "2018-02-23 13:01:53",
"mod_datetime": "2018-02-23 13:01:53",
"name": "Karli Marie",
"display_as": "Karli Marie",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/contact\/view\/387",
"user_type_name": "Billing",
"username": "karli@example.com",
"customer_id": 38
}
},
"security_token": "9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo"
}
A customer status has changed
This is an event that is kicked off when a customers status has changed.
Note these fields:
context_str = customer
context_id = ...
(this will be thecustomer_id
of the customer)event = status
A customer status has changed
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: 9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo
X-Chargeover-Source: example.chargeover.com
{
"context_str": "customer",
"context_id": "38",
"event": "status",
"data": {
"customer": {
"superuser_id": 387,
"external_key": null,
"token": "y10e3vbl3o9a",
"company": "Karli Marie ",
"bill_addr1": "56 Greene Avenue ",
"bill_addr2": null,
"bill_addr3": null,
"bill_city": "New Haven",
"bill_state": "CT ",
"bill_postcode": null,
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"terms_id": 2,
"class_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"admin_id": 3,
"campaign_id": null,
"custtype_id": null,
"currency_id": 1,
"language_id": 1,
"brand_id": 1,
"default_paymethod": null,
"default_creditcard_id": null,
"default_ach_id": null,
"tax_ident": "",
"no_taxes": false,
"no_dunning": false,
"write_datetime": "2018-02-23 13:01:53",
"write_ipaddr": "127.0.0.1",
"mod_datetime": "2018-02-23 13:01:53",
"mod_ipaddr": "127.0.0.1",
"terms_name": "Net 30",
"terms_days": 30,
"class_name": "",
"paid": 1996,
"total": 2096,
"balance": 100,
"url_statementlink": "http:\/\/dev.chargeover.com\/r\/statement\/view\/y10e3vbl3o9a",
"url_paymethodlink": "http:\/\/dev.chargeover.com\/r\/paymethod\/i\/y10e3vbl3o9a",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/customer\/view\/38",
"admin_name": "ChargeOver Support",
"admin_email": "support@chargeover.com",
"currency_symbol": "$",
"currency_iso4217": "USD",
"custtype_name": "",
"display_as": "Karli Marie ",
"ship_block": "",
"bill_block": "56 Greene Avenue \nNew Haven CT\nUnited States",
"superuser_name": "Karli Marie",
"superuser_first_name": "Karli",
"superuser_last_name": "Marie",
"superuser_phone": null,
"superuser_email": "karli@example.com",
"superuser_token": "44yzf79u168x",
"customer_id": 38,
"parent_customer_id": null,
"invoice_delivery": "email",
"dunning_delivery": "email",
"customer_status_id": 1,
"customer_status_name": "Current",
"customer_status_str": "active-current",
"customer_status_state": "a",
"superuser_username": "karli@example.com"
},
"user": {
"user_id": 387,
"external_key": null,
"first_name": "Karli",
"middle_name_glob": null,
"last_name": "Marie",
"name_suffix": null,
"title": null,
"email": "karli@example.com",
"token": "44yzf79u168x",
"phone": null,
"user_type_id": 1,
"write_datetime": "2018-02-23 13:01:53",
"mod_datetime": "2018-02-23 13:01:53",
"name": "Karli Marie",
"display_as": "Karli Marie",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/contact\/view\/387",
"user_type_name": "Billing",
"username": "karli@example.com",
"customer_id": 38
}
},
"security_token": "9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo"
}
Users (Contacts)
A user is created
This event occurs when a new user/contact is created.
Note these fields:
context_str = user
context_id = ...
(this will be theuser_id
of the new user)event = insert
A user is created
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: Ma4hWVUNLx0O5zfmJSjQ3AgwpRdY7qu1
X-Chargeover-Source: example.chargeover.com
{
"context_str": "user",
"context_id": 370,
"event": "insert",
"data": {
"user": {
"user_id": 370,
"external_key": null,
"first_name": "John",
"middle_name_glob": null,
"last_name": "Doe",
"name_suffix": null,
"title": "",
"email": "johndoe@chargeover.com",
"phone": "860-634-1602",
"write_datetime": "2014-12-20 12:29:31",
"mod_datetime": "2014-12-20 12:29:31",
"name": "John Doe",
"display_as": "John Doe",
"username": "johndoe@chargeover.com"
},
"the_users_password": ""
},
"security_token": "Ma4hWVUNLx0O5zfmJSjQ3AgwpRdY7qu1"
}
A user is updated
This event occurs when an existing user/contact is updated.
Note these fields:
context_str = user
context_id = ...
(this will be theuser_id
of the user)event = update
A user is updated
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: 9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo
X-Chargeover-Source: example.chargeover.com
{
"context_str": "user",
"context_id": "355",
"event": "update",
"data": {
"user": {
"user_id": 355,
"external_key": null,
"first_name": "Jane",
"middle_name_glob": null,
"last_name": "Doe",
"name_suffix": null,
"title": "",
"email": "jane@example.com",
"token": "xp40296s7en2",
"phone": "111-111-1111",
"user_type_id": 1,
"write_datetime": "2017-06-13 12:52:40",
"mod_datetime": "2017-06-15 14:13:40",
"name": "Jane Doe",
"display_as": "Jane Doe",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/contact\/view\/355",
"user_type_name": "Billing",
"username": "prt8anm9wqfd",
"customer_id": 3
}
},
"security_token": "9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo"
}
A user is deleted
This event occurs when an existing user/contact is deleted.
Note these fields:
context_str = user
context_id = ...
(this will be theuser_id
of the user)event = delete
A user is deleted
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: 9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo
X-Chargeover-Source: example.chargeover.com
{
"context_str": "user",
"context_id": "349",
"event": "delete",
"data": {
"user": {
"user_id": 349,
"external_key": null,
"first_name": "Test",
"middle_name_glob": "Customer",
"last_name": "2",
"name_suffix": null,
"title": "",
"email": "",
"token": "11l8803esn53",
"phone": "",
"user_type_id": 1,
"write_datetime": "2017-04-06 14:35:18",
"mod_datetime": "2017-04-06 14:35:18",
"name": "Test Customer 2",
"display_as": "Test Customer 2",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/contact\/view\/349",
"user_type_name": "Billing",
"username": "4j9FHYA0GOrZ",
"customer_id": 3
}
},
"security_token": "9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo"
}
Quotes
A quote status has changed
This event occurs when an quote changes status (for example, if the quote is marked as lost or if it is accepted by the customer).
Note these fields:
context_str = quote
context_id = ...
(this will be thequote_id
value of the quote that changed)event = status
Your script should examine these attributes to determine the new status of the quote:
quote.quote_status_str
quote.quote_status_state
quote.quote_status_name
attribute, which provides a human-friendly status value.If a quote has been accepted and turned into an invoice, there will be an
invoices
attribute containing the related invoice.If a quote has been accepted and turned into a subscription, there will be a
packages
attribute containing the related subscription.
A quote status has changed
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: kdZOgKUzDt6bylJH1LrVjMx5m0wYScpA
X-Chargeover-Source: example.chargeover.com
{
"context_str": "quote",
"context_id": "204",
"event": "status",
"data": {
"quote": {
"quote_id": 204,
"terms_id": 2,
"admin_id": 1,
"currency_id": 1,
"token": "0264d61l0504",
"refnumber": "204",
"paycycle": "mon",
"bill_addr1": "72 E Blue Grass Road",
"bill_addr2": "",
"bill_addr3": "",
"bill_city": "Minneapolis",
"bill_state": "MN",
"bill_postcode": "55416",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"memo": "",
"custom_1": null,
"custom_2": null,
"custom_3": null,
"write_datetime": "2019-08-14 15:25:41",
"quote_status_name": "Accepted",
"quote_status_str": "closed-won",
"quote_status_state": "c",
"currency_symbol": "$",
"currency_iso4217": "USD",
"total": 10.95,
"setup": 0,
"terms_name": "Net 30",
"date": "2019-08-14",
"due_date": "2019-09-13",
"bill_block": "72 E Blue Grass Road\r\nMinneapolis, MN 55416\r\nUnited States",
"ship_block": "",
"url_permalink": "http:\/\/dev1.chargeover.com\/r\/quote\/view\/0264d61l0504",
"url_pdflink": "http:\/\/dev1.chargeover.com\/r\/quote\/pdf\/0264d61l0504",
"url_self": "http:\/\/dev1.chargeover.com\/admin\/r\/quote\/view\/204",
"url_acceptlink": "http:\/\/dev1.chargeover.com\/r\/quote\/accept\/0264d61l0504",
"url_rejectlink": "http:\/\/dev1.chargeover.com\/r\/quote\/reject\/0264d61l0504",
"customer_id": 294,
"line_items": [
{
"quote_id": 204,
"item_id": 1,
"tierset_id": 1,
"descrip": "This is a ChargeOver test item.",
"line_rate": 10.95,
"line_quantity": 1,
"is_base": false,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"item_name": "My Test Service Plan",
"item_external_key": null,
"item_token": "d70a9a880d95",
"item_accounting_sku": null,
"tierset": {
"tierset_id": 1,
"currency_id": 1,
"setup": 0,
"base": 10.95,
"minimum": 0,
"percent": 0,
"paycycle": "evy",
"pricemodel": "fla",
"write_datetime": "2019-07-25 12:39:07",
"mod_datetime": "2019-07-25 12:39:07",
"currency_symbol": "$",
"currency_iso4217": "USD",
"setup_formatted": "$ 0.00",
"base_formatted": "$ 10.95",
"minimum_formatted": "$ 0.00",
"percent_formatted": "0 %",
"pricemodel_desc": "Flat Pricing (example: $X dollars every billing cycle)",
"tiers": []
},
"line_total": 10.95,
"line_subtotal": 10.95,
"line_item_id": 376
}
]
},
"customer": {
"superuser_id": 247,
"external_key": null,
"token": "q21f64t8645l",
"company": "John Doe's Company",
"bill_addr1": "72 E Blue Grass Road",
"bill_addr2": null,
"bill_addr3": null,
"bill_city": "Minneapolis",
"bill_state": "MN",
"bill_postcode": "55416",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"terms_id": 2,
"class_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"custom_7": null,
"custom_8": null,
"custom_9": null,
"custom_10": null,
"custom_11": null,
"custom_12": null,
"custom_13": null,
"custom_14": null,
"custom_15": null,
"custom_16": null,
"custom_17": null,
"custom_18": null,
"custom_19": null,
"custom_20": null,
"admin_id": 1,
"campaign_id": null,
"currency_id": 1,
"language_id": 1,
"brand_id": 1,
"default_paymethod": null,
"default_creditcard_id": null,
"default_ach_id": null,
"tax_ident": "",
"no_taxes": false,
"no_dunning": false,
"write_datetime": "2019-08-14 10:38:26",
"write_ipaddr": "10.80.1.1",
"mod_datetime": "2019-08-14 10:38:26",
"mod_ipaddr": "10.80.1.1",
"terms_name": "Net 30",
"terms_days": 30,
"class_name": "",
"paid": 0,
"total": 0,
"balance": 0,
"url_statementlink": "http:\/\/dev1.chargeover.com\/r\/statement\/view\/q21f64t8645l",
"url_paymethodlink": "http:\/\/dev1.chargeover.com\/r\/paymethod\/i\/q21f64t8645l",
"url_self": "http:\/\/dev1.chargeover.com\/admin\/r\/customer\/view\/294",
"admin_name": "ChargeOver Support",
"admin_email": "support@ChargeOver.com",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "John Doe's Company",
"ship_block": "",
"bill_block": "72 E Blue Grass Road\nMinneapolis MN 55416\nUnited States",
"superuser_name": "John Doe",
"superuser_first_name": "John",
"superuser_last_name": "Doe",
"superuser_phone": null,
"superuser_email": "jon@example.com",
"superuser_token": "5650i41z234v",
"customer_id": 294,
"parent_customer_id": null,
"invoice_delivery": "email",
"dunning_delivery": "email",
"customer_status_id": 1,
"customer_status_name": "Current",
"customer_status_str": "active-current",
"customer_status_state": "a",
"superuser_username": "jon@example.com"
},
"invoices": [],
"packages": [
{
"terms_id": 2,
"class_id": null,
"admin_id": 1,
"currency_id": 1,
"brand_id": 1,
"external_key": null,
"token": "w155dyrztju9",
"nickname": "",
"paymethod": "inv",
"paycycle": "mon",
"bill_addr1": "72 E Blue Grass Road",
"bill_addr2": null,
"bill_addr3": null,
"bill_city": "Minneapolis",
"bill_state": "MN",
"bill_postcode": "55416",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"creditcard_id": null,
"ach_id": null,
"tokenized_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"write_datetime": "2019-08-14 15:28:49",
"mod_datetime": "2019-08-14 15:28:49",
"start_datetime": "2019-08-14 15:28:49",
"suspendfrom_datetime": null,
"suspendto_datetime": null,
"cancel_datetime": null,
"holduntil_datetime": null,
"terms_name": "Net 30",
"terms_days": 30,
"currency_symbol": "$",
"currency_iso4217": "USD",
"class_name": "",
"amount_collected": 0,
"amount_invoiced": 0,
"amount_due": 0,
"is_overdue": false,
"days_overdue": 0,
"next_invoice_datetime": "2019-08-15 00:00:01",
"cancel_reason": null,
"paycycle_name": "Monthly",
"paymethod_name": "Invoice",
"url_self": "http:\/\/dev1.chargeover.com\/admin\/r\/package\/view\/45",
"package_id": 45,
"customer_id": 294,
"package_status_id": 2,
"package_status_name": "Current",
"package_status_str": "active-current",
"package_status_state": "a"
}
]
},
"security_token": "kdZOgKUzDt6bylJH1LrVjMx5m0wYScpA"
}
A quote is created
This event occurs when a quote is created.
Note these fields:
context_str = quote
context_id = ...
(this will be thequote_id
of the quote)event = insert
A quote is created
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: kdZOgKUzDt6bylJH1LrVjMx5m0wYScpA
X-Chargeover-Source: example.chargeover.com
{
"context_str": "quote",
"context_id": "204",
"event": "insert",
"data": {
"quote": {
"quote_id": 204,
"terms_id": 2,
"admin_id": 1,
"currency_id": 1,
"token": "0264d61l0504",
"refnumber": "204",
"paycycle": "mon",
"bill_addr1": "72 E Blue Grass Road",
"bill_addr2": null,
"bill_addr3": null,
"bill_city": "Minneapolis",
"bill_state": "MN",
"bill_postcode": "55416",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"memo": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"write_datetime": "2019-08-14 15:25:41",
"quote_status_name": "New",
"quote_status_str": "open-new",
"quote_status_state": "o",
"currency_symbol": "$",
"currency_iso4217": "USD",
"total": 10.95,
"setup": 0,
"terms_name": "Net 30",
"date": "2019-08-14",
"due_date": "2019-09-13",
"bill_block": "72 E Blue Grass Road\r\nMinneapolis, MN 55416\r\nUnited States",
"ship_block": "",
"url_permalink": "http:\/\/dev1.chargeover.com\/r\/quote\/view\/0264d61l0504",
"url_pdflink": "http:\/\/dev1.chargeover.com\/r\/quote\/pdf\/0264d61l0504",
"url_self": "http:\/\/dev1.chargeover.com\/admin\/r\/quote\/view\/204",
"url_acceptlink": "http:\/\/dev1.chargeover.com\/r\/quote\/accept\/0264d61l0504",
"url_rejectlink": "http:\/\/dev1.chargeover.com\/r\/quote\/reject\/0264d61l0504",
"customer_id": 294,
"line_items": [
{
"quote_id": 204,
"item_id": 1,
"tierset_id": 1,
"descrip": "This is a ChargeOver test item.",
"line_rate": 10.95,
"line_quantity": 1,
"is_base": false,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"item_name": "My Test Service Plan",
"item_external_key": null,
"item_token": "d70a9a880d95",
"item_accounting_sku": null,
"tierset": {
"tierset_id": 1,
"currency_id": 1,
"setup": 0,
"base": 10.95,
"minimum": 0,
"percent": 0,
"paycycle": "evy",
"pricemodel": "fla",
"write_datetime": "2019-07-25 12:39:07",
"mod_datetime": "2019-07-25 12:39:07",
"currency_symbol": "$",
"currency_iso4217": "USD",
"setup_formatted": "$ 0.00",
"base_formatted": "$ 10.95",
"minimum_formatted": "$ 0.00",
"percent_formatted": "0 %",
"pricemodel_desc": "Flat Pricing (example: $X dollars every billing cycle)",
"tiers": []
},
"line_total": 10.95,
"line_subtotal": 10.95,
"line_item_id": 376
}
]
},
"customer": {
"superuser_id": 247,
"external_key": null,
"token": "q21f64t8645l",
"company": "John Doe's Company",
"bill_addr1": "72 E Blue Grass Road",
"bill_addr2": null,
"bill_addr3": null,
"bill_city": "Minneapolis",
"bill_state": "MN",
"bill_postcode": "55416",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"terms_id": 2,
"class_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"custom_7": null,
"custom_8": null,
"custom_9": null,
"custom_10": null,
"custom_11": null,
"custom_12": null,
"custom_13": null,
"custom_14": null,
"custom_15": null,
"custom_16": null,
"custom_17": null,
"custom_18": null,
"custom_19": null,
"custom_20": null,
"admin_id": 1,
"campaign_id": null,
"currency_id": 1,
"language_id": 1,
"brand_id": 1,
"default_paymethod": null,
"default_creditcard_id": null,
"default_ach_id": null,
"tax_ident": "",
"no_taxes": false,
"no_dunning": false,
"write_datetime": "2019-08-14 10:38:26",
"write_ipaddr": "10.80.1.1",
"mod_datetime": "2019-08-14 10:38:26",
"mod_ipaddr": "10.80.1.1",
"terms_name": "Net 30",
"terms_days": 30,
"class_name": "",
"paid": 0,
"total": 0,
"balance": 0,
"url_statementlink": "http:\/\/dev1.chargeover.com\/r\/statement\/view\/q21f64t8645l",
"url_paymethodlink": "http:\/\/dev1.chargeover.com\/r\/paymethod\/i\/q21f64t8645l",
"url_self": "http:\/\/dev1.chargeover.com\/admin\/r\/customer\/view\/294",
"admin_name": "ChargeOver Support",
"admin_email": "support@ChargeOver.com",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "John Doe's Company",
"ship_block": "",
"bill_block": "72 E Blue Grass Road\nMinneapolis MN 55416\nUnited States",
"superuser_name": "John Doe",
"superuser_first_name": "John",
"superuser_last_name": "Doe",
"superuser_phone": null,
"superuser_email": "jon@example.com",
"superuser_token": "5650i41z234v",
"customer_id": 294,
"parent_customer_id": null,
"invoice_delivery": "email",
"dunning_delivery": "email",
"customer_status_id": 1,
"customer_status_name": "Current",
"customer_status_str": "active-current",
"customer_status_state": "a",
"superuser_username": "jon@example.com"
},
"invoices": [],
"packages": []
},
"security_token": "kdZOgKUzDt6bylJH1LrVjMx5m0wYScpA"
}
A quote is updated
This event occurs when a quote is updated.
Note that this event will NOT occur when a quote has been lost or accepted/converted into an invoice (because the actual quote itself hasn't changed in these cases).
If you want to know when quote changes status (e.g. from "open" to "closed-accepted") you should listen for the status
webhook on quotes.
Note these fields:
context_str = quote
context_id = ...
(this will be thequote_id
of the quote)event = update
A quote is updated
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: kdZOgKUzDt6bylJH1LrVjMx5m0wYScpA
X-Chargeover-Source: example.chargeover.com
{
"context_str": "quote",
"context_id": "204",
"event": "update",
"data": {
"quote": {
"quote_id": 204,
"terms_id": 2,
"admin_id": 1,
"currency_id": 1,
"token": "0264d61l0504",
"refnumber": "204",
"paycycle": "mon",
"bill_addr1": "72 E Blue Grass Road",
"bill_addr2": "",
"bill_addr3": "",
"bill_city": "Minneapolis",
"bill_state": "MN",
"bill_postcode": "55416",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"memo": "",
"custom_1": null,
"custom_2": null,
"custom_3": null,
"write_datetime": "2019-08-14 15:25:41",
"quote_status_name": "New",
"quote_status_str": "open-new",
"quote_status_state": "o",
"currency_symbol": "$",
"currency_iso4217": "USD",
"total": 10.95,
"setup": 0,
"terms_name": "Net 30",
"date": "2019-08-14",
"due_date": "2019-09-13",
"bill_block": "72 E Blue Grass Road\r\nMinneapolis, MN 55416\r\nUnited States",
"ship_block": "",
"url_permalink": "http:\/\/dev1.chargeover.com\/r\/quote\/view\/0264d61l0504",
"url_pdflink": "http:\/\/dev1.chargeover.com\/r\/quote\/pdf\/0264d61l0504",
"url_self": "http:\/\/dev1.chargeover.com\/admin\/r\/quote\/view\/204",
"url_acceptlink": "http:\/\/dev1.chargeover.com\/r\/quote\/accept\/0264d61l0504",
"url_rejectlink": "http:\/\/dev1.chargeover.com\/r\/quote\/reject\/0264d61l0504",
"customer_id": 294,
"line_items": [
{
"quote_id": 204,
"item_id": 1,
"tierset_id": 1,
"descrip": "This is a ChargeOver test item.",
"line_rate": 10.95,
"line_quantity": 1,
"is_base": false,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"item_name": "My Test Service Plan",
"item_external_key": null,
"item_token": "d70a9a880d95",
"item_accounting_sku": null,
"tierset": {
"tierset_id": 1,
"currency_id": 1,
"setup": 0,
"base": 10.95,
"minimum": 0,
"percent": 0,
"paycycle": "evy",
"pricemodel": "fla",
"write_datetime": "2019-07-25 12:39:07",
"mod_datetime": "2019-07-25 12:39:07",
"currency_symbol": "$",
"currency_iso4217": "USD",
"setup_formatted": "$ 0.00",
"base_formatted": "$ 10.95",
"minimum_formatted": "$ 0.00",
"percent_formatted": "0 %",
"pricemodel_desc": "Flat Pricing (example: $X dollars every billing cycle)",
"tiers": []
},
"line_total": 10.95,
"line_subtotal": 10.95,
"line_item_id": 376
}
]
},
"customer": {
"superuser_id": 247,
"external_key": null,
"token": "q21f64t8645l",
"company": "John Doe's Company",
"bill_addr1": "72 E Blue Grass Road",
"bill_addr2": null,
"bill_addr3": null,
"bill_city": "Minneapolis",
"bill_state": "MN",
"bill_postcode": "55416",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"terms_id": 2,
"class_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"custom_7": null,
"custom_8": null,
"custom_9": null,
"custom_10": null,
"custom_11": null,
"custom_12": null,
"custom_13": null,
"custom_14": null,
"custom_15": null,
"custom_16": null,
"custom_17": null,
"custom_18": null,
"custom_19": null,
"custom_20": null,
"admin_id": 1,
"campaign_id": null,
"currency_id": 1,
"language_id": 1,
"brand_id": 1,
"default_paymethod": null,
"default_creditcard_id": null,
"default_ach_id": null,
"tax_ident": "",
"no_taxes": false,
"no_dunning": false,
"write_datetime": "2019-08-14 10:38:26",
"write_ipaddr": "10.80.1.1",
"mod_datetime": "2019-08-14 10:38:26",
"mod_ipaddr": "10.80.1.1",
"terms_name": "Net 30",
"terms_days": 30,
"class_name": "",
"paid": 0,
"total": 0,
"balance": 0,
"url_statementlink": "http:\/\/dev1.chargeover.com\/r\/statement\/view\/q21f64t8645l",
"url_paymethodlink": "http:\/\/dev1.chargeover.com\/r\/paymethod\/i\/q21f64t8645l",
"url_self": "http:\/\/dev1.chargeover.com\/admin\/r\/customer\/view\/294",
"admin_name": "ChargeOver Support",
"admin_email": "support@ChargeOver.com",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "John Doe's Company",
"ship_block": "",
"bill_block": "72 E Blue Grass Road\nMinneapolis MN 55416\nUnited States",
"superuser_name": "John Doe",
"superuser_first_name": "John",
"superuser_last_name": "Doe",
"superuser_phone": null,
"superuser_email": "jon@example.com",
"superuser_token": "5650i41z234v",
"customer_id": 294,
"parent_customer_id": null,
"invoice_delivery": "email",
"dunning_delivery": "email",
"customer_status_id": 1,
"customer_status_name": "Current",
"customer_status_str": "active-current",
"customer_status_state": "a",
"superuser_username": "jon@example.com"
},
"invoices": [],
"packages": []
},
"security_token": "kdZOgKUzDt6bylJH1LrVjMx5m0wYScpA"
}
The nightly event occurs
This is an event that is kicked off nightly for each quote.
This event only occurs for new and open quotes. Won or lost quotes will not fire this event.
Internally, this event is used for updating caches, consistency checks etc.
Externally, you can choose to hook into this event and use it for whatever you would like.
Note these fields:
context_str = quote
context_id = ...
(this will be thequote_id
of the quote)event = nightly
The nightly event occurs
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: kdZOgKUzDt6bylJH1LrVjMx5m0wYScpA
X-Chargeover-Source: example.chargeover.com
{
"context_str": "quote",
"context_id": "204",
"event": "nightly",
"data": {
"quote": {
"quote_id": 204,
"terms_id": 2,
"admin_id": 1,
"currency_id": 1,
"token": "0264d61l0504",
"refnumber": "204",
"paycycle": "mon",
"bill_addr1": "72 E Blue Grass Road",
"bill_addr2": "",
"bill_addr3": "",
"bill_city": "Minneapolis",
"bill_state": "MN",
"bill_postcode": "55416",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"memo": "",
"custom_1": null,
"custom_2": null,
"custom_3": null,
"write_datetime": "2019-08-14 15:25:41",
"quote_status_name": "New",
"quote_status_str": "open-new",
"quote_status_state": "o",
"currency_symbol": "$",
"currency_iso4217": "USD",
"total": 10.95,
"setup": 0,
"terms_name": "Net 30",
"date": "2019-08-14",
"due_date": "2019-09-13",
"bill_block": "72 E Blue Grass Road\r\nMinneapolis, MN 55416\r\nUnited States",
"ship_block": "",
"url_permalink": "http:\/\/dev1.chargeover.com\/r\/quote\/view\/0264d61l0504",
"url_pdflink": "http:\/\/dev1.chargeover.com\/r\/quote\/pdf\/0264d61l0504",
"url_self": "http:\/\/dev1.chargeover.com\/admin\/r\/quote\/view\/204",
"url_acceptlink": "http:\/\/dev1.chargeover.com\/r\/quote\/accept\/0264d61l0504",
"url_rejectlink": "http:\/\/dev1.chargeover.com\/r\/quote\/reject\/0264d61l0504",
"customer_id": 294,
"line_items": [
{
"quote_id": 204,
"item_id": 1,
"tierset_id": 1,
"descrip": "This is a ChargeOver test item.",
"line_rate": 10.95,
"line_quantity": 1,
"is_base": false,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"item_name": "My Test Service Plan",
"item_external_key": null,
"item_token": "d70a9a880d95",
"item_accounting_sku": null,
"tierset": {
"tierset_id": 1,
"currency_id": 1,
"setup": 0,
"base": 10.95,
"minimum": 0,
"percent": 0,
"paycycle": "evy",
"pricemodel": "fla",
"write_datetime": "2019-07-25 12:39:07",
"mod_datetime": "2019-07-25 12:39:07",
"currency_symbol": "$",
"currency_iso4217": "USD",
"setup_formatted": "$ 0.00",
"base_formatted": "$ 10.95",
"minimum_formatted": "$ 0.00",
"percent_formatted": "0 %",
"pricemodel_desc": "Flat Pricing (example: $X dollars every billing cycle)",
"tiers": []
},
"line_total": 10.95,
"line_subtotal": 10.95,
"line_item_id": 376
}
]
},
"customer": {
"superuser_id": 247,
"external_key": null,
"token": "q21f64t8645l",
"company": "John Doe's Company",
"bill_addr1": "72 E Blue Grass Road",
"bill_addr2": null,
"bill_addr3": null,
"bill_city": "Minneapolis",
"bill_state": "MN",
"bill_postcode": "55416",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"terms_id": 2,
"class_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"custom_7": null,
"custom_8": null,
"custom_9": null,
"custom_10": null,
"custom_11": null,
"custom_12": null,
"custom_13": null,
"custom_14": null,
"custom_15": null,
"custom_16": null,
"custom_17": null,
"custom_18": null,
"custom_19": null,
"custom_20": null,
"admin_id": 1,
"campaign_id": null,
"currency_id": 1,
"language_id": 1,
"brand_id": 1,
"default_paymethod": null,
"default_creditcard_id": null,
"default_ach_id": null,
"tax_ident": "",
"no_taxes": false,
"no_dunning": false,
"write_datetime": "2019-08-14 10:38:26",
"write_ipaddr": "10.80.1.1",
"mod_datetime": "2019-08-14 10:38:26",
"mod_ipaddr": "10.80.1.1",
"terms_name": "Net 30",
"terms_days": 30,
"class_name": "",
"paid": 0,
"total": 0,
"balance": 0,
"url_statementlink": "http:\/\/dev1.chargeover.com\/r\/statement\/view\/q21f64t8645l",
"url_paymethodlink": "http:\/\/dev1.chargeover.com\/r\/paymethod\/i\/q21f64t8645l",
"url_self": "http:\/\/dev1.chargeover.com\/admin\/r\/customer\/view\/294",
"admin_name": "ChargeOver Support",
"admin_email": "support@ChargeOver.com",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "John Doe's Company",
"ship_block": "",
"bill_block": "72 E Blue Grass Road\nMinneapolis MN 55416\nUnited States",
"superuser_name": "John Doe",
"superuser_first_name": "John",
"superuser_last_name": "Doe",
"superuser_phone": null,
"superuser_email": "jon@example.com",
"superuser_token": "5650i41z234v",
"customer_id": 294,
"parent_customer_id": null,
"invoice_delivery": "email",
"dunning_delivery": "email",
"customer_status_id": 1,
"customer_status_name": "Current",
"customer_status_str": "active-current",
"customer_status_state": "a",
"superuser_username": "jon@example.com"
},
"invoices": [],
"packages": []
},
"security_token": "kdZOgKUzDt6bylJH1LrVjMx5m0wYScpA"
}
A quote is accepted
This event occurs when a quote is accepted and generates an invoice/subscription.
Note these fields:
context_str = quote
context_id = ...
(this will be thequote_id
value of the quote that changed)event = won
Your script should look for these values in these fields:
quote.quote_status_str = closed-won
quote.quote_status_state = c
quote.quote_status_name = Accepted
If a quote has been accepted and turned into an invoice, there will be an
invoices
attribute containing the related invoice.If a quote has been accepted and turned into a subscription, there will be a
packages
attribute containing the related subscription.
A quote is accepted
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: kdZOgKUzDt6bylJH1LrVjMx5m0wYScpA
X-Chargeover-Source: example.chargeover.com
{
"context_str": "quote",
"context_id": "204",
"event": "won",
"data": {
"quote": {
"quote_id": 204,
"terms_id": 2,
"admin_id": 1,
"currency_id": 1,
"token": "0264d61l0504",
"refnumber": "204",
"paycycle": "mon",
"bill_addr1": "72 E Blue Grass Road",
"bill_addr2": "",
"bill_addr3": "",
"bill_city": "Minneapolis",
"bill_state": "MN",
"bill_postcode": "55416",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"memo": "",
"custom_1": null,
"custom_2": null,
"custom_3": null,
"write_datetime": "2019-08-14 15:25:41",
"quote_status_name": "Accepted",
"quote_status_str": "closed-won",
"quote_status_state": "c",
"currency_symbol": "$",
"currency_iso4217": "USD",
"total": 10.95,
"setup": 0,
"terms_name": "Net 30",
"date": "2019-08-14",
"due_date": "2019-09-13",
"bill_block": "72 E Blue Grass Road\r\nMinneapolis, MN 55416\r\nUnited States",
"ship_block": "",
"url_permalink": "https:\/\/dev1.chargeover.com\/r\/quote\/view\/0264d61l0504",
"url_pdflink": "https:\/\/dev1.chargeover.com\/r\/quote\/pdf\/0264d61l0504",
"url_self": "https:\/\/dev1.chargeover.com\/admin\/r\/quote\/view\/204",
"url_acceptlink": "https:\/\/dev1.chargeover.com\/r\/quote\/accept\/0264d61l0504",
"url_rejectlink": "https:\/\/dev1.chargeover.com\/r\/quote\/reject\/0264d61l0504",
"won_datetime": "2019-08-14 15:30:14",
"lost_datetime": "",
"customer_id": 294,
"line_items": [
{
"quote_id": 204,
"item_id": 1,
"tierset_id": 1,
"descrip": "This is a ChargeOver test item.",
"line_rate": 10.95,
"line_quantity": 1,
"is_base": false,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"item_name": "My Test Service Plan",
"item_external_key": null,
"item_token": "d70a9a880d95",
"item_accounting_sku": null,
"tierset": {
"tierset_id": 1,
"currency_id": 1,
"setup": 0,
"base": 10.95,
"minimum": 0,
"percent": 0,
"paycycle": "evy",
"pricemodel": "fla",
"write_datetime": "2019-07-25 12:39:07",
"mod_datetime": "2019-07-25 12:39:07",
"currency_symbol": "$",
"currency_iso4217": "USD",
"setup_formatted": "$ 0.00",
"base_formatted": "$ 10.95",
"minimum_formatted": "$ 0.00",
"percent_formatted": "0 %",
"pricemodel_desc": "Flat Pricing (example: $X dollars every billing cycle)",
"tiers": []
},
"line_total": 10.95,
"line_subtotal": 10.95,
"line_item_id": 376
}
]
},
"customer": {
"superuser_id": 247,
"external_key": null,
"token": "q21f64t8645l",
"company": "John Doe's Company",
"bill_addr1": "72 E Blue Grass Road",
"bill_addr2": null,
"bill_addr3": null,
"bill_city": "Minneapolis",
"bill_state": "MN",
"bill_postcode": "55416",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"terms_id": 2,
"class_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"custom_7": null,
"custom_8": null,
"custom_9": null,
"custom_10": null,
"custom_11": null,
"custom_12": null,
"custom_13": null,
"custom_14": null,
"custom_15": null,
"custom_16": null,
"custom_17": null,
"custom_18": null,
"custom_19": null,
"custom_20": null,
"admin_id": 1,
"campaign_id": null,
"currency_id": 1,
"language_id": 1,
"brand_id": 1,
"default_paymethod": null,
"default_creditcard_id": null,
"default_ach_id": null,
"tax_ident": "",
"no_taxes": false,
"no_dunning": false,
"write_datetime": "2019-08-14 10:38:26",
"write_ipaddr": "10.80.1.1",
"mod_datetime": "2019-08-14 10:38:26",
"mod_ipaddr": "10.80.1.1",
"terms_name": "Net 30",
"terms_days": 30,
"class_name": "",
"paid": 0,
"total": 0,
"balance": 0,
"url_statementlink": "https:\/\/dev1.chargeover.com\/r\/statement\/view\/q21f64t8645l",
"url_paymethodlink": "https:\/\/dev1.chargeover.com\/r\/paymethod\/i\/q21f64t8645l",
"url_self": "https:\/\/dev1.chargeover.com\/admin\/r\/customer\/view\/294",
"admin_name": "ChargeOver Support",
"admin_email": "support@ChargeOver.com",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "John Doe's Company",
"ship_block": "",
"bill_block": "72 E Blue Grass Road\nMinneapolis MN 55416\nUnited States",
"superuser_name": "John Doe",
"superuser_first_name": "John",
"superuser_last_name": "Doe",
"superuser_phone": null,
"superuser_email": "jon@example.com",
"superuser_token": "5650i41z234v",
"customer_id": 294,
"parent_customer_id": null,
"invoice_delivery": "email",
"dunning_delivery": "email",
"customer_status_id": 1,
"customer_status_name": "Current",
"customer_status_str": "active-current",
"customer_status_state": "a",
"superuser_username": "jon@example.com"
},
"invoices": [],
"packages": [
{
"terms_id": 2,
"class_id": null,
"admin_id": 1,
"currency_id": 1,
"brand_id": 1,
"external_key": null,
"token": "w155dyrztju9",
"nickname": "",
"paymethod": "inv",
"paycycle": "mon",
"bill_addr1": "72 E Blue Grass Road",
"bill_addr2": null,
"bill_addr3": null,
"bill_city": "Minneapolis",
"bill_state": "MN",
"bill_postcode": "55416",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"creditcard_id": null,
"ach_id": null,
"tokenized_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"write_datetime": "2019-08-14 15:28:49",
"mod_datetime": "2019-08-14 15:28:49",
"start_datetime": "2019-08-14 15:28:49",
"suspendfrom_datetime": null,
"suspendto_datetime": null,
"cancel_datetime": null,
"holduntil_datetime": null,
"terms_name": "Net 30",
"terms_days": 30,
"currency_symbol": "$",
"currency_iso4217": "USD",
"class_name": "",
"amount_collected": 0,
"amount_invoiced": 0,
"amount_due": 0,
"is_overdue": false,
"days_overdue": 0,
"next_invoice_datetime": "2019-08-15 00:00:01",
"cancel_reason": null,
"paycycle_name": "Monthly",
"paymethod_name": "Invoice",
"url_self": "https:\/\/dev1.chargeover.com\/admin\/r\/package\/view\/45",
"package_id": 45,
"customer_id": 294,
"package_status_id": 2,
"package_status_name": "Current",
"package_status_str": "active-current",
"package_status_state": "a"
}
]
},
"security_token": "kdZOgKUzDt6bylJH1LrVjMx5m0wYScpA"
}
A quote is rejected
This event occurs when a quote is rejected.
Note these fields:
context_str = quote
context_id = ...
(this will be thequote_id
value of the quote that changed)event = lost
Your script should look for these values in these fields:
quote.quote_status_str = closed-lost
quote.quote_status_state = c
quote.quote_status_name = Rejected
A quote is rejected
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: kdZOgKUzDt6bylJH1LrVjMx5m0wYScpA
X-Chargeover-Source: example.chargeover.com
{
"context_str": "quote",
"context_id": "204",
"event": "lost",
"data": {
"quote": {
"quote_id": 204,
"terms_id": 2,
"admin_id": 1,
"currency_id": 1,
"token": "0264d61l0504",
"refnumber": "204",
"paycycle": "mon",
"bill_addr1": "72 E Blue Grass Road",
"bill_addr2": "",
"bill_addr3": "",
"bill_city": "Minneapolis",
"bill_state": "MN",
"bill_postcode": "55416",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"memo": "",
"custom_1": null,
"custom_2": null,
"custom_3": null,
"write_datetime": "2019-08-14 15:25:41",
"quote_status_name": "Rejected",
"quote_status_str": "closed-lost",
"quote_status_state": "c",
"currency_symbol": "$",
"currency_iso4217": "USD",
"total": 10.95,
"setup": 0,
"terms_name": "Net 30",
"date": "2019-08-14",
"due_date": "2019-09-13",
"bill_block": "72 E Blue Grass Road\r\nMinneapolis, MN 55416\r\nUnited States",
"ship_block": "",
"url_permalink": "https:\/\/dev1.chargeover.com\/r\/quote\/view\/0264d61l0504",
"url_pdflink": "https:\/\/dev1.chargeover.com\/r\/quote\/pdf\/0264d61l0504",
"url_self": "https:\/\/dev1.chargeover.com\/admin\/r\/quote\/view\/204",
"url_acceptlink": "https:\/\/dev1.chargeover.com\/r\/quote\/accept\/0264d61l0504",
"url_rejectlink": "https:\/\/dev1.chargeover.com\/r\/quote\/reject\/0264d61l0504",
"won_datetime": "",
"lost_datetime": "2019-08-14 15:30:14",
"customer_id": 294,
"line_items": [
{
"quote_id": 204,
"item_id": 1,
"tierset_id": 1,
"descrip": "This is a ChargeOver test item.",
"line_rate": 10.95,
"line_quantity": 1,
"is_base": false,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"item_name": "My Test Service Plan",
"item_external_key": null,
"item_token": "d70a9a880d95",
"item_accounting_sku": null,
"tierset": {
"tierset_id": 1,
"currency_id": 1,
"setup": 0,
"base": 10.95,
"minimum": 0,
"percent": 0,
"paycycle": "evy",
"pricemodel": "fla",
"write_datetime": "2019-07-25 12:39:07",
"mod_datetime": "2019-07-25 12:39:07",
"currency_symbol": "$",
"currency_iso4217": "USD",
"setup_formatted": "$ 0.00",
"base_formatted": "$ 10.95",
"minimum_formatted": "$ 0.00",
"percent_formatted": "0 %",
"pricemodel_desc": "Flat Pricing (example: $X dollars every billing cycle)",
"tiers": []
},
"line_total": 10.95,
"line_subtotal": 10.95,
"line_item_id": 376
}
]
},
"customer": {
"superuser_id": 247,
"external_key": null,
"token": "q21f64t8645l",
"company": "John Doe's Company",
"bill_addr1": "72 E Blue Grass Road",
"bill_addr2": null,
"bill_addr3": null,
"bill_city": "Minneapolis",
"bill_state": "MN",
"bill_postcode": "55416",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"terms_id": 2,
"class_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"custom_7": null,
"custom_8": null,
"custom_9": null,
"custom_10": null,
"custom_11": null,
"custom_12": null,
"custom_13": null,
"custom_14": null,
"custom_15": null,
"custom_16": null,
"custom_17": null,
"custom_18": null,
"custom_19": null,
"custom_20": null,
"admin_id": 1,
"campaign_id": null,
"currency_id": 1,
"language_id": 1,
"brand_id": 1,
"default_paymethod": null,
"default_creditcard_id": null,
"default_ach_id": null,
"tax_ident": "",
"no_taxes": false,
"no_dunning": false,
"write_datetime": "2019-08-14 10:38:26",
"write_ipaddr": "10.80.1.1",
"mod_datetime": "2019-08-14 10:38:26",
"mod_ipaddr": "10.80.1.1",
"terms_name": "Net 30",
"terms_days": 30,
"class_name": "",
"paid": 0,
"total": 0,
"balance": 0,
"url_statementlink": "https:\/\/dev1.chargeover.com\/r\/statement\/view\/q21f64t8645l",
"url_paymethodlink": "https:\/\/dev1.chargeover.com\/r\/paymethod\/i\/q21f64t8645l",
"url_self": "https:\/\/dev1.chargeover.com\/admin\/r\/customer\/view\/294",
"admin_name": "ChargeOver Support",
"admin_email": "support@ChargeOver.com",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "John Doe's Company",
"ship_block": "",
"bill_block": "72 E Blue Grass Road\nMinneapolis MN 55416\nUnited States",
"superuser_name": "John Doe",
"superuser_first_name": "John",
"superuser_last_name": "Doe",
"superuser_phone": null,
"superuser_email": "jon@example.com",
"superuser_token": "5650i41z234v",
"customer_id": 294,
"parent_customer_id": null,
"invoice_delivery": "email",
"dunning_delivery": "email",
"customer_status_id": 1,
"customer_status_name": "Current",
"customer_status_str": "active-current",
"customer_status_state": "a",
"superuser_username": "jon@example.com"
},
"invoices": [],
"packages": []
},
"security_token": "kdZOgKUzDt6bylJH1LrVjMx5m0wYScpA"
}
Invoices
An invoice status has changed
This event occurs when an invoice changes status (for example, when an invoice becomes overdue).
Note these fields:
context_str = invoice
context_id = ...
(this will be theinvoice_id
value of the invoice that changed)event = status
Your script should examine these attributes to determine the new status of the invoice:
invoice.invoice_status_str
invoice.invoice_status_state
invoice.invoice_status_name
attribute, which provides a human-friendly status value.
An invoice status has changed
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: xhtfLO9TumgUs6QRpzr8cKMFPHqAnad2
X-Chargeover-Source: example.chargeover.com
{
"context_str": "invoice",
"context_id": "10006",
"event": "status",
"data": {
"invoice": {
"invoice_id": 10006,
"currency_id": 1,
"terms_id": 2,
"token": "3qxb5ae82onp",
"refnumber": "10006",
"bill_addr1": null,
"bill_addr2": null,
"bill_addr3": null,
"bill_city": null,
"bill_state": null,
"bill_postcode": null,
"bill_country": null,
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"write_datetime": "2014-12-09 12:20:02",
"void_datetime": null,
"currency_symbol": "$",
"currency_iso4217": "USD",
"invoice_status_name": "Overdue",
"invoice_status_str": "open-overdue",
"invoice_status_state": "o",
"total": 131.4,
"credits": 0,
"payments": 0,
"sum_base": 131.4,
"sum_usage": 0,
"sum_onetime": 0,
"is_paid": false,
"balance": 131.4,
"is_void": false,
"due_date": "2014-01-07",
"terms_name": "Net 30",
"days_overdue": 336,
"is_overdue": true,
"date": "2013-12-08",
"delay_datetime": null,
"bill_block": "",
"ship_block": "",
"url_permalink": "http:\/\/dev.chargeover.com\/signup\/r\/invoice\/view\/3qxb5ae82onp",
"url_paylink": "http:\/\/dev.chargeover.com\/signup\/r\/trans\/pay\/3qxb5ae82onp",
"url_pdflink": "http:\/\/dev.chargeover.com\/signup\/r\/invoice\/pdf\/3qxb5ae82onp",
"package_id": 556,
"customer_id": 1
},
"customer": {
"superuser_id": 348,
"external_key": null,
"token": "b93jhu7p1y56",
"company": "Test Customer",
"bill_addr1": null,
"bill_addr2": null,
"bill_addr3": null,
"bill_city": null,
"bill_state": null,
"bill_postcode": null,
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"currency_id": 1,
"write_datetime": "2014-12-02 14:02:23",
"write_ipaddr": "172.16.16.102",
"mod_datetime": "2014-12-02 14:02:23",
"mod_ipaddr": "172.16.16.102",
"paid": 28.85,
"total": 229.95,
"balance": 201.1,
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "Test Customer",
"ship_block": "",
"bill_block": "United States",
"superuser_name": "Test Customer",
"superuser_first_name": "Test",
"superuser_last_name": "Customer",
"superuser_phone": "",
"superuser_email": "keith@chargeover.com",
"customer_id": 1
},
"user": {
"user_id": 348,
"external_key": null,
"first_name": "Test",
"middle_name_glob": null,
"last_name": "Customer",
"name_suffix": null,
"title": "",
"email": "keith@chargeover.com",
"phone": "",
"write_datetime": "2014-12-02 14:02:23",
"mod_datetime": "2014-12-02 14:25:13",
"name": "Test Customer",
"display_as": "Test Customer",
"username": "keith@chargeover.com"
}
},
"security_token": "xhtfLO9TumgUs6QRpzr8cKMFPHqAnad2"
}
An invoice is created
This event occurs when an invoice is created (regardless of whether it is a one-off, manually created invoice, or created via a recurring package).
Note these fields:
context_str = invoice
context_id = ...
(this will be theinvoice_id
of the invoice)event = insert
An invoice is created
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: SFkJ3fy02n17YxNXm96tCGUKVTOAIBsH
X-Chargeover-Source: example.chargeover.com
{
"context_str": "invoice",
"context_id": "10815",
"event": "insert",
"data": {
"invoice": {
"invoice_id": 10815,
"currency_id": 1,
"brand_id": 1,
"terms_id": 3,
"admin_id": null,
"external_key": null,
"token": "j945432hl069",
"refnumber": "10815",
"cycle_pre_from_date": "2018-10-10",
"cycle_pre_to_date": "2018-10-10",
"cycle_this_date": "2018-10-10",
"cycle_post_from_date": "2018-10-09",
"cycle_post_to_date": "2018-10-09",
"bill_addr1": "610 Scimitar Bay",
"bill_addr2": null,
"bill_addr3": null,
"bill_city": "Dover",
"bill_state": "DE",
"bill_postcode": null,
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"memo": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"write_datetime": "2018-10-10 07:21:24",
"void_datetime": null,
"currency_symbol": "$",
"currency_iso4217": "USD",
"admin_name": "",
"invoice_status_name": "Unpaid",
"invoice_status_str": "open-unpaid",
"invoice_status_state": "o",
"subtotal": 500,
"total": 500,
"taxes": 0,
"credits": 0,
"payments": 0,
"writeoffs": 0,
"declines": 0,
"applied": 0,
"sum_base": 500,
"sum_usage": 0,
"sum_onetime": 0,
"is_paid": false,
"paid_date": null,
"balance": 500,
"is_void": false,
"due_date": "2018-10-10",
"terms_name": "Due on Receipt",
"terms_days": 0,
"days_overdue": 0,
"is_overdue": false,
"date": "2018-10-10",
"bill_block": "610 Scimitar Bay\r\nDover, DE \r\nUnited States",
"ship_block": "",
"url_permalink": "https:\/\/dev.chargeover.com\/r\/invoice\/view\/j945432hl069",
"url_paylink": "https:\/\/dev.chargeover.com\/r\/trans\/pay\/j945432hl069",
"url_pdflink": "https:\/\/dev.chargeover.com\/r\/invoice\/pdf\/j945432hl069",
"url_self": "https:\/\/dev.chargeover.com\/admin\/r\/invoice\/view\/10815",
"line_items": [
{
"invoice_id": 10815,
"item_id": 18,
"tierset_id": 50,
"admin_id": null,
"descrip": null,
"line_rate": 5,
"line_quantity": 100,
"line_prorate": 1,
"line_usage": 0,
"usage_from": 0,
"usage_to": 0,
"tax_id": null,
"tax_group_id": null,
"tax_taxable": 500,
"tax_taxed": 0,
"tax_total": 0,
"is_base": true,
"is_free": false,
"is_setup": false,
"is_usage": false,
"is_recurring": true,
"is_latefee": false,
"is_taxed": false,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"item_name": "Daily Service",
"item_external_key": null,
"item_token": "39r76kcav37n",
"item_accounting_sku": null,
"item_units": "",
"tax_name": null,
"tax_group_name": null,
"line_subtotal": 500,
"line_total": 500,
"line_item_id": 3958,
"package_line_id": 697
}
],
"package_id": 634,
"customer_id": 88
},
"customer": {
"superuser_id": 445,
"external_key": null,
"token": "pc287567l06t",
"company": "Alberta LeClair",
"bill_addr1": "610 Scimitar Bay",
"bill_addr2": "",
"bill_addr3": "",
"bill_city": "Dover",
"bill_state": "DE",
"bill_postcode": "",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"terms_id": 3,
"class_id": 0,
"custom_1": "",
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"custom_7": null,
"custom_8": null,
"custom_9": null,
"custom_10": null,
"admin_id": null,
"campaign_id": null,
"currency_id": 1,
"language_id": 1,
"brand_id": 1,
"default_paymethod": null,
"default_creditcard_id": null,
"default_ach_id": null,
"tax_ident": "",
"no_taxes": false,
"no_dunning": false,
"write_datetime": "2017-08-15 14:18:07",
"write_ipaddr": "130.215.221.76",
"mod_datetime": "2018-01-15 12:28:20",
"mod_ipaddr": "192.168.21.10",
"terms_name": "Due on Receipt",
"terms_days": 0,
"class_name": "",
"paid": 2017.31,
"total": 94514.11,
"balance": 92496.8,
"url_statementlink": "https:\/\/dev.chargeover.com\/r\/statement\/view\/pc287567l06t",
"url_paymethodlink": "https:\/\/dev.chargeover.com\/r\/paymethod\/i\/pc287567l06t",
"url_self": "https:\/\/dev.chargeover.com\/admin\/r\/customer\/view\/88",
"admin_name": "",
"admin_email": "",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "Alberta LeClair",
"ship_block": "",
"bill_block": "610 Scimitar Bay\nDover DE\nUnited States",
"superuser_name": "Alberta LeClair",
"superuser_first_name": "Alberta",
"superuser_last_name": "LeClair",
"superuser_phone": "403-830-3056",
"superuser_email": "bert@example.com",
"superuser_token": "z655bp84in0l",
"customer_id": 88,
"parent_customer_id": null,
"invoice_delivery": "print",
"dunning_delivery": "print",
"customer_status_id": 2,
"customer_status_name": "Overdue",
"customer_status_str": "active-overdue",
"customer_status_state": "a",
"superuser_username": "wtxq69vug4e2"
},
"user": {
"user_id": 445,
"external_key": null,
"first_name": "Alberta",
"middle_name_glob": null,
"last_name": "LeClair",
"name_suffix": null,
"title": "",
"email": "bert@example.com",
"token": "z655bp84in0l",
"phone": "403-830-3056",
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"user_type_id": 1,
"write_datetime": "2017-08-15 14:18:07",
"mod_datetime": "2018-01-15 12:29:01",
"name": "Alberta LeClair",
"display_as": "Alberta LeClair",
"url_self": "https:\/\/dev.chargeover.com\/admin\/r\/contact\/view\/445",
"user_type_name": "Billing",
"username": "wtxq69vug4e2",
"customer_id": 88
}
},
"security_token": "SFkJ3fy02n17YxNXm96tCGUKVTOAIBsH"
}
An invoice is updated
This event occurs when an invoice is updated.
Note that this event will NOT occur when an invoice is marked paid (because the actual invoice hasn't changed in that case -- a payment has just been applied to the existing, unchanged invoice).
If you want to know when an invoice changes status (e.g. from "unpaid" to "paid") you should listen for the status
webhook on invoices.
Note these fields:
context_str = invoice
context_id = ...
(this will be theinvoice_id
of the invoice)event = update
An invoice is updated
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: 9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo
X-Chargeover-Source: example.chargeover.com
{
"context_str": "invoice",
"context_id": "5013",
"event": "update",
"data": {
"invoice": {
"invoice_id": 5013,
"currency_id": 1,
"terms_id": 1,
"admin_id": 3,
"external_key": null,
"token": "k144z45u46dh",
"refnumber": "5013",
"cycle_pre_from_date": "2017-06-15",
"cycle_pre_to_date": "2017-07-14",
"cycle_this_date": "2017-06-15",
"cycle_post_from_date": "2017-05-15",
"cycle_post_to_date": "2017-06-14",
"bill_addr1": "34 Doe Street",
"bill_addr2": "",
"bill_addr3": "",
"bill_city": "City",
"bill_state": "New State",
"bill_postcode": "",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"memo": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"write_datetime": "2017-06-15 12:41:26",
"void_datetime": null,
"currency_symbol": "$",
"currency_iso4217": "USD",
"admin_name": "Karli Palmer",
"invoice_status_name": "Unpaid",
"invoice_status_str": "open-unpaid",
"invoice_status_state": "o",
"subtotal": 10.95,
"total": 10.95,
"taxes": 0,
"credits": 0,
"payments": 0,
"writeoffs": 0,
"declines": 0,
"applied": 0,
"sum_base": 10.95,
"sum_usage": 0,
"sum_onetime": 0,
"is_paid": false,
"paid_date": null,
"balance": 10.95,
"is_void": false,
"due_date": "2017-06-30",
"terms_name": "Net 15",
"terms_days": 15,
"days_overdue": 0,
"is_overdue": false,
"date": "2017-06-15",
"bill_block": "34 Doe Street\r\nCity, New State \r\nUnited States",
"ship_block": "",
"url_permalink": "http:\/\/dev.chargeover.com\/r\/invoice\/view\/k144z45u46dh",
"url_paylink": "http:\/\/dev.chargeover.com\/r\/trans\/pay\/k144z45u46dh",
"url_pdflink": "http:\/\/dev.chargeover.com\/r\/invoice\/pdf\/k144z45u46dh",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/invoice\/view\/5013",
"line_items": [
{
"invoice_id": 5013,
"item_id": 1,
"tierset_id": 1,
"admin_id": null,
"descrip": "This is a ChargeOver test item.",
"line_rate": 10.95,
"line_quantity": 1,
"line_prorate": 1,
"line_usage": 0,
"usage_from": 0,
"usage_to": 0,
"tax_id": null,
"tax_group_id": null,
"tax_taxable": 0,
"tax_taxed": 0,
"tax_total": 0,
"is_base": true,
"is_free": false,
"is_setup": false,
"is_usage": false,
"is_recurring": true,
"is_latefee": "0",
"is_taxed": "0",
"custom_1": null,
"custom_2": null,
"custom_3": null,
"item_name": "My Test Service Plan",
"item_external_key": null,
"item_token": "3405ed3c8ef2",
"item_accounting_sku": null,
"item_units": "",
"tax_name": null,
"tax_group_name": null,
"line_subtotal": 10.95,
"line_total": 10.95,
"line_item_id": 16,
"package_line_id": 9
}
],
"package_id": 9,
"customer_id": 8
},
"customer": {
"superuser_id": 354,
"external_key": null,
"token": "2ygprd9569t4",
"company": "John Doe's Company, LLC",
"bill_addr1": "34 Doe Street",
"bill_addr2": "",
"bill_addr3": "",
"bill_city": "City",
"bill_state": "New State",
"bill_postcode": "",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"terms_id": 3,
"class_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"admin_id": 3,
"campaign_id": null,
"currency_id": 1,
"language_id": 1,
"brand_id": 1,
"no_taxes": false,
"no_dunning": false,
"write_datetime": "2017-06-08 15:39:59",
"write_ipaddr": "127.0.0.1",
"mod_datetime": "2017-06-13 12:40:51",
"mod_ipaddr": "127.0.0.1",
"terms_name": "Due on Receipt",
"terms_days": 0,
"paid": 10.95,
"total": 82.85,
"balance": 71.9,
"url_paymethodlink": "http:\/\/dev.chargeover.com\/r\/paymethod\/i\/2ygprd9569t4",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/customer\/view\/8",
"admin_name": "Karli Palmer",
"admin_email": "karli@chargeover.com",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "John Doe's Company, LLC",
"ship_block": "",
"bill_block": "34 Doe Street\nCity New State\nUnited States",
"superuser_name": "John Doe",
"superuser_first_name": "John",
"superuser_last_name": "Doe",
"superuser_phone": "",
"superuser_email": "john@example.com",
"superuser_token": "4e7t9r5a03fv",
"customer_id": 8,
"invoice_delivery": "email",
"dunning_delivery": "email",
"customer_status_id": 1,
"customer_status_name": "Current",
"customer_status_str": "active-current",
"customer_status_state": "a",
"superuser_username": "john@example.com"
},
"user": {
"user_id": 354,
"external_key": null,
"first_name": "John",
"middle_name_glob": null,
"last_name": "Doe",
"name_suffix": null,
"title": "",
"email": "john@example.com",
"token": "4e7t9r5a03fv",
"phone": "",
"user_type_id": 1,
"write_datetime": "2017-06-08 15:39:59",
"mod_datetime": "2017-06-08 15:39:59",
"name": "John Doe",
"display_as": "John Doe",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/contact\/view\/354",
"user_type_name": "Billing",
"username": "john@example.com",
"customer_id": 8
}
},
"security_token": "9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo"
}
An invoice is voided
This event occurs when an invoice is marked void.
Note these fields:
context_str = invoice
context_id = ...
(this will be theinvoice_id
of the invoice)event = void
An invoice is voided
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: Ma4hWVUNLx0O5zfmJSjQ3AgwpRdY7qu2
X-Chargeover-Source: example.chargeover.com
{
"context_str": "invoice",
"context_id": "5012",
"event": "void",
"data": {
"invoice": {
"invoice_id": 5012,
"currency_id": 1,
"terms_id": 1,
"admin_id": 3,
"external_key": null,
"token": "6055c2ym94v3",
"refnumber": "5012",
"cycle_pre_from_date": null,
"cycle_pre_to_date": null,
"cycle_this_date": null,
"cycle_post_from_date": null,
"cycle_post_to_date": null,
"bill_addr1": "45 Somewhere Avenue",
"bill_addr2": null,
"bill_addr3": null,
"bill_city": "City",
"bill_state": "State",
"bill_postcode": null,
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"memo": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"write_datetime": "2017-06-15 09:44:52",
"void_datetime": "2017-06-15 09:45:43",
"currency_symbol": "$",
"currency_iso4217": "USD",
"admin_name": "Karli Palmer",
"invoice_status_name": "Void",
"invoice_status_str": "closed-void",
"invoice_status_state": "c",
"subtotal": 10.95,
"total": 10.95,
"taxes": 0,
"credits": 0,
"payments": 0,
"writeoffs": 0,
"declines": 0,
"applied": 0,
"sum_base": 0,
"sum_usage": 0,
"sum_onetime": 10.95,
"is_paid": true,
"paid_date": null,
"balance": 0,
"is_void": true,
"due_date": "2017-06-30",
"terms_name": "Net 15",
"terms_days": 15,
"days_overdue": 0,
"is_overdue": false,
"date": "2017-06-15",
The nightly event occurs
This is an event that is kicked off nightly for each invoice.
This event only occurs for unpaid/overdue invoices. Voided or paid invoices will not fire this event.
Internally, this event is used for updating caches, consistency checks, scheduled events for invoices, etc.
Externally, you can choose to hook into this event and use it for whatever you would like.
Note these fields:
context_str = invoice
context_id = ...
(this will be theinvoice_id
of the invoice)event = nightly
The nightly event occurs
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: 9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo
X-Chargeover-Source: example.chargeover.com
{
"context_str": "invoice",
"context_id": "5098",
"event": "nightly",
"data": {
"invoice": {
"invoice_id": 5098,
"currency_id": 1,
"brand_id": 1,
"terms_id": 2,
"admin_id": null,
"external_key": null,
"token": "3yfeo482g362",
"refnumber": "5098",
"cycle_pre_from_date": null,
"cycle_pre_to_date": null,
"cycle_this_date": null,
"cycle_post_from_date": null,
"cycle_post_to_date": null,
"bill_addr1": "56 Greene Avenue ",
"bill_addr2": null,
"bill_addr3": null,
"bill_city": "New Haven",
"bill_state": "CT ",
"bill_postcode": null,
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"memo": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"write_datetime": "2018-03-07 10:51:57",
"void_datetime": null,
"currency_symbol": "$",
"currency_iso4217": "USD",
"admin_name": "",
"invoice_status_name": "Unpaid",
"invoice_status_str": "open-unpaid",
"invoice_status_state": "o",
"subtotal": 100,
"total": 100,
"taxes": 0,
"credits": 0,
"payments": 0,
"writeoffs": 0,
"declines": 0,
"applied": 0,
"sum_base": 0,
"sum_usage": 0,
"sum_onetime": 100,
"is_paid": false,
"paid_date": null,
"balance": 100,
"is_void": false,
"due_date": "2018-04-06",
"terms_name": "Net 30",
"terms_days": 30,
"days_overdue": 0,
"is_overdue": false,
"date": "2018-03-07",
"bill_block": "56 Greene Avenue \r\nNew Haven, CT \r\nUnited States",
"ship_block": "",
"url_permalink": "http:\/\/dev.chargeover.com\/r\/invoice\/view\/3yfeo482g362",
"url_paylink": "http:\/\/dev.chargeover.com\/r\/trans\/pay\/3yfeo482g362",
"url_pdflink": "http:\/\/dev.chargeover.com\/r\/invoice\/pdf\/3yfeo482g362",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/invoice\/view\/5098",
"line_items": [
{
"invoice_id": 5098,
"item_id": 259,
"tierset_id": null,
"admin_id": null,
"descrip": "Subscription setup fee",
"line_rate": 100,
"line_quantity": 1,
"line_prorate": 1,
"line_usage": 0,
"usage_from": 0,
"usage_to": 0,
"tax_id": null,
"tax_group_id": null,
"tax_taxable": 100,
"tax_taxed": 0,
"tax_total": 0,
"is_base": false,
"is_free": false,
"is_setup": false,
"is_usage": false,
"is_recurring": false,
"is_latefee": false,
"is_taxed": false,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"item_name": "Monthly Service Setup Fee ",
"item_external_key": null,
"item_token": "wynuc6g849b8",
"item_accounting_sku": null,
"item_units": null,
"tax_name": null,
"tax_group_name": null,
"line_subtotal": 100,
"line_total": 100,
"line_item_id": 158,
"package_line_id": null
}
],
"package_id": null,
"customer_id": 38
},
"customer": {
"superuser_id": 387,
"external_key": null,
"token": "y10e3vbl3o9a",
"company": "Karli Marie ",
"bill_addr1": "56 Greene Avenue ",
"bill_addr2": null,
"bill_addr3": null,
"bill_city": "New Haven",
"bill_state": "CT ",
"bill_postcode": null,
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"terms_id": 2,
"class_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"admin_id": 3,
"campaign_id": null,
"currency_id": 1,
"language_id": 1,
"brand_id": 1,
"default_paymethod": null,
"default_creditcard_id": null,
"default_ach_id": null,
"tax_ident": "",
"no_taxes": false,
"no_dunning": false,
"write_datetime": "2018-02-23 13:01:53",
"write_ipaddr": "127.0.0.1",
"mod_datetime": "2018-02-23 13:01:53",
"mod_ipaddr": "127.0.0.1",
"terms_name": "Net 30",
"terms_days": 30,
"class_name": "",
"paid": 1996,
"total": 2096,
"balance": 100,
"url_statementlink": "http:\/\/dev.chargeover.com\/r\/statement\/view\/y10e3vbl3o9a",
"url_paymethodlink": "http:\/\/dev.chargeover.com\/r\/paymethod\/i\/y10e3vbl3o9a",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/customer\/view\/38",
"admin_name": "ChargeOver Support",
"admin_email": "support@chargeover.com",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "Karli Marie ",
"ship_block": "",
"bill_block": "56 Greene Avenue \nNew Haven CT\nUnited States",
"superuser_name": "Karli Marie",
"superuser_first_name": "Karli",
"superuser_last_name": "Marie",
"superuser_phone": null,
"superuser_email": "karli@example.com",
"superuser_token": "44yzf79u168x",
"customer_id": 38,
"parent_customer_id": null,
"invoice_delivery": "email",
"dunning_delivery": "email",
"customer_status_id": 1,
"customer_status_name": "Current",
"customer_status_str": "active-current",
"customer_status_state": "a",
"superuser_username": "karli@example.com"
},
"user": {
"user_id": 387,
"external_key": null,
"first_name": "Karli",
"middle_name_glob": null,
"last_name": "Marie",
"name_suffix": null,
"title": null,
"email": "karli@example.com",
"token": "44yzf79u168x",
"phone": null,
"user_type_id": 1,
"write_datetime": "2018-02-23 13:01:53",
"mod_datetime": "2018-02-23 13:01:53",
"name": "Karli Marie",
"display_as": "Karli Marie",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/contact\/view\/387",
"user_type_name": "Billing",
"username": "karli@example.com",
"customer_id": 38
}
},
"security_token": "9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo"
}
Subscriptions (Packages)
A subscription status has changed
This event occurs when a package changes status (for example, when a package is cancelled it goes from an "active" status to a "cancelled" status).
Note these fields:
context_str = package
context_id = ...
(this will be thepackage_id
value of the package that changed)event = status
Your script should examine these attributes to determine the new status of the package:
package.package_status_str
package.package_status_state
package.package_status_name
attribute, which provides a human-friendly status value.
A subscription status has changed
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: xhtfLO9TumgUs6QRpzr8cKMFPHqAnad2
X-Chargeover-Source: example.chargeover.com
{
"context_str": "package",
"context_id": "556",
"event": "status",
"data": {
"package": {
"terms_id": 2,
"currency_id": 1,
"external_key": null,
"token": "ozevrx738b02",
"nickname": "",
"paymethod": "inv",
"paycycle": "yrl",
"bill_addr1": null,
"bill_addr2": null,
"bill_addr3": null,
"bill_city": null,
"bill_state": null,
"bill_postcode": null,
"bill_country": null,
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"creditcard_id": null,
"ach_id": null,
"tokenized_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"write_datetime": "2014-12-09 12:19:52",
"mod_datetime": "2014-12-09 12:19:52",
"suspendfrom_datetime": null,
"suspendto_datetime": null,
"cancel_datetime": null,
"holduntil_datetime": null,
"currency_symbol": "$",
"currency_iso4217": "USD",
"amount_collected": 0,
"amount_invoiced": 131.4,
"amount_due": 131.4,
"next_invoice_datetime": "2014-12-10 14:15:39",
"package_id": 556,
"customer_id": 1,
"package_status_id": 3,
"package_status_name": "Over Due",
"package_status_str": "active-overdue",
"package_status_state": "a",
"line_items": [
{
"item_id": 1,
"tierset_id": 1,
"external_key": null,
"nickname": "",
"descrip": "This is a ChargeOver test item.",
"custom_1": null,
"custom_2": null,
"custom_3": null,
"subscribe_datetime": "2014-12-09 12:19:52",
"cancel_datetime": null,
"expire_datetime": null,
"expire_recurs": null,
"license": "",
"item_name": "My Test Service Plan",
"item_external_key": null,
"item_accounting_sku": null,
"item_units": "",
"line_item_id": 558
}
]
},
"customer": {
"superuser_id": 348,
"external_key": null,
"token": "b93jhu7p1y56",
"company": "Test Customer",
"bill_addr1": null,
"bill_addr2": null,
"bill_addr3": null,
"bill_city": null,
"bill_state": null,
"bill_postcode": null,
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"currency_id": 1,
"write_datetime": "2014-12-02 14:02:23",
"write_ipaddr": "172.16.16.102",
"mod_datetime": "2014-12-02 14:02:23",
"mod_ipaddr": "172.16.16.102",
"paid": 28.85,
"total": 229.95,
"balance": 201.1,
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "Test Customer",
"ship_block": "",
"bill_block": "United States",
"superuser_name": "Test Customer",
"superuser_first_name": "Test",
"superuser_last_name": "Customer",
"superuser_phone": "",
"superuser_email": "keith@chargeover.com",
"customer_id": 1
},
"user": {
"user_id": 348,
"external_key": null,
"first_name": "Test",
"middle_name_glob": null,
"last_name": "Customer",
"name_suffix": null,
"title": "",
"email": "keith@chargeover.com",
"phone": "",
"write_datetime": "2014-12-02 14:02:23",
"mod_datetime": "2014-12-02 14:25:13",
"name": "Test Customer",
"display_as": "Test Customer",
"username": "keith@chargeover.com"
}
},
"security_token": "xhtfLO9TumgUs6QRpzr8cKMFPHqAnad2"
}
A subscription is created
This event occurs when a package is first created.
Note these fields:
context_str = package
context_id = ...
(this will be thepackage_id
value for the newly created package)event = insert
A subscription is created
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: Q3putY0lSXn9OKNg15a4x8sHmBUjDWVh
X-Chargeover-Source: example.chargeover.com
{
"context_str": "package",
"context_id": 261,
"event": "insert",
"data": {
"package": {
"terms_id": 3,
"currency_id": 1,
"external_key": null,
"nickname": "",
"paymethod": "inv",
"paycycle": "mon",
"bill_addr1": null,
"bill_addr2": null,
"bill_addr3": null,
"bill_city": null,
"bill_state": null,
"bill_postcode": null,
"bill_country": null,
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"creditcard_id": null,
"ach_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"write_datetime": "2014-06-13 13:39:43",
"mod_datetime": null,
"suspendfrom_datetime": null,
"suspendto_datetime": null,
"cancel_datetime": null,
"holduntil_datetime": null,
"currency_symbol": "$",
"currency_iso4217": "USD",
"amount_collected": 0,
"amount_invoiced": 0,
"amount_due": 0,
"next_invoice_datetime": "2014-06-14 00:00:01",
"package_id": 261,
"customer_id": 1,
"package_status_id": 2,
"package_status_name": "Current",
"package_status_str": "active-current",
"package_status_state": "a"
},
"customer": {
"superuser_id": 1,
"external_key": "120051",
"company": "The Test Company, LLC",
"bill_addr1": "1324 West Atlantic Blvd",
"bill_addr2": "Suite D",
"bill_addr3": "",
"bill_city": "Coral Springs",
"bill_state": "FL",
"bill_postcode": "33071",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"currency_id": 1,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"write_datetime": "2013-10-23 16:44:57",
"write_ipaddr": "173.162.78.89",
"mod_datetime": "2014-06-07 00:39:45",
"mod_ipaddr": "127.0.0.1",
"paid": 3400,
"total": 4103.5,
"balance": 703.5,
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "The Test Company, LLC",
"ship_block": "",
"bill_block": "1234 West Atlantic Blvd\nSuite D\nCoral Springs FL 33071\nUnited States",
"superuser_name": "Keith Palmer",
"superuser_first_name": "Keith",
"superuser_last_name": "Palmer",
"superuser_phone": "",
"superuser_email": "",
"customer_id": 1
},
"user": {
"user_id": 1,
"external_key": null,
"first_name": "Keith",
"middle_name_glob": null,
"last_name": "Palmer",
"name_suffix": null,
"title": "",
"email": "",
"phone": "",
"write_datetime": "2013-10-23 16:44:57",
"mod_datetime": "2014-04-25 08:23:56",
"name": "Keith Palmer",
"display_as": "Keith Palmer",
"username": "keith@chargeover.com"
}
},
"security_token": "Q3putY0lSXn9OKNg15a4x8sHmBUjDWVh"
}
A subscription is updated
This event occurs when a package is updated.
Note that this event will NOT occur if an individual line on a package is added, upgraded/downgraded, or removed.
For those events, please listen for the package upgrade
event.
Note these fields:
context_str = package
context_id = ...
(this will be thepackage_id
of the package)event = update
A subscription is updated
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: 9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo
X-Chargeover-Source: example.chargeover.com
{
"context_str": "package",
"context_id": "7",
"event": "update",
"data": {
"package": {
"terms_id": 2,
"class_id": null,
"admin_id": 3,
"currency_id": 1,
"external_key": null,
"token": "w8933juxg4m1",
"nickname": "",
"paymethod": "inv",
"paycycle": "dly",
"bill_addr1": null,
"bill_addr2": null,
"bill_addr3": null,
"bill_city": null,
"bill_state": null,
"bill_postcode": null,
"bill_country": null,
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"creditcard_id": null,
"ach_id": null,
"tokenized_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"write_datetime": "2017-06-12 11:28:43",
"mod_datetime": "2017-06-15 12:18:06",
"start_datetime": "2017-06-12 11:28:43",
"suspendfrom_datetime": null,
"suspendto_datetime": null,
"cancel_datetime": null,
"holduntil_datetime": null,
"terms_name": "Net 30",
"terms_days": 30,
"currency_symbol": "$",
"currency_iso4217": "USD",
"amount_collected": 0,
"amount_invoiced": 0,
"amount_due": 0,
"is_overdue": false,
"days_overdue": 0,
"next_invoice_datetime": "2017-06-16 12:18:06",
"cancel_reason": null,
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/package\/view\/7",
"package_id": 7,
"customer_id": 2,
"package_status_id": 2,
"package_status_name": "Current",
"package_status_str": "active-current",
"package_status_state": "a",
"line_items": [
{
"item_id": 249,
"tierset_id": 7,
"admin_id": null,
"external_key": null,
"nickname": "",
"descrip": "Test item",
"line_quantity": 1,
"trial_days": 0,
"trial_recurs": "0",
"trial_units": 0,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"subscribe_datetime": "2017-06-12 11:28:43",
"subscribe_prorate_from_datetime": null,
"subscribe_prorate_to_datetime": null,
"cancel_datetime": null,
"expire_datetime": null,
"expire_recurs": null,
"item_name": "Test Plan ",
"item_external_key": null,
"item_accounting_sku": null,
"item_token": "53g1u812b4lq",
"item_type": "service",
"item_units": null,
"item_is_usage": false,
"line_item_id": 7,
"package_id": 7
}
]
},
"customer": {
"superuser_id": 348,
"external_key": null,
"token": "7b4ouwl31vd1",
"company": "Test Customer",
"bill_addr1": "123 Oak Street",
"bill_addr2": "",
"bill_addr3": "",
"bill_city": "City",
"bill_state": "State",
"bill_postcode": "45467",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"terms_id": 6,
"class_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"admin_id": 3,
"campaign_id": null,
"currency_id": 1,
"language_id": 1,
"brand_id": 1,
"no_taxes": false,
"no_dunning": false,
"write_datetime": "2017-03-31 10:58:34",
"write_ipaddr": "127.0.0.1",
"mod_datetime": "2017-06-13 12:49:26",
"mod_ipaddr": "127.0.0.1",
"terms_name": "Net 90",
"terms_days": 90,
"paid": 21.9,
"total": 21.9,
"balance": 0,
"url_paymethodlink": "http:\/\/dev.chargeover.com\/r\/paymethod\/i\/7b4ouwl31vd1",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/customer\/view\/2",
"admin_name": "Karli Palmer",
"admin_email": "karli@chargeover.com",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "Test Customer",
"ship_block": "",
"bill_block": "123 Oak Street\nCity State 45467\nUnited States",
"superuser_name": "Test Customer",
"superuser_first_name": "Test",
"superuser_last_name": "Customer",
"superuser_phone": "",
"superuser_email": "customer@example.com",
"superuser_token": "3w1d88my0a8r",
"customer_id": 2,
"invoice_delivery": "email",
"dunning_delivery": "email",
"customer_status_id": 1,
"customer_status_name": "Current",
"customer_status_str": "active-current",
"customer_status_state": "a",
"superuser_username": "teQdRnjFTPNg"
},
"user": {
"user_id": 348,
"external_key": null,
"first_name": "Test",
"middle_name_glob": null,
"last_name": "Customer",
"name_suffix": null,
"title": "",
"email": "customer@example.com",
"token": "3w1d88my0a8r",
"phone": "",
"user_type_id": 1,
"write_datetime": "2017-03-31 10:58:34",
"mod_datetime": "2017-06-13 12:48:56",
"name": "Test Customer",
"display_as": "Test Customer",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/contact\/view\/348",
"user_type_name": "Billing",
"username": "teQdRnjFTPNg",
"customer_id": 2
}
},
"security_token": "9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo"
}
A subscription has generated an invoice
This event occurs when an invoice is generated from a package.
Note these fields:
context_str = package
context_id = ...
(this will be thepackage_id
of the package)event = generate
A subscription has generated an invoice
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: 9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo
X-Chargeover-Source: example.chargeover.com
{
"context_str": "package",
"context_id": "9",
"event": "generate",
"data": {
"package": {
"terms_id": 3,
"class_id": null,
"admin_id": 3,
"currency_id": 1,
"external_key": null,
"token": "zt515p3x0489",
"nickname": "",
"paymethod": "inv",
"paycycle": "mon",
"bill_addr1": null,
"bill_addr2": null,
"bill_addr3": null,
"bill_city": null,
"bill_state": null,
"bill_postcode": null,
"bill_country": null,
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"creditcard_id": null,
"ach_id": null,
"tokenized_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"write_datetime": "2017-06-15 12:41:22",
"mod_datetime": "2017-06-15 12:41:22",
"start_datetime": "2017-06-15 12:41:22",
"suspendfrom_datetime": null,
"suspendto_datetime": null,
"cancel_datetime": null,
"holduntil_datetime": null,
"terms_name": "Due on Receipt",
"terms_days": 0,
"currency_symbol": "$",
"currency_iso4217": "USD",
"amount_collected": 0,
"amount_invoiced": 0,
"amount_due": 0,
"is_overdue": false,
"days_overdue": 0,
"next_invoice_datetime": "2017-06-16 00:00:01",
"cancel_reason": null,
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/package\/view\/9",
"package_id": 9,
"customer_id": 8,
"package_status_id": 2,
"package_status_name": "Current",
"package_status_str": "active-current",
"package_status_state": "a",
"line_items": [
{
"item_id": 1,
"tierset_id": 1,
"admin_id": null,
"external_key": null,
"nickname": "",
"descrip": "This is a ChargeOver test item.",
"line_quantity": 1,
"trial_days": 0,
"trial_recurs": "0",
"trial_units": 0,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"subscribe_datetime": "2017-06-15 12:41:22",
"subscribe_prorate_from_datetime": null,
"subscribe_prorate_to_datetime": null,
"cancel_datetime": null,
"expire_datetime": null,
"expire_recurs": null,
"item_name": "My Test Service Plan",
"item_external_key": null,
"item_accounting_sku": null,
"item_token": "3405ed3c8ef2",
"item_type": "service",
"item_units": "",
"item_is_usage": false,
"line_item_id": 9,
"package_id": 9
}
]
},
"customer": {
"superuser_id": 354,
"external_key": null,
"token": "2ygprd9569t4",
"company": "John Doe's Company, LLC",
"bill_addr1": "34 Doe Street",
"bill_addr2": "",
"bill_addr3": "",
"bill_city": "City",
"bill_state": "New State",
"bill_postcode": "",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"terms_id": 3,
"class_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"admin_id": 3,
"campaign_id": null,
"currency_id": 1,
"language_id": 1,
"brand_id": 1,
"no_taxes": false,
"no_dunning": false,
"write_datetime": "2017-06-08 15:39:59",
"write_ipaddr": "127.0.0.1",
"mod_datetime": "2017-06-13 12:40:51",
"mod_ipaddr": "127.0.0.1",
"terms_name": "Due on Receipt",
"terms_days": 0,
"paid": 10.95,
"total": 82.85,
"balance": 71.9,
"url_paymethodlink": "http:\/\/dev.chargeover.com\/r\/paymethod\/i\/2ygprd9569t4",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/customer\/view\/8",
"admin_name": "Karli Palmer",
"admin_email": "karli@chargeover.com",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "John Doe's Company, LLC",
"ship_block": "",
"bill_block": "34 Doe Street\nCity New State\nUnited States",
"superuser_name": "John Doe",
"superuser_first_name": "John",
"superuser_last_name": "Doe",
"superuser_phone": "",
"superuser_email": "john@example.com",
"superuser_token": "4e7t9r5a03fv",
"customer_id": 8,
"invoice_delivery": "email",
"dunning_delivery": "email",
"customer_status_id": 1,
"customer_status_name": "Current",
"customer_status_str": "active-current",
"customer_status_state": "a",
"superuser_username": "john@example.com"
},
"user": {
"user_id": 354,
"external_key": null,
"first_name": "John",
"middle_name_glob": null,
"last_name": "Doe",
"name_suffix": null,
"title": "",
"email": "john@example.com",
"token": "4e7t9r5a03fv",
"phone": "",
"user_type_id": 1,
"write_datetime": "2017-06-08 15:39:59",
"mod_datetime": "2017-06-08 15:39:59",
"name": "John Doe",
"display_as": "John Doe",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/contact\/view\/354",
"user_type_name": "Billing",
"username": "john@example.com",
"customer_id": 8
}
},
"security_token": "9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo"
}
The invoice cycle for a subscription changes
This event occurs when a package's payment cycle changes (e.g. from monthly to yearly)
Note these fields:
context_str = package
context_id = ...
(this will be thepackage_id
of the package)event = paycycle
The invoice cycle for a subscription changes
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: 9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo
X-Chargeover-Source: example.chargeover.com
{
"context_str": "package",
"context_id": "7",
"event": "paycycle",
"data": {
"package": {
"terms_id": 2,
"class_id": null,
"admin_id": 3,
"currency_id": 1,
"external_key": null,
"token": "w8933juxg4m1",
"nickname": "",
"paymethod": "inv",
"paycycle": "mon",
"bill_addr1": null,
"bill_addr2": null,
"bill_addr3": null,
"bill_city": null,
"bill_state": null,
"bill_postcode": null,
"bill_country": null,
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"creditcard_id": null,
"ach_id": null,
"tokenized_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"write_datetime": "2017-06-12 11:28:43",
"mod_datetime": "2017-06-15 12:18:06",
"start_datetime": "2017-06-12 11:28:43",
"suspendfrom_datetime": null,
"suspendto_datetime": null,
"cancel_datetime": null,
"holduntil_datetime": null,
"terms_name": "Net 30",
"terms_days": 30,
"currency_symbol": "$",
"currency_iso4217": "USD",
"amount_collected": 0,
"amount_invoiced": 0,
"amount_due": 0,
"is_overdue": false,
"days_overdue": 0,
"next_invoice_datetime": "2017-07-12 00:00:01",
"cancel_reason": null,
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/package\/view\/7",
"package_id": 7,
"customer_id": 2,
"package_status_id": 2,
"package_status_name": "Current",
"package_status_str": "active-current",
"package_status_state": "a",
"line_items": [
{
"item_id": 249,
"tierset_id": 7,
"admin_id": null,
"external_key": null,
"nickname": "",
"descrip": "Test item",
"line_quantity": 1,
"trial_days": 0,
"trial_recurs": "0",
"trial_units": 0,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"subscribe_datetime": "2017-06-12 11:28:43",
"subscribe_prorate_from_datetime": null,
"subscribe_prorate_to_datetime": null,
"cancel_datetime": null,
"expire_datetime": null,
"expire_recurs": null,
"item_name": "Test Plan ",
"item_external_key": null,
"item_accounting_sku": null,
"item_token": "53g1u812b4lq",
"item_type": "service",
"item_units": null,
"item_is_usage": false,
"line_item_id": 7,
"package_id": 7
}
]
},
"customer": {
"superuser_id": 348,
"external_key": null,
"token": "7b4ouwl31vd1",
"company": "Test Customer",
"bill_addr1": "123 Oak Street",
"bill_addr2": "",
"bill_addr3": "",
"bill_city": "City",
"bill_state": "State",
"bill_postcode": "45467",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"terms_id": 6,
"class_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"admin_id": 3,
"campaign_id": null,
"currency_id": 1,
"language_id": 1,
"brand_id": 1,
"no_taxes": false,
"no_dunning": false,
"write_datetime": "2017-03-31 10:58:34",
"write_ipaddr": "127.0.0.1",
"mod_datetime": "2017-06-13 12:49:26",
"mod_ipaddr": "127.0.0.1",
"terms_name": "Net 90",
"terms_days": 90,
"paid": 21.9,
"total": 21.9,
"balance": 0,
"url_paymethodlink": "http:\/\/dev.chargeover.com\/r\/paymethod\/i\/7b4ouwl31vd1",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/customer\/view\/2",
"admin_name": "Karli Palmer",
"admin_email": "karli@chargeover.com",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "Test Customer",
"ship_block": "",
"bill_block": "123 Oak Street\nCity State 45467\nUnited States",
"superuser_name": "Test Customer",
"superuser_first_name": "Test",
"superuser_last_name": "Customer",
"superuser_phone": "",
"superuser_email": "customer@example.com",
"superuser_token": "3w1d88my0a8r",
"customer_id": 2,
"invoice_delivery": "email",
"dunning_delivery": "email",
"customer_status_id": 1,
"customer_status_name": "Current",
"customer_status_str": "active-current",
"customer_status_state": "a",
"superuser_username": "teQdRnjFTPNg"
},
"user": {
"user_id": 348,
"external_key": null,
"first_name": "Test",
"middle_name_glob": null,
"last_name": "Customer",
"name_suffix": null,
"title": "",
"email": "customer@example.com",
"token": "3w1d88my0a8r",
"phone": "",
"user_type_id": 1,
"write_datetime": "2017-03-31 10:58:34",
"mod_datetime": "2017-06-13 12:48:56",
"name": "Test Customer",
"display_as": "Test Customer",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/contact\/view\/348",
"user_type_name": "Billing",
"username": "teQdRnjFTPNg",
"customer_id": 2
}
},
"security_token": "9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo"
}
The pay method for a subscription changes
This event occurs when a package's payment method changes (e.g. from credit card to ACH/bank account)
Note these fields:
context_str = package
context_id = ...
(this will be thepackage_id
of the package)event = paymethod
The pay method for a subscription changes
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: cf64bcbf80c3e65cd813aaaebff32fa7
X-Chargeover-Source: example.chargeover.com
{
"context_str": "package",
"context_id": "542",
"event": "paymethod",
"data": {
"package": {
"terms_id": 2,
"class_id": null,
"admin_id": null,
"currency_id": 1,
"brand_id": 1,
"external_key": null,
"token": "8mv7fnq2q2c2",
"nickname": "",
"paymethod": "crd",
"paycycle": "six",
"bill_addr1": null,
"bill_addr2": null,
"bill_addr3": null,
"bill_city": null,
"bill_state": null,
"bill_postcode": null,
"bill_country": null,
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"creditcard_id": 124,
"ach_id": null,
"tokenized_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": "",
"custom_4": "",
"custom_5": "",
"custom_6": "",
"custom_7": null,
"custom_8": null,
"custom_9": null,
"custom_10": null,
"custom_11": null,
"custom_12": null,
"custom_13": null,
"custom_14": null,
"custom_15": null,
"custom_16": null,
"custom_17": null,
"custom_18": null,
"custom_19": null,
"custom_20": null,
"write_datetime": "2023-01-23T09:50:15-06:00",
"mod_datetime": "2023-01-23T11:05:25-06:00",
"start_datetime": "2023-01-23T09:50:15-06:00",
"suspendfrom_datetime": null,
"suspendto_datetime": null,
"cancel_datetime": null,
"holduntil_datetime": "2023-05-26T00:00:00-05:00",
"terms_name": "Net 30",
"terms_days": 30,
"currency_symbol": "$",
"currency_iso4217": "USD",
"class_name": "",
"brand_name": "My Test Company",
"amount_collected": 0,
"amount_invoiced": 839.07,
"amount_due": 839.07,
"is_overdue": false,
"days_overdue": 0,
"amount_overdue": 0,
"next_invoice_datetime": "2023-05-26T00:00:00-05:00",
"cancel_reason": null,
"paycycle_name": "Every six months",
"paymethod_name": "Credit Card",
"url_self": "https:\/\/dev1.chargeover.com\/admin\/r\/package\/view\/542",
"package_id": 542,
"customer_id": 19753,
"package_status_id": 2,
"mrr": 0,
"arr": 0,
"paymethod_detail": "Visa x0148",
"package_status_name": "Current",
"package_status_str": "active-current",
"package_status_state": "a",
"line_items": [
{
"item_id": 165,
"tierset_id": 4917,
"admin_id": null,
"external_key": null,
"nickname": "",
"descrip": null,
"line_quantity": 1,
"trial_days": 0,
"trial_recurs": 0,
"trial_units": 0,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"custom_7": null,
"custom_8": null,
"custom_9": null,
"custom_10": null,
"custom_11": null,
"custom_12": null,
"custom_13": null,
"custom_14": null,
"custom_15": null,
"custom_16": null,
"custom_17": null,
"custom_18": null,
"custom_19": null,
"custom_20": null,
"subscribe_datetime": "2023-01-23T09:50:15-06:00",
"subscribe_prorate_from_datetime": "2023-01-25T00:00:00-06:00",
"subscribe_prorate_to_datetime": "2023-05-25T23:59:59-05:00",
"cancel_datetime": null,
"expire_datetime": null,
"expire_recurs": null,
"item_name": "Test Product",
"item_external_key": null,
"item_accounting_sku": null,
"item_token": "4emftybcxm40",
"item_type": "service",
"item_units": null,
"item_is_usage": false,
"line_item_id": 1056,
"package_id": 542,
"tierset": {
"tierset_id": 4917,
"currency_id": 1,
"setup": 0,
"base": 1269,
"minimum": 0,
"percent": 0,
"paycycle": "evy",
"pricemodel": "fla",
"write_datetime": "2023-01-23T09:50:05-06:00",
"mod_datetime": "2023-01-23T09:50:05-06:00",
"currency_symbol": "$",
"currency_iso4217": "USD",
"setup_formatted": "$ 0.00",
"base_formatted": "$ 1269.00",
"minimum_formatted": "$ 0.00",
"percent_formatted": "0 %",
"pricemodel_desc": "Flat Pricing (example: $X dollars every billing cycle)",
"tiers": []
}
}
]
},
"customer": {
"superuser_id": 19994,
"external_key": null,
"token": "t8ft0pd43xce",
"company": "John Doe's Company, LLC",
"bill_addr1": null,
"bill_addr2": null,
"bill_addr3": null,
"bill_city": null,
"bill_state": null,
"bill_postcode": null,
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": "United States",
"ship_notes": null,
"terms_id": 2,
"class_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"custom_7": null,
"custom_8": null,
"custom_9": null,
"custom_10": null,
"custom_11": null,
"custom_12": null,
"custom_13": null,
"custom_14": null,
"custom_15": null,
"custom_16": null,
"custom_17": null,
"custom_18": null,
"custom_19": null,
"custom_20": null,
"admin_id": null,
"campaign_id": null,
"currency_id": 1,
"language_id": 1,
"brand_id": 1,
"default_paymethod": null,
"default_creditcard_id": null,
"default_ach_id": null,
"tax_ident": "",
"no_taxes": false,
"no_dunning": false,
"no_latefees": false,
"write_datetime": "2023-01-23T09:28:43-06:00",
"write_ipaddr": "192.168.0.1",
"mod_datetime": "2023-01-23T09:28:43-06:00",
"mod_ipaddr": "192.168.0.1",
"terms_name": "Net 30",
"terms_days": 30,
"class_name": "",
"paid": 0,
"total": 2617.21,
"balance": 2617.21,
"url_statementlink": "https:\/\/dev1.chargeover.com\/r\/statement\/view\/t8ft0pd43xce",
"url_paymethodlink": "https:\/\/dev1.chargeover.com\/r\/paymethod\/i\/t8ft0pd43xce",
"url_self": "https:\/\/dev1.chargeover.com\/admin\/r\/customer\/view\/19753",
"admin_name": "",
"admin_email": "",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "John Doe's Company, LLC",
"ship_block": "United States",
"bill_block": "United States",
"superuser_name": "Test Prorate",
"superuser_first_name": "John",
"superuser_last_name": "Doe",
"superuser_phone": null,
"superuser_email": null,
"superuser_token": "bvhbgfpbmoik",
"customer_id": 19753,
"parent_customer_id": null,
"invoice_delivery": "email",
"dunning_delivery": "email",
"customer_status_id": 1,
"mrr": 523,
"arr": 6276,
"customer_status_name": "Current",
"customer_status_str": "active-current",
"customer_status_state": "a",
"superuser_username": "EUbN07tnFVrM"
},
"user": {
"user_id": 19994,
"external_key": null,
"first_name": "John",
"middle_name_glob": null,
"last_name": "Doe",
"name_suffix": null,
"title": null,
"email": null,
"token": "bvhbgfpbmoik",
"phone": null,
"mobile": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"user_type_id": 1,
"write_datetime": "2023-01-23T09:28:43-06:00",
"mod_datetime": "2023-01-23T09:28:43-06:00",
"name": "Test Prorate",
"display_as": "Test Prorate",
"url_self": "https:\/\/dev1.chargeover.com\/admin\/r\/contact\/view\/19994",
"user_type_name": "Billing",
"username": "EUbN07tnFVrM",
"password": null,
"customer_id": 19753
}
},
"security_token": "cf64bcbf80c3e65cd813aaaebff32fa7"
}
A subscription is cancelled
This event occurs when a package is cancelled.
Note these fields:
context_str = package
context_id = ...
(this will be thepackage_id
of the package)event = cancel
A subscription is cancelled
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: 9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo
X-Chargeover-Source: example.chargeover.com
{
"context_str": "package",
"context_id": "2",
"event": "cancel",
"data": {
"package": {
"terms_id": 2,
"class_id": null,
"admin_id": 3,
"currency_id": 1,
"external_key": null,
"token": "10v34wl1f096",
"nickname": "",
"paymethod": "ach",
"paycycle": "mon",
"bill_addr1": null,
"bill_addr2": null,
"bill_addr3": null,
"bill_city": null,
"bill_state": null,
"bill_postcode": null,
"bill_country": null,
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"creditcard_id": null,
"ach_id": 1,
"tokenized_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"write_datetime": "2017-04-11 16:56:50",
"mod_datetime": "2017-04-11 16:56:50",
"start_datetime": "2017-04-11 16:56:50",
"suspendfrom_datetime": null,
"suspendto_datetime": null,
"cancel_datetime": "2017-06-15 11:12:46",
"holduntil_datetime": null,
"terms_name": "Net 30",
"terms_days": 30,
"currency_symbol": "$",
"currency_iso4217": "USD",
"amount_collected": 32.85,
"amount_invoiced": 32.85,
"amount_due": 0,
"is_overdue": false,
"days_overdue": 0,
"next_invoice_datetime": null,
"cancel_reason": "Unknown or unspecified reason",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/package\/view\/2",
"package_id": 2,
"customer_id": 3,
"package_status_id": 5,
"package_status_name": "Canceled manually",
"package_status_str": "canceled-manual",
"package_status_state": "c",
"line_items": [
{
"item_id": 1,
"tierset_id": 1,
"admin_id": null,
"external_key": null,
"nickname": "",
"descrip": "This is a ChargeOver test item.",
"line_quantity": 3,
"trial_days": 0,
"trial_recurs": "0",
"trial_units": 0,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"subscribe_datetime": "2017-04-11 16:56:50",
"subscribe_prorate_from_datetime": null,
"subscribe_prorate_to_datetime": null,
"cancel_datetime": "2017-06-15 11:12:46",
"expire_datetime": null,
"expire_recurs": null,
"item_name": "My Test Service Plan",
"item_external_key": null,
"item_accounting_sku": null,
"item_token": "3405ed3c8ef2",
"item_type": "service",
"item_units": "",
"item_is_usage": false,
"line_item_id": 2,
"package_id": 2
}
]
},
"customer": {
"superuser_id": 355,
"external_key": null,
"token": "cw3457d7szn7",
"company": "Test Customer , LLC",
"bill_addr1": "45 Somewhere Avenue",
"bill_addr2": "",
"bill_addr3": "",
"bill_city": "City",
"bill_state": "State",
"bill_postcode": "",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"terms_id": 1,
"class_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"admin_id": 3,
"campaign_id": null,
"currency_id": 1,
"language_id": 1,
"brand_id": 1,
"no_taxes": false,
"no_dunning": false,
"write_datetime": "2017-04-06 14:35:18",
"write_ipaddr": "127.0.0.1",
"mod_datetime": "2017-06-13 12:53:13",
"mod_ipaddr": "127.0.0.1",
"terms_name": "Net 15",
"terms_days": 15,
"paid": 65.7,
"total": 90.7,
"balance": 25,
"url_paymethodlink": "http:\/\/dev.chargeover.com\/r\/paymethod\/i\/cw3457d7szn7",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/customer\/view\/3",
"admin_name": "Karli Palmer",
"admin_email": "karli@chargeover.com",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "Test Customer , LLC",
"ship_block": "",
"bill_block": "45 Somewhere Avenue\nCity State\nUnited States",
"superuser_name": "Jane Doe",
"superuser_first_name": "Jane",
"superuser_last_name": "Doe",
"superuser_phone": "",
"superuser_email": "",
"superuser_token": "xp40296s7en2",
"customer_id": 3,
"invoice_delivery": "email",
"dunning_delivery": "email",
"customer_status_id": 1,
"customer_status_name": "Current",
"customer_status_str": "active-current",
"customer_status_state": "a",
"superuser_username": "prt8anm9wqfd"
},
"user": {
"user_id": 355,
"external_key": null,
"first_name": "Jane",
"middle_name_glob": null,
"last_name": "Doe",
"name_suffix": null,
"title": "",
"email": "",
"token": "xp40296s7en2",
"phone": "",
"user_type_id": 1,
"write_datetime": "2017-06-13 12:52:40",
"mod_datetime": "2017-06-13 12:52:40",
"name": "Jane Doe",
"display_as": "Jane Doe",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/contact\/view\/355",
"user_type_name": "Billing",
"username": "prt8anm9wqfd",
"customer_id": 3
}
},
"security_token": "9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo"
}
A subscription is upgraded or downgraded
This event occurs when a package is upgraded or downgraded (i.e. a line on the package is added, removed, or changed in any way)
Note these fields:
context_str = package
context_id = ...
(this will be thepackage_id
of the package)event = upgrade
A subscription is upgraded or downgraded
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: Ma4hWVUNLx0O5zfmJSjQ3AgwpRdY7qu1
X-Chargeover-Source: example.chargeover.com
{
"context_str": "package",
"context_id": "670",
"event": "upgrade",
"data": {
"package": {
"terms_id": 2,
"currency_id": 1,
"external_key": null,
"token": "p0julwxm3vbn",
"nickname": "",
"paymethod": "inv",
"paycycle": "mon",
"bill_addr1": null,
"bill_addr2": null,
"bill_addr3": null,
"bill_city": null,
"bill_state": null,
"bill_postcode": null,
"bill_country": null,
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"creditcard_id": null,
"ach_id": null,
"tokenized_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"write_datetime": "2014-12-20 12:58:39",
"mod_datetime": "2014-12-20 12:59:35",
"suspendfrom_datetime": null,
"suspendto_datetime": null,
"cancel_datetime": null,
"holduntil_datetime": null,
"currency_symbol": "$",
"currency_iso4217": "USD",
"amount_collected": 0,
"amount_invoiced": 10.95,
"amount_due": 10.95,
"next_invoice_datetime": "2015-01-20 00:00:01",
"package_id": 670,
"customer_id": 160,
"package_status_id": 2,
"package_status_name": "Current",
"package_status_str": "active-current",
"package_status_state": "a"
},
"customer": {
"superuser_id": 370,
"external_key": null,
"token": "lrqtxpimw5vo",
"company": "John Doe's Company, LLC",
"bill_addr1": "72 E Blue Grass Road",
"bill_addr2": "Suite D",
"bill_addr3": null,
"bill_city": "Mt Pleasant",
"bill_state": "MI",
"bill_postcode": "48858",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"currency_id": 1,
"write_datetime": "2014-12-20 12:29:31",
"write_ipaddr": "172.16.4.176",
"mod_datetime": "2014-12-20 12:29:31",
"mod_ipaddr": "172.16.4.176",
"paid": 0,
"total": 52.85,
"balance": 52.85,
"url_paymethodlink": "http:\/\/dev.chargeover.com\/signup\/r\/paymethod\/i\/lrqtxpimw5vo",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "John Doe's Company, LLC",
"ship_block": "",
"bill_block": "72 E Blue Grass Road\nSuite D\nMt Pleasant MI 48858\nUnited States",
"superuser_name": "John Doe",
"superuser_first_name": "John",
"superuser_last_name": "Doe",
"superuser_phone": "860-634-1602",
"superuser_email": "johndoe@chargeover.com",
"customer_id": 160
},
"user": {
"user_id": 370,
"external_key": null,
"first_name": "John",
"middle_name_glob": null,
"last_name": "Doe",
"name_suffix": null,
"title": "",
"email": "johndoe@chargeover.com",
"phone": "860-634-1602",
"write_datetime": "2014-12-20 12:29:31",
"mod_datetime": "2014-12-20 12:29:31",
"name": "John Doe",
"display_as": "John Doe",
"username": "johndoe@chargeover.com"
},
"old_line_item": {
"item_id": 1,
"tierset_id": 1,
"external_key": null,
"nickname": "",
"descrip": "This is a ChargeOver test item.",
"custom_1": null,
"custom_2": null,
"custom_3": null,
"subscribe_datetime": "2014-12-20 12:58:39",
"cancel_datetime": "2014-12-20 12:59:35",
"expire_datetime": null,
"expire_recurs": null,
"license": "",
"item_name": "My Test Service Plan",
"item_external_key": null,
"item_accounting_sku": null,
"item_units": "",
"line_item_id": 713
},
"new_line_item": {
"item_id": 1,
"tierset_id": 1,
"external_key": null,
"nickname": "",
"descrip": "Updated line description.",
"custom_1": null,
"custom_2": null,
"custom_3": null,
"subscribe_datetime": "2014-12-20 12:59:35",
"cancel_datetime": null,
"expire_datetime": null,
"expire_recurs": null,
"license": "",
"item_name": "My Test Service Plan",
"item_external_key": null,
"item_accounting_sku": null,
"item_units": "",
"line_item_id": 714
}
},
"security_token": "Ma4hWVUNLx0O5zfmJSjQ3AgwpRdY7qu1"
}
A subscription is suspended
This event occurs when a package is suspended.
Note these fields:
context_str = package
context_id = ...
(this will be thepackage_id
of the package)event = suspend
A subscription is suspended
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: 9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo
X-Chargeover-Source: example.chargeover.com
{
"context_str": "package",
"context_id": "38",
"event": "suspend",
"data": {
"package": {
"terms_id": 2,
"class_id": null,
"admin_id": 4,
"currency_id": 1,
"brand_id": 1,
"external_key": null,
"token": "4u2y9nm541dg",
"nickname": "",
"paymethod": "crd",
"paycycle": "mon",
"bill_addr1": null,
"bill_addr2": null,
"bill_addr3": null,
"bill_city": null,
"bill_state": null,
"bill_postcode": null,
"bill_country": null,
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"creditcard_id": 18,
"ach_id": null,
"tokenized_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"write_datetime": "2018-03-01 12:02:07",
"mod_datetime": "2018-03-01 12:02:07",
"start_datetime": "2018-03-01 12:02:07",
"suspendfrom_datetime": "2018-04-13 10:19:42",
"suspendto_datetime": "2038-01-01 00:00:01",
"cancel_datetime": null,
"holduntil_datetime": null,
"terms_name": "Net 30",
"terms_days": 30,
"currency_symbol": "$",
"currency_iso4217": "USD",
"class_name": "",
"amount_collected": 50,
"amount_invoiced": 50,
"amount_due": 0,
"is_overdue": false,
"days_overdue": 0,
"next_invoice_datetime": null,
"cancel_reason": null,
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/package\/view\/38",
"package_id": 38,
"customer_id": 37,
"package_status_id": 8,
"package_status_name": "Suspended",
"package_status_str": "suspended-suspended",
"package_status_state": "s",
"line_items": [
{
"item_id": 257,
"tierset_id": 24,
"admin_id": null,
"external_key": null,
"nickname": "",
"descrip": "TEXTING SERVICE:\r\n500 outbound\/sent text messages a month.",
"line_quantity": 1,
"trial_days": 0,
"trial_recurs": 0,
"trial_units": 0,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"subscribe_datetime": "2018-03-01 12:02:07",
"subscribe_prorate_from_datetime": null,
"subscribe_prorate_to_datetime": null,
"cancel_datetime": null,
"expire_datetime": null,
"expire_recurs": null,
"item_name": "500 Text Messages Monthly Service",
"item_external_key": null,
"item_accounting_sku": null,
"item_token": "28e4wuaik092",
"item_type": "service",
"item_units": "",
"item_is_usage": false,
"line_item_id": 47,
"package_id": 38
}
]
},
"customer": {
"superuser_id": 386,
"external_key": null,
"token": "46661z16tliu",
"company": "Connecticut Flooring Company",
"bill_addr1": "74 Cowles Avenue",
"bill_addr2": "",
"bill_addr3": "",
"bill_city": "Willington",
"bill_state": "CT",
"bill_postcode": "06279",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"terms_id": 2,
"class_id": 0,
"custom_1": "",
"custom_2": "",
"custom_3": "",
"custom_4": null,
"custom_5": null,
"custom_6": null,
"admin_id": 4,
"campaign_id": null,
"currency_id": 1,
"language_id": 1,
"brand_id": 1,
"default_paymethod": "crd",
"default_creditcard_id": 18,
"default_ach_id": null,
"tax_ident": "",
"no_taxes": false,
"no_dunning": false,
"write_datetime": "2018-02-22 10:52:51",
"write_ipaddr": "127.0.0.1",
"mod_datetime": "2018-03-01 11:56:38",
"mod_ipaddr": "127.0.0.1",
"terms_name": "Net 30",
"terms_days": 30,
"class_name": "",
"paid": 1763,
"total": 3243,
"balance": 1480,
"url_statementlink": "http:\/\/dev.chargeover.com\/r\/statement\/view\/46661z16tliu",
"url_paymethodlink": "http:\/\/dev.chargeover.com\/r\/paymethod\/i\/46661z16tliu",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/customer\/view\/37",
"admin_name": "Dave Tyson",
"admin_email": "dave@example.com",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "Connecticut Flooring Company",
"ship_block": "",
"bill_block": "74 Cowles Avenue\nWillington CT 06279\nUnited States",
"superuser_name": "Karli Marie",
"superuser_first_name": "Karli",
"superuser_last_name": "Marie",
"superuser_phone": "",
"superuser_email": "karli@example.com",
"superuser_token": "gdz737x5ye81",
"customer_id": 37,
"parent_customer_id": null,
"invoice_delivery": "email",
"dunning_delivery": "email",
"customer_status_id": 1,
"customer_status_name": "Current",
"customer_status_str": "active-current",
"customer_status_state": "a",
"superuser_username": "dCMntSrhFQB7"
},
"user": {
"user_id": 386,
"external_key": null,
"first_name": "Karli",
"middle_name_glob": null,
"last_name": "Marie",
"name_suffix": null,
"title": "",
"email": "karli@example.com",
"token": "gdz737x5ye81",
"phone": "",
"user_type_id": 1,
"write_datetime": "2018-02-22 10:52:51",
"mod_datetime": "2018-03-01 11:57:09",
"name": "Karli Marie",
"display_as": "Karli Marie",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/contact\/view\/386",
"user_type_name": "Billing",
"username": "dCMntSrhFQB7",
"customer_id": 37
}
},
"security_token": "9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo"
}
A subscription is unsuspended
This event occurs when a package is unsuspended (removed from a suspended state).
Note these fields:
context_str = package
context_id = ...
(this will be thepackage_id
of the package)event = unsuspend
A subscription is unsuspended
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: 9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo
X-Chargeover-Source: example.chargeover.com
{
"context_str": "package",
"context_id": "37",
"event": "unsuspend",
"data": {
"package": {
"terms_id": 2,
"class_id": 0,
"admin_id": 3,
"currency_id": 1,
"brand_id": 1,
"external_key": null,
"token": "c7xpuko238ry",
"nickname": "",
"paymethod": "inv",
"paycycle": "mon",
"bill_addr1": null,
"bill_addr2": null,
"bill_addr3": null,
"bill_city": null,
"bill_state": null,
"bill_postcode": null,
"bill_country": null,
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"creditcard_id": null,
"ach_id": null,
"tokenized_id": null,
"custom_1": "",
"custom_2": "",
"custom_3": "",
"custom_4": null,
"custom_5": null,
"write_datetime": "2018-03-01 11:27:59",
"mod_datetime": "2018-03-01 11:59:20",
"start_datetime": "2018-03-01 11:27:59",
"suspendfrom_datetime": null,
"suspendto_datetime": null,
"cancel_datetime": null,
"holduntil_datetime": null,
"terms_name": "Net 30",
"terms_days": 30,
"currency_symbol": "$",
"currency_iso4217": "USD",
"class_name": "",
"amount_collected": 1060,
"amount_invoiced": 1060,
"amount_due": 0,
"is_overdue": false,
"days_overdue": 0,
"next_invoice_datetime": "2018-05-01 00:00:01",
"cancel_reason": null,
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/package\/view\/37",
"package_id": 37,
"customer_id": 37,
"package_status_id": 2,
"package_status_name": "Current",
"package_status_str": "active-current",
"package_status_state": "a",
"line_items": [
{
"item_id": 258,
"tierset_id": 27,
"admin_id": null,
"external_key": null,
"nickname": "",
"descrip": "Business Federal Income Tax Return - Standard Client",
"line_quantity": 1,
"trial_days": 0,
"trial_recurs": 0,
"trial_units": 0,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"subscribe_datetime": "2018-03-01 11:27:59",
"subscribe_prorate_from_datetime": null,
"subscribe_prorate_to_datetime": null,
"cancel_datetime": null,
"expire_datetime": null,
"expire_recurs": null,
"item_name": "Business Federal Tax Return - Standard",
"item_external_key": null,
"item_accounting_sku": null,
"item_token": "z48kw2colj7y",
"item_type": "service",
"item_units": "",
"item_is_usage": false,
"line_item_id": 46,
"package_id": 37
}
]
},
"customer": {
"superuser_id": 386,
"external_key": null,
"token": "46661z16tliu",
"company": "Connecticut Flooring Company",
"bill_addr1": "74 Cowles Avenue",
"bill_addr2": "",
"bill_addr3": "",
"bill_city": "Willington",
"bill_state": "CT",
"bill_postcode": "06279",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"terms_id": 2,
"class_id": 0,
"custom_1": "",
"custom_2": "",
"custom_3": "",
"custom_4": null,
"custom_5": null,
"custom_6": null,
"admin_id": 4,
"campaign_id": null,
"currency_id": 1,
"language_id": 1,
"brand_id": 1,
"default_paymethod": "crd",
"default_creditcard_id": 18,
"default_ach_id": null,
"tax_ident": "",
"no_taxes": false,
"no_dunning": false,
"write_datetime": "2018-02-22 10:52:51",
"write_ipaddr": "127.0.0.1",
"mod_datetime": "2018-03-01 11:56:38",
"mod_ipaddr": "127.0.0.1",
"terms_name": "Net 30",
"terms_days": 30,
"class_name": "",
"paid": 1763,
"total": 3243,
"balance": 1480,
"url_statementlink": "http:\/\/dev.chargeover.com\/r\/statement\/view\/46661z16tliu",
"url_paymethodlink": "http:\/\/dev.chargeover.com\/r\/paymethod\/i\/46661z16tliu",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/customer\/view\/37",
"admin_name": "Dave Tyson",
"admin_email": "dave@example.com",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "Connecticut Flooring Company",
"ship_block": "",
"bill_block": "74 Cowles Avenue\nWillington CT 06279\nUnited States",
"superuser_name": "Karli Marie",
"superuser_first_name": "Karli",
"superuser_last_name": "Marie",
"superuser_phone": "",
"superuser_email": "karli@example.com",
"superuser_token": "gdz737x5ye81",
"customer_id": 37,
"parent_customer_id": null,
"invoice_delivery": "email",
"dunning_delivery": "email",
"customer_status_id": 1,
"customer_status_name": "Current",
"customer_status_str": "active-current",
"customer_status_state": "a",
"superuser_username": "dCMntSrhFQB7"
},
"user": {
"user_id": 386,
"external_key": null,
"first_name": "Karli",
"middle_name_glob": null,
"last_name": "Marie",
"name_suffix": null,
"title": "",
"email": "karli@example.com",
"token": "gdz737x5ye81",
"phone": "",
"user_type_id": 1,
"write_datetime": "2018-02-22 10:52:51",
"mod_datetime": "2018-03-01 11:57:09",
"name": "Karli Marie",
"display_as": "Karli Marie",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/contact\/view\/386",
"user_type_name": "Billing",
"username": "dCMntSrhFQB7",
"customer_id": 37
}
},
"security_token": "9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo"
}
The nightly event occurs
This is an event that is kicked off nightly for each subscription.
This event only occurs for active subscriptions. Cancelled subscriptions will not fire this event.
Internally, this event is used for updating caches, consistency checks, scheduled events for subscriptions, etc.
Externally, you can choose to hook into this event and use it for whatever you would like.
Note these fields:
context_str = package
context_id = ...
(this will be thepackage_id
of the subscription)event = nightly
The nightly event occurs
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: 9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo
X-Chargeover-Source: example.chargeover.com
{
"context_str": "package",
"context_id": "25",
"event": "nightly",
"data": {
"package": {
"terms_id": 2,
"class_id": null,
"admin_id": 3,
"currency_id": 1,
"brand_id": 1,
"external_key": null,
"token": "67obsv258i2a",
"nickname": "",
"paymethod": "ach",
"paycycle": "mon",
"bill_addr1": null,
"bill_addr2": null,
"bill_addr3": null,
"bill_city": null,
"bill_state": null,
"bill_postcode": null,
"bill_country": null,
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"creditcard_id": null,
"ach_id": 5,
"tokenized_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"write_datetime": "2017-11-27 15:24:38",
"mod_datetime": "2017-11-27 15:24:38",
"start_datetime": "2017-11-27 15:24:38",
"suspendfrom_datetime": null,
"suspendto_datetime": null,
"cancel_datetime": null,
"holduntil_datetime": null,
"terms_name": "Net 30",
"terms_days": 30,
"currency_symbol": "$",
"currency_iso4217": "USD",
"class_name": "",
"amount_collected": 600,
"amount_invoiced": 600,
"amount_due": 0,
"is_overdue": false,
"days_overdue": 0,
"next_invoice_datetime": "2018-03-27 00:00:01",
"cancel_reason": null,
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/package\/view\/25",
"package_id": 25,
"customer_id": 24,
"package_status_id": 2,
"package_status_name": "Current",
"package_status_str": "active-current",
"package_status_state": "a",
"line_items": [
{
"item_id": 259,
"tierset_id": 26,
"admin_id": null,
"external_key": null,
"nickname": "",
"descrip": "Subscription setup fee",
"line_quantity": 1,
"trial_days": 0,
"trial_recurs": 0,
"trial_units": 0,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"subscribe_datetime": "2017-11-27 15:24:38",
"subscribe_prorate_from_datetime": null,
"subscribe_prorate_to_datetime": null,
"cancel_datetime": null,
"expire_datetime": null,
"expire_recurs": null,
"item_name": "Monthly Service Setup Fee ",
"item_external_key": null,
"item_accounting_sku": null,
"item_token": "wynuc6g849b8",
"item_type": "service",
"item_units": null,
"item_is_usage": false,
"line_item_id": 31,
"package_id": 25
},
{
"item_id": 253,
"tierset_id": 20,
"admin_id": null,
"external_key": null,
"nickname": "",
"descrip": "This is a test plan",
"line_quantity": 1,
"trial_days": 0,
"trial_recurs": 0,
"trial_units": 0,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"subscribe_datetime": "2017-11-27 15:24:38",
"subscribe_prorate_from_datetime": null,
"subscribe_prorate_to_datetime": null,
"cancel_datetime": null,
"expire_datetime": null,
"expire_recurs": null,
"item_name": "Premium Gold Plan",
"item_external_key": null,
"item_accounting_sku": null,
"item_token": "a69y82p1s2jv",
"item_type": "service",
"item_units": "",
"item_is_usage": false,
"line_item_id": 32,
"package_id": 25
}
]
},
"customer": {
"superuser_id": 371,
"external_key": null,
"token": "89vu31psg46e",
"company": "Alexander Sanders",
"bill_addr1": null,
"bill_addr2": null,
"bill_addr3": null,
"bill_city": null,
"bill_state": null,
"bill_postcode": null,
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"terms_id": 2,
"class_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"admin_id": 3,
"campaign_id": null,
"currency_id": 1,
"language_id": 1,
"brand_id": 1,
"default_paymethod": null,
"default_creditcard_id": null,
"default_ach_id": null,
"tax_ident": "",
"no_taxes": false,
"no_dunning": false,
"write_datetime": "2017-09-23 12:58:19",
"write_ipaddr": "127.0.0.1",
"mod_datetime": "2017-09-23 12:58:19",
"mod_ipaddr": "127.0.0.1",
"terms_name": "Net 30",
"terms_days": 30,
"class_name": "",
"paid": 1150,
"total": 2650,
"balance": 1500,
"url_statementlink": "http:\/\/dev.chargeover.com\/r\/statement\/view\/89vu31psg46e",
"url_paymethodlink": "http:\/\/dev.chargeover.com\/r\/paymethod\/i\/89vu31psg46e",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/customer\/view\/24",
"admin_name": "ChargeOver Support",
"admin_email": "support@chargeover.com",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "Alexander Sanders",
"ship_block": "",
"bill_block": "United States",
"superuser_name": "Alexander Sanders",
"superuser_first_name": "Alexander",
"superuser_last_name": "Sanders",
"superuser_phone": "678-890-3453",
"superuser_email": "alex@example.com",
"superuser_token": "q159fshr4x53",
"customer_id": 24,
"parent_customer_id": null,
"invoice_delivery": "email",
"dunning_delivery": "email",
"customer_status_id": 2,
"customer_status_name": "Over Due",
"customer_status_str": "active-overdue",
"customer_status_state": "a",
"superuser_username": "alex@example.com"
},
"user": {
"user_id": 371,
"external_key": null,
"first_name": "Alexander",
"middle_name_glob": null,
"last_name": "Sanders",
"name_suffix": null,
"title": "",
"email": "alex@example.com",
"token": "q159fshr4x53",
"phone": "678-890-3453",
"user_type_id": 1,
"write_datetime": "2017-09-23 12:58:19",
"mod_datetime": "2017-09-23 12:58:19",
"name": "Alexander Sanders",
"display_as": "Alexander Sanders",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/contact\/view\/371",
"user_type_name": "Billing",
"username": "alex@example.com",
"customer_id": 24
}
},
"security_token": "9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo"
}
The subscription invoice generation is delayed
This event occurs when a subscription is configured to delay invoicing.
Note these fields:
context_str = package
context_id = ...
(this will be thepackage_id
of the package)event = hold
The subscription invoice generation is delayed
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: Ma4hWVUNLx0O5zfmJSjQ3AgwpRdY7qu2
X-Chargeover-Source: example.chargeover.com
{
"package": {
"terms_id": 2,
"class_id": null,
"admin_id": 1,
"currency_id": 1,
"brand_id": 1,
"external_key": null,
"token": "5fgbmvwuz3p6",
"nickname": "",
"paymethod": "inv",
"paycycle": "mon",
"bill_addr1": null,
"bill_addr2": null,
"bill_addr3": null,
"bill_city": null,
"bill_state": null,
"bill_postcode": null,
"bill_country": null,
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"creditcard_id": null,
"ach_id": null,
"tokenized_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"custom_7": null,
"custom_8": null,
"custom_9": null,
"custom_10": null,
"custom_11": null,
"custom_12": null,
"custom_13": null,
"custom_14": null,
"custom_15": null,
"custom_16": null,
"custom_17": null,
"custom_18": null,
"custom_19": null,
"custom_20": null,
"write_datetime": "2023-02-23 12:40:15",
"mod_datetime": "2023-02-23 12:40:15",
"start_datetime": "2023-02-23 12:40:15",
"suspendfrom_datetime": null,
"suspendto_datetime": null,
"cancel_datetime": null,
"holduntil_datetime": "2023-03-31 00:00:00",
"terms_name": "Net 30",
"terms_days": 30,
"currency_symbol": "$",
"currency_iso4217": "USD",
"class_name": "",
"brand_name": "Default",
"amount_collected": 0,
"amount_invoiced": 0,
"amount_due": 0,
"is_overdue": false,
"days_overdue": 0,
"amount_overdue": 0,
"next_invoice_datetime": "2023-03-31 00:00:00",
"cancel_reason": null,
"paycycle_name": "Monthly",
"paymethod_name": "Invoice",
"url_self": "https:\/\/dev1.chargeover.test\/admin\/r\/package\/view\/37",
"package_id": 37,
"customer_id": 272,
"package_status_id": 2,
"mrr": 0,
"arr": 0,
"paymethod_detail": "Invoice",
"package_status_name": "Current",
"package_status_str": "active-current",
"package_status_state": "a",
"line_items": [
{
"item_id": 1,
"tierset_id": 3,
"admin_id": null,
"external_key": null,
"nickname": "",
"descrip": "This is a ChargeOver test item.",
"line_quantity": 1,
"trial_days": 0,
"trial_recurs": 0,
"trial_units": 0,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"custom_7": null,
"custom_8": null,
"custom_9": null,
"custom_10": null,
"custom_11": null,
"custom_12": null,
"custom_13": null,
"custom_14": null,
"custom_15": null,
"custom_16": null,
"custom_17": null,
"custom_18": null,
"custom_19": null,
"custom_20": null,
"subscribe_datetime": "2023-02-23 12:40:15",
"subscribe_prorate_from_datetime": null,
"subscribe_prorate_to_datetime": null,
"cancel_datetime": null,
"expire_datetime": null,
"expire_recurs": null,
"item_name": "My Test Service Plan",
"item_external_key": null,
"item_accounting_sku": null,
"item_token": "259b2eebdb33",
"item_type": "service",
"item_units": "",
"item_is_usage": false,
"line_item_id": 39,
"package_id": 37,
"tierset": {
"tierset_id": 3,
"currency_id": 1,
"setup": 0,
"base": 10.95,
"minimum": 0,
"percent": 0,
"paycycle": "evy",
"pricemodel": "fla",
"write_datetime": "2022-11-11 10:56:11",
"mod_datetime": "2022-11-11 10:56:11",
"currency_symbol": "$",
"currency_iso4217": "USD",
"setup_formatted": "$ 0.00",
"base_formatted": "$ 10.95",
"minimum_formatted": "$ 0.00",
"percent_formatted": "0 %",
"pricemodel_desc": "Flat Pricing (example: $X dollars every billing cycle)",
"tiers": []
}
}
]
},
"customer": {
"superuser_id": 266,
"external_key": null,
"token": "536pxrz7yn3v",
"company": "Test Customer",
"bill_addr1": null,
"bill_addr2": null,
"bill_addr3": null,
"bill_city": null,
"bill_state": null,
"bill_postcode": null,
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"terms_id": 2,
"class_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"custom_7": null,
"custom_8": null,
"custom_9": null,
"custom_10": null,
"custom_11": null,
"custom_12": null,
"custom_13": null,
"custom_14": null,
"custom_15": null,
"custom_16": null,
"custom_17": null,
"custom_18": null,
"custom_19": null,
"custom_20": null,
"admin_id": 1,
"campaign_id": null,
"currency_id": 1,
"language_id": 1,
"brand_id": 1,
"default_paymethod": null,
"default_creditcard_id": null,
"default_ach_id": null,
"tax_ident": "",
"no_taxes": false,
"no_dunning": false,
"no_latefees": false,
"write_datetime": "2023-02-23 12:38:37",
"write_ipaddr": "172.29.0.1",
"mod_datetime": "2023-02-23 12:38:37",
"mod_ipaddr": "172.29.0.1",
"terms_name": "Net 30",
"terms_days": 30,
"class_name": "",
"paid": 0,
"total": 0,
"balance": 0,
"url_statementlink": "https:\/\/dev1.chargeover.test\/r\/statement\/view\/536pxrz7yn3v",
"url_paymethodlink": "https:\/\/dev1.chargeover.test\/r\/paymethod\/i\/536pxrz7yn3v",
"url_self": "https:\/\/dev1.chargeover.test\/admin\/r\/customer\/view\/272",
"admin_name": "Jane Doe",
"admin_email": "support@ChargeOver.com",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "Test Customer",
"ship_block": "",
"bill_block": "United States",
"superuser_name": "Test Customer",
"superuser_first_name": "Test",
"superuser_last_name": "Customer",
"superuser_phone": null,
"superuser_email": null,
"superuser_token": "im9e95ankq2e",
"customer_id": 272,
"parent_customer_id": null,
"invoice_delivery": "email",
"dunning_delivery": "email",
"customer_status_id": 1,
"mrr": 0,
"arr": 0,
"customer_status_name": "Current",
"customer_status_str": "active-current",
"customer_status_state": "a",
"superuser_username": "bEg5i9F0Opnj"
},
"user": {
"user_id": 266,
"external_key": null,
"first_name": "Test",
"middle_name_glob": null,
"last_name": "Customer",
"name_suffix": null,
"title": null,
"email": null,
"token": "im9e95ankq2e",
"phone": null,
"mobile": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"user_type_id": 1,
"write_datetime": "2023-02-23 12:38:38",
"mod_datetime": "2023-02-23 12:38:38",
"name": "Test Customer",
"display_as": "Test Customer",
"url_self": "https:\/\/dev1.chargeover.test\/admin\/r\/contact\/view\/266",
"user_type_name": "Billing",
"username": "bEg5i9F0Opnj",
"password": null,
"customer_id": 272
}
}
The cancelled subscription is un-canceled
This event occurs when a cancelled subscription is switched back to an active status.
Note these fields:
context_str = package
context_id = ...
(this will be thepackage_id
of the package)event = uncancel
The cancelled subscription is un-canceled
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: Ma4hWVUNLx0O5zfmJSjQ3AgwpRdY7qu2
X-Chargeover-Source: example.chargeover.com
{
"package": {
"terms_id": 2,
"class_id": null,
"admin_id": 1,
"currency_id": 1,
"brand_id": 1,
"external_key": null,
"token": "5fgbmvwuz3p6",
"nickname": "",
"paymethod": "inv",
"paycycle": "mon",
"bill_addr1": null,
"bill_addr2": null,
"bill_addr3": null,
"bill_city": null,
"bill_state": null,
"bill_postcode": null,
"bill_country": null,
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"creditcard_id": null,
"ach_id": null,
"tokenized_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"custom_7": null,
"custom_8": null,
"custom_9": null,
"custom_10": null,
"custom_11": null,
"custom_12": null,
"custom_13": null,
"custom_14": null,
"custom_15": null,
"custom_16": null,
"custom_17": null,
"custom_18": null,
"custom_19": null,
"custom_20": null,
"write_datetime": "2023-02-23 12:40:15",
"mod_datetime": "2023-02-23 12:40:15",
"start_datetime": "2023-02-23 12:40:15",
"suspendfrom_datetime": null,
"suspendto_datetime": null,
"cancel_datetime": null,
"holduntil_datetime": "2023-03-31 00:00:00",
"terms_name": "Net 30",
"terms_days": 30,
"currency_symbol": "$",
"currency_iso4217": "USD",
"class_name": "",
"brand_name": "Default",
"amount_collected": 0,
"amount_invoiced": 0,
"amount_due": 0,
"is_overdue": false,
"days_overdue": 0,
"amount_overdue": 0,
"next_invoice_datetime": "2023-03-31 00:00:00",
"cancel_reason": null,
"paycycle_name": "Monthly",
"paymethod_name": "Invoice",
"url_self": "https:\/\/dev1.chargeover.test\/admin\/r\/package\/view\/37",
"package_id": 37,
"customer_id": 272,
"package_status_id": 2,
"mrr": 0,
"arr": 0,
"paymethod_detail": "Invoice",
"package_status_name": "Current",
"package_status_str": "active-current",
"package_status_state": "a",
"line_items": [
{
"item_id": 1,
"tierset_id": 3,
"admin_id": null,
"external_key": null,
"nickname": "",
"descrip": "This is a ChargeOver test item.",
"line_quantity": 1,
"trial_days": 0,
"trial_recurs": 0,
"trial_units": 0,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"custom_7": null,
"custom_8": null,
"custom_9": null,
"custom_10": null,
"custom_11": null,
"custom_12": null,
"custom_13": null,
"custom_14": null,
"custom_15": null,
"custom_16": null,
"custom_17": null,
"custom_18": null,
"custom_19": null,
"custom_20": null,
"subscribe_datetime": "2023-02-23 12:40:15",
"subscribe_prorate_from_datetime": null,
"subscribe_prorate_to_datetime": null,
"cancel_datetime": null,
"expire_datetime": null,
"expire_recurs": null,
"item_name": "My Test Service Plan",
"item_external_key": null,
"item_accounting_sku": null,
"item_token": "259b2eebdb33",
"item_type": "service",
"item_units": "",
"item_is_usage": false,
"line_item_id": 39,
"package_id": 37,
"tierset": {
"tierset_id": 3,
"currency_id": 1,
"setup": 0,
"base": 10.95,
"minimum": 0,
"percent": 0,
"paycycle": "evy",
"pricemodel": "fla",
"write_datetime": "2022-11-11 10:56:11",
"mod_datetime": "2022-11-11 10:56:11",
"currency_symbol": "$",
"currency_iso4217": "USD",
"setup_formatted": "$ 0.00",
"base_formatted": "$ 10.95",
"minimum_formatted": "$ 0.00",
"percent_formatted": "0 %",
"pricemodel_desc": "Flat Pricing (example: $X dollars every billing cycle)",
"tiers": []
}
}
]
},
"customer": {
"superuser_id": 266,
"external_key": null,
"token": "536pxrz7yn3v",
"company": "Test Customer",
"bill_addr1": null,
"bill_addr2": null,
"bill_addr3": null,
"bill_city": null,
"bill_state": null,
"bill_postcode": null,
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"terms_id": 2,
"class_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"custom_7": null,
"custom_8": null,
"custom_9": null,
"custom_10": null,
"custom_11": null,
"custom_12": null,
"custom_13": null,
"custom_14": null,
"custom_15": null,
"custom_16": null,
"custom_17": null,
"custom_18": null,
"custom_19": null,
"custom_20": null,
"admin_id": 1,
"campaign_id": null,
"currency_id": 1,
"language_id": 1,
"brand_id": 1,
"default_paymethod": null,
"default_creditcard_id": null,
"default_ach_id": null,
"tax_ident": "",
"no_taxes": false,
"no_dunning": false,
"no_latefees": false,
"write_datetime": "2023-02-23 12:38:37",
"write_ipaddr": "172.29.0.1",
"mod_datetime": "2023-02-23 12:38:37",
"mod_ipaddr": "172.29.0.1",
"terms_name": "Net 30",
"terms_days": 30,
"class_name": "",
"paid": 0,
"total": 0,
"balance": 0,
"url_statementlink": "https:\/\/dev1.chargeover.test\/r\/statement\/view\/536pxrz7yn3v",
"url_paymethodlink": "https:\/\/dev1.chargeover.test\/r\/paymethod\/i\/536pxrz7yn3v",
"url_self": "https:\/\/dev1.chargeover.test\/admin\/r\/customer\/view\/272",
"admin_name": "Jane Doe",
"admin_email": "support@ChargeOver.com",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "Test Customer",
"ship_block": "",
"bill_block": "United States",
"superuser_name": "Test Customer",
"superuser_first_name": "Test",
"superuser_last_name": "Customer",
"superuser_phone": null,
"superuser_email": null,
"superuser_token": "im9e95ankq2e",
"customer_id": 272,
"parent_customer_id": null,
"invoice_delivery": "email",
"dunning_delivery": "email",
"customer_status_id": 1,
"mrr": 0,
"arr": 0,
"customer_status_name": "Current",
"customer_status_str": "active-current",
"customer_status_state": "a",
"superuser_username": "bEg5i9F0Opnj"
},
"user": {
"user_id": 266,
"external_key": null,
"first_name": "Test",
"middle_name_glob": null,
"last_name": "Customer",
"name_suffix": null,
"title": null,
"email": null,
"token": "im9e95ankq2e",
"phone": null,
"mobile": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"user_type_id": 1,
"write_datetime": "2023-02-23 12:38:38",
"mod_datetime": "2023-02-23 12:38:38",
"name": "Test Customer",
"display_as": "Test Customer",
"url_self": "https:\/\/dev1.chargeover.test\/admin\/r\/contact\/view\/266",
"user_type_name": "Billing",
"username": "bEg5i9F0Opnj",
"password": null,
"customer_id": 272
}
}
Items (Products, Discounts)
An item is created
This event occurs when an item is created.
Note these fields:
context_str = item
context_id = ...
(this will be theitem_id
of the item)event = insert
An item is created
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: QxA8p9EtRa30vwfW6BicjIOHXoyUD7Te
X-Chargeover-Source: example.chargeover.com
{
"context_str": "item",
"context_id": "346",
"event": "insert",
"data": {
"item": {
"item_id": 346,
"item_type": "service",
"tierset_id": null,
"name": "Test Product",
"description": null,
"units": null,
"enabled": true,
"accounting_sku": null,
"external_key": null,
"token": "zi9y726b14a5",
"custom_1": null,
"custom_2": null,
"custom_3": null,
"write_datetime": "2019-08-13 07:48:10",
"mod_datetime": "2019-08-13 07:48:10",
"units_plural": "",
"expire_recurs": null,
"trial_recurs": null,
"tiersets": [
{
"tierset_id": 617,
"currency_id": 1,
"setup": 0,
"base": 195.95,
"minimum": 0,
"percent": 0,
"paycycle": "evy",
"pricemodel": "fla",
"write_datetime": "2019-08-13 07:48:10",
"mod_datetime": "2019-08-13 07:48:10",
"currency_symbol": "$",
"currency_iso4217": "USD",
"setup_formatted": "$ 0.00",
"base_formatted": "$ 195.95",
"minimum_formatted": "$ 0.00",
"percent_formatted": "0 %",
"pricemodel_desc": "Flat Pricing (example: $X dollars every billing cycle)",
"tiers": []
},
{
"tierset_id": 618,
"currency_id": 2,
"setup": 0,
"base": 195.95,
"minimum": 0,
"percent": 0,
"paycycle": "evy",
"pricemodel": "fla",
"write_datetime": "2019-08-13 07:48:10",
"mod_datetime": "2019-08-13 07:48:10",
"currency_symbol": "CAD$",
"currency_iso4217": "CAD",
"setup_formatted": "CAD$ 0.00",
"base_formatted": "CAD$ 195.95",
"minimum_formatted": "CAD$ 0.00",
"percent_formatted": "0 %",
"pricemodel_desc": "Flat Pricing (example: $X dollars every billing cycle)",
"tiers": []
},
{
"tierset_id": 619,
"currency_id": 6,
"setup": 0,
"base": 195.95,
"minimum": 0,
"percent": 0,
"paycycle": "evy",
"pricemodel": "fla",
"write_datetime": "2019-08-13 07:48:10",
"mod_datetime": "2019-08-13 07:48:10",
"currency_symbol": "A$",
"currency_iso4217": "AUD",
"setup_formatted": "A$ 0.00",
"base_formatted": "A$ 195.95",
"minimum_formatted": "A$ 0.00",
"percent_formatted": "0 %",
"pricemodel_desc": "Flat Pricing (example: $X dollars every billing cycle)",
"tiers": []
}
]
}
},
"security_token": "QxA8p9EtRa30vwfW6BicjIOHXoyUD7Te"
}
An item is updated
This event occurs when an item is updated.
Note these fields:
context_str = item
context_id = ...
(this will be theitem_id
of the item)event = update
An item is updated
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: QxA8p9EtRa30vwfW6BicjIOHXoyUD7Te
X-Chargeover-Source: example.chargeover.com
{
"context_str": "item",
"context_id": "346",
"event": "update",
"data": {
"item": {
"item_id": 346,
"item_type": "service",
"tierset_id": 619,
"name": "Test Product",
"description": "",
"units": "",
"enabled": true,
"accounting_sku": "",
"external_key": null,
"token": "zi9y726b14a5",
"custom_1": null,
"custom_2": null,
"custom_3": null,
"write_datetime": "2019-08-13 07:48:10",
"mod_datetime": "2019-08-13 07:52:51",
"units_plural": "",
"expire_recurs": null,
"trial_recurs": "0",
"tiersets": [
{
"tierset_id": 620,
"currency_id": 1,
"setup": 0,
"base": 195.95,
"minimum": 0,
"percent": 0,
"paycycle": "evy",
"pricemodel": "fla",
"write_datetime": "2019-08-13 07:52:51",
"mod_datetime": "2019-08-13 07:52:51",
"currency_symbol": "$",
"currency_iso4217": "USD",
"setup_formatted": "$ 0.00",
"base_formatted": "$ 195.95",
"minimum_formatted": "$ 0.00",
"percent_formatted": "0 %",
"pricemodel_desc": "Flat Pricing (example: $X dollars every billing cycle)",
"tiers": []
},
{
"tierset_id": 621,
"currency_id": 2,
"setup": 0,
"base": 195.95,
"minimum": 0,
"percent": 0,
"paycycle": "evy",
"pricemodel": "fla",
"write_datetime": "2019-08-13 07:52:51",
"mod_datetime": "2019-08-13 07:52:51",
"currency_symbol": "CAD$",
"currency_iso4217": "CAD",
"setup_formatted": "CAD$ 0.00",
"base_formatted": "CAD$ 195.95",
"minimum_formatted": "CAD$ 0.00",
"percent_formatted": "0 %",
"pricemodel_desc": "Flat Pricing (example: $X dollars every billing cycle)",
"tiers": []
},
{
"tierset_id": 622,
"currency_id": 6,
"setup": 0,
"base": 195.95,
"minimum": 0,
"percent": 0,
"paycycle": "evy",
"pricemodel": "fla",
"write_datetime": "2019-08-13 07:52:51",
"mod_datetime": "2019-08-13 07:52:51",
"currency_symbol": "A$",
"currency_iso4217": "AUD",
"setup_formatted": "A$ 0.00",
"base_formatted": "A$ 195.95",
"minimum_formatted": "A$ 0.00",
"percent_formatted": "0 %",
"pricemodel_desc": "Flat Pricing (example: $X dollars every billing cycle)",
"tiers": []
}
]
}
},
"security_token": "QxA8p9EtRa30vwfW6BicjIOHXoyUD7Te"
}
Transactions (Payments, Refunds, Credits)
A transaction status has changed
This event occurs when a transaction changes status (for example, when a transaction is voided).
Note these fields:
context_str = transaction
context_id = ...
(this will be thetransaction_id
value of the transaction that changed)event = status
A transaction status has changed
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: g6VkmXzSGb42PLodDCTqeiJ5Ol3n7frF
X-Chargeover-Source: example.chargeover.com
{
"context_str": "transaction",
"context_id": "39",
"event": "status",
"data": {
"transaction": {
"transaction_id": 39,
"gateway_id": 204,
"currency_id": 1,
"external_key": null,
"token": "p70t78m16u0v",
"transaction_date": "2016-04-24",
"gateway_status": 1,
"gateway_transid": "12345",
"gateway_msg": "",
"amount": 50,
"fee": 0,
"transaction_type": "pay",
"transaction_method": "Paid by Check",
"transaction_detail": "12345",
"transaction_datetime": "2016-04-24 15:53:04",
"transaction_ipaddr": "172.16.16.140",
"void_datetime": "2016-04-26 02:00:00",
"transaction_type_name": "Payment",
"currency_symbol": "$",
"currency_iso4217": "USD",
"customer_id": 17
},
"customer": {
"superuser_id": 363,
"external_key": null,
"token": "vdo15953f650",
"company": "Test new rate",
"bill_addr1": "56 Cowles Road",
"bill_addr2": "",
"bill_addr3": "",
"bill_city": "San Fran",
"bill_state": "CA",
"bill_postcode": "90213",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": "",
"ship_addr2": "",
"ship_addr3": "",
"ship_city": "",
"ship_state": "",
"ship_postcode": "",
"ship_country": "",
"ship_notes": null,
"terms_id": 2,
"class_id": null,
"custom_1": "",
"custom_2": null,
"custom_3": null,
"admin_id": 4,
"campaign_id": null,
"currency_id": 1,
"language_id": 0,
"brand_id": 1,
"no_taxes": false,
"no_dunning": false,
"write_datetime": "2016-04-22 16:10:25",
"write_ipaddr": "172.16.16.140",
"mod_datetime": "2016-04-22 16:11:03",
"mod_ipaddr": "172.16.16.140",
"terms_name": "Net 30",
"terms_days": 30,
"paid": 0,
"total": 34.83,
"balance": 34.83,
"url_paymethodlink": "http:\/\/dev.chargeover.com\/r\/paymethod\/i\/vdo15953f650",
"admin_name": "Keith Palmer",
"admin_email": "keith@ChargeOver.com",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "Test new rate",
"ship_block": "",
"bill_block": "56 Cowles Road\nSan Fran CA 90213\nUnited States",
"superuser_name": "admin",
"superuser_first_name": "admin",
"superuser_last_name": "",
"superuser_phone": "",
"superuser_email": "",
"superuser_token": "0118fey185pl",
"customer_id": 17,
"customer_status_id": 1,
"customer_status_name": "Current",
"customer_status_str": "active-current",
"customer_status_state": "a",
"superuser_username": "0IHCUYAks7a4"
},
"user": {
"user_id": 363,
"external_key": null,
"first_name": "admin",
"middle_name_glob": null,
"last_name": "",
"name_suffix": null,
"title": "",
"email": "",
"token": "0118fey185pl",
"phone": "",
"user_type_id": 1,
"write_datetime": "2016-04-22 16:10:25",
"mod_datetime": "2016-04-22 16:10:25",
"name": "admin",
"display_as": "admin",
"user_type_name": "Billing",
"username": "0IHCUYAks7a4"
}
},
"security_token": "g6VkmXzSGb42PLodDCTqeiJ5Ol3n7frF"
}
A transaction is created
This event occurs when a transaction is created.
Note that this event will occur for both successful and failed transactions. You can check the status of the transaction by
examining the gateway_status
attribute of the transaction object.
Note these fields:
context_str = transaction
context_id = ...
(this will be thetransaction_id
of the transaction)event = insert
A transaction is created
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: 9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo
X-Chargeover-Source: example.chargeover.com
{
"context_str": "transaction",
"context_id": "7",
"event": "insert",
"data": {
"transaction": {
"transaction_id": 7,
"gateway_id": 201,
"currency_id": 1,
"external_key": null,
"token": "k4ufy9i769vt",
"transaction_date": "2017-06-08",
"gateway_status": 1,
"gateway_transid": "*CHARGE: Test Credit Card* [1496950958]",
"gateway_msg": "",
"gateway_err_code": 0,
"gateway_err_detail": null,
"gateway_method": "visa",
"amount": 60.95,
"fee": 0,
"transaction_type": "pay",
"transaction_method": "Visa",
"transaction_detail": "x4444",
"transaction_datetime": "2017-06-08 15:42:38",
"transaction_ipaddr": "127.0.0.1",
"void_datetime": null,
"transaction_status_name": "Success",
"transaction_status_str": "ok-successful",
"transaction_status_state": "o",
"transaction_type_name": "Payment",
"applied": 60.95,
"currency_symbol": "$",
"currency_iso4217": "USD",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/transaction\/view\/7",
"customer_id": 8,
"unapplied": 0,
"applied_to": [
{
"invoice_id": 5007,
"applied": 10.95
},
{
"invoice_id": 5008,
"applied": 50
}
]
},
"customer": {
"superuser_id": 354,
"external_key": null,
"token": "2ygprd9569t4",
"company": "John Doe's Company, LLC",
"bill_addr1": "34 Address Street",
"bill_addr2": null,
"bill_addr3": null,
"bill_city": "City",
"bill_state": "State",
"bill_postcode": null,
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"terms_id": 2,
"class_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"admin_id": 3,
"campaign_id": null,
"currency_id": 1,
"language_id": 1,
"brand_id": 1,
"no_taxes": false,
"no_dunning": false,
"write_datetime": "2017-06-08 15:39:59",
"write_ipaddr": "127.0.0.1",
"mod_datetime": "2017-06-08 15:39:59",
"mod_ipaddr": "127.0.0.1",
"terms_name": "Net 30",
"terms_days": 30,
"paid": 60.95,
"total": 60.95,
"balance": 0,
"url_paymethodlink": "http:\/\/dev.chargeover.com\/r\/paymethod\/i\/2ygprd9569t4",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/customer\/view\/8",
"admin_name": "Karli Palmer",
"admin_email": "karli@chargeover.com",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "John Doe's Company, LLC",
"ship_block": "",
"bill_block": "34 Address Street\nCity State\nUnited States",
"superuser_name": "John Doe",
"superuser_first_name": "John",
"superuser_last_name": "Doe",
"superuser_phone": "",
"superuser_email": "john@example.com",
"superuser_token": "4e7t9r5a03fv",
"customer_id": 8,
"invoice_delivery": "email",
"dunning_delivery": "email",
"customer_status_id": 1,
"customer_status_name": "Current",
"customer_status_str": "active-current",
"customer_status_state": "a",
"superuser_username": "john@example.com"
},
"user": {
"user_id": 354,
"external_key": null,
"first_name": "John",
"middle_name_glob": null,
"last_name": "Doe",
"name_suffix": null,
"title": "",
"email": "john@example.com",
"token": "4e7t9r5a03fv",
"phone": "",
"user_type_id": 1,
"write_datetime": "2017-06-08 15:39:59",
"mod_datetime": "2017-06-08 15:39:59",
"name": "John Doe",
"display_as": "John Doe",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/contact\/view\/354",
"user_type_name": "Billing",
"username": "john@example.com",
"customer_id": 8
}
},
"security_token": "9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo"
}
A transaction is applied to an invoice
This event occurs when a transaction is applied to an invoice.
Note these fields:
context_str = transaction
context_id = ...
(this will be thetransaction_id
of the transaction)event = apply
A transaction is applied to an invoice
A transaction is marked void
This event occurs when a transaction is marked void.
Note these fields:
context_str = transaction
context_id = ...
(this will be thetransaction_id
of the transaction)event = void
A transaction is marked void
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: 9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo
X-Chargeover-Source: example.chargeover.com
{
"context_str": "transaction",
"context_id": "7",
"event": "void",
"data": {
"transaction": {
"transaction_id": 7,
"gateway_id": 201,
"currency_id": 1,
"external_key": null,
"token": "k4ufy9i769vt",
"transaction_date": "2017-06-08",
"gateway_status": 1,
"gateway_transid": "*CHARGE: Test Credit Card* [1496950958]",
"gateway_msg": "",
"gateway_err_code": 0,
"gateway_err_detail": null,
"gateway_method": "visa",
"amount": 60.95,
"fee": 0,
"transaction_type": "pay",
"transaction_method": "Visa",
"transaction_detail": "x4444",
"transaction_datetime": "2017-06-08 15:42:38",
"transaction_ipaddr": "127.0.0.1",
"void_datetime": null,
"transaction_status_name": "Success",
"transaction_status_str": "ok-successful",
"transaction_status_state": "o",
"transaction_type_name": "Payment",
"applied": 60.95,
"currency_symbol": "$",
"currency_iso4217": "USD",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/transaction\/view\/7",
"customer_id": 8,
"unapplied": 0,
"applied_to": [
{
"invoice_id": 5007,
"applied": 10.95
},
{
"invoice_id": 5008,
"applied": 50
}
]
},
"customer": {
"superuser_id": 354,
"external_key": null,
"token": "2ygprd9569t4",
"company": "John Doe's Company, LLC",
"bill_addr1": "34 Doe Street",
"bill_addr2": "",
"bill_addr3": "",
"bill_city": "City",
"bill_state": "New State",
"bill_postcode": "",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"terms_id": 3,
"class_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"admin_id": 3,
"campaign_id": null,
"currency_id": 1,
"language_id": 1,
"brand_id": 1,
"no_taxes": false,
"no_dunning": false,
"write_datetime": "2017-06-08 15:39:59",
"write_ipaddr": "127.0.0.1",
"mod_datetime": "2017-06-13 12:40:51",
"mod_ipaddr": "127.0.0.1",
"terms_name": "Due on Receipt",
"terms_days": 0,
"paid": 10.95,
"total": 71.9,
"balance": 60.95,
"url_paymethodlink": "http:\/\/dev.chargeover.com\/r\/paymethod\/i\/2ygprd9569t4",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/customer\/view\/8",
"admin_name": "Karli Palmer",
"admin_email": "karli@chargeover.com",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "John Doe's Company, LLC",
"ship_block": "",
"bill_block": "34 Doe Street\nCity New State\nUnited States",
"superuser_name": "John Doe",
"superuser_first_name": "John",
"superuser_last_name": "Doe",
"superuser_phone": "",
"superuser_email": "john@example.com",
"superuser_token": "4e7t9r5a03fv",
"customer_id": 8,
"invoice_delivery": "email",
"dunning_delivery": "email",
"customer_status_id": 1,
"customer_status_name": "Current",
"customer_status_str": "active-current",
"customer_status_state": "a",
"superuser_username": "john@example.com"
},
"user": {
"user_id": 354,
"external_key": null,
"first_name": "John",
"middle_name_glob": null,
"last_name": "Doe",
"name_suffix": null,
"title": "",
"email": "john@example.com",
"token": "4e7t9r5a03fv",
"phone": "",
"user_type_id": 1,
"write_datetime": "2017-06-08 15:39:59",
"mod_datetime": "2017-06-08 15:39:59",
"name": "John Doe",
"display_as": "John Doe",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/contact\/view\/354",
"user_type_name": "Billing",
"username": "john@example.com",
"customer_id": 8
}
},
"security_token": "9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo"
}
ACH/eChecks
Automated Clearing House (ACH)/eChecks
An ACH/eCheck account created
This event occurs when an ACH payment method is created.
Note these fields:
context_str = ach
-
context_id = ...
(this will be theach_id
of the new ACH paymethod) event = insert
An ACH/eCheck account created
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: Jy5gsouFvDh3ZwS241xpHbPt0K7MkcTW
X-Chargeover-Source: example.chargeover.com
{
"context_str": "ach",
"context_id": 6,
"event": "insert",
"data": {
"ach": {
"ach_id": 6,
"external_key": null,
"type": "chec",
"token": "b9tiqm03s4x5",
"type_name": "Checking",
"customer_id": 1,
"name": "Jon Doe",
"mask_bank": "xxxxxxxxxxxxxxxxxxxxLOAN",
"mask_number": "xx3456",
"mask_routing": "xxxxx6789"
}
},
"security_token": "Jy5gsouFvDh3ZwS241xpHbPt0K7MkcTW"
}
ACH/eCheck account updated
This event occurs when an ACH payment method is updated.
Note these fields:
context_str = ach
-
context_id = ...
(this is theach_id
of the ACH paymethod) event = update
ACH/eCheck account updated
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: Jy5gsouFvDh3ZwS241xpHbPt0K7MkcTW
X-Chargeover-Source: example.chargeover.com
{
"context_str": "ach",
"context_id": 6,
"event": "update",
"data": {
"ach": {
"ach_id": 6,
"external_key": null,
"type": "chec",
"token": "b9tiqm03s4x5",
"type_name": "Checking",
"customer_id": 1,
"name": "Jon Doe",
"mask_bank": "xxxxxxxxxxxxxxxxxxxxLOAN",
"mask_number": "xx3456",
"mask_routing": "xxxxx6789"
}
},
"security_token": "Jy5gsouFvDh3ZwS241xpHbPt0K7MkcTW"
}
ACH/eCheck account deleted
This event occurs when an ACH payment method is deleted.
Note these fields:
context_str = ach
-
context_id = ...
(this was theach_id
of the deleted ACH paymethod) event = delete
ACH/eCheck account deleted
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: Jy5gsouFvDh3ZwS241xpHbPt0K7MkcTW
X-Chargeover-Source: example.chargeover.com
{
"context_str": "ach",
"context_id": "6",
"event": "delete",
"data": {
"ach": {
"ach_id": 6,
"external_key": null,
"type": "chec",
"token": "b9tiqm03s4x5",
"type_name": "Checking",
"customer_id": 1,
"name": "Jon Doe",
"mask_bank": "xxxxxxxxxxxxxxxxxxxxLOAN",
"mask_number": "xx3456",
"mask_routing": "xxxxx6789"
}
},
"security_token": "Jy5gsouFvDh3ZwS241xpHbPt0K7MkcTW"
}
ACH/eCheck account approved for autopay
This event occurs when an ACH payment method is opted into autopay.
Note these fields:
context_str = ach
-
context_id = ...
(this will be theach_id
of the ACH account that has been approved for autopay) event = autopay
ACH/eCheck account approved for autopay
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: 9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo
X-Chargeover-Source: example.chargeover.com
{
"context_str": "ach",
"context_id": "12",
"event": "autopay",
"data": {
"ach": {
"ach_id": 12,
"external_key": null,
"type": "chec",
"token": "5191s5l4di8j",
"type_name": "Checking",
"customer_id": 39,
"name": "Marissa Lexington",
"mask_bank": "xBANK",
"mask_number": "x6667",
"mask_routing": "x3004"
},
"customer": {
"superuser_id": 388,
"external_key": null,
"token": "wm0tg96n446e",
"company": "Knittin' Kittens Yarn Co.",
"bill_addr1": "56 Highland Street",
"bill_addr2": "",
"bill_addr3": "",
"bill_city": "Coventry",
"bill_state": "CT",
"bill_postcode": "06238",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"terms_id": 2,
"class_id": 0,
"custom_1": "",
"custom_2": "",
"custom_3": "",
"custom_4": null,
"custom_5": null,
"custom_6": null,
"admin_id": null,
"campaign_id": null,
"currency_id": 1,
"language_id": 1,
"brand_id": 1,
"default_paymethod": null,
"default_creditcard_id": null,
"default_ach_id": null,
"tax_ident": "",
"no_taxes": false,
"no_dunning": false,
"write_datetime": "2018-03-01 12:24:55",
"write_ipaddr": "127.0.0.1",
"mod_datetime": "2018-03-01 12:28:16",
"mod_ipaddr": "127.0.0.1",
"terms_name": "Net 30",
"terms_days": 30,
"class_name": "",
"paid": 150,
"total": 150,
"balance": 0,
"url_statementlink": "http:\/\/dev.chargeover.com\/r\/statement\/view\/wm0tg96n446e",
"url_paymethodlink": "http:\/\/dev.chargeover.com\/r\/paymethod\/i\/wm0tg96n446e",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/customer\/view\/39",
"admin_name": "",
"admin_email": "",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "Knittin' Kittens Yarn Co.",
"ship_block": "",
"bill_block": "56 Highland Street\nCoventry CT 06238\nUnited States",
"superuser_name": "Marissa Lexington",
"superuser_first_name": "Marissa",
"superuser_last_name": "Lexington",
"superuser_phone": "222-333-1111",
"superuser_email": "marissa@example.com",
"superuser_token": "28x81ky92vow",
"customer_id": 39,
"parent_customer_id": null,
"invoice_delivery": "email",
"dunning_delivery": "email",
"customer_status_id": 1,
"customer_status_name": "Current",
"customer_status_str": "active-current",
"customer_status_state": "a",
"superuser_username": "marissa@example.com"
},
"user": {
"user_id": 388,
"external_key": null,
"first_name": "Marissa",
"middle_name_glob": null,
"last_name": "Lexington",
"name_suffix": null,
"title": null,
"email": "marissa@example.com",
"token": "28x81ky92vow",
"phone": "222-333-1111",
"user_type_id": 1,
"write_datetime": "2018-03-01 12:24:55",
"mod_datetime": "2018-03-01 12:24:55",
"name": "Marissa Lexington",
"display_as": "Marissa Lexington",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/contact\/view\/388",
"user_type_name": "Billing",
"username": "marissa@example.com",
"customer_id": 39
}
},
"security_token": "9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo"
}
Credit Cards
Credit Cards
Credit card created
This event occurs when a new credit card is added.
Note these fields:
context_str = creditcard
-
context_id = ...
(this will be thecreditcard_id
of the new credit card) event = insert
Credit card created
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: Jy5gsouFvDh3ZwS241xpHbPt0K7MkcTW
X-Chargeover-Source: example.chargeover.com
{
"context_str": "creditcard",
"context_id": 55,
"event": "insert",
"data": {
"creditcard": {
"creditcard_id": 55,
"external_key": null,
"type": "visa",
"token": "3nvojm1967wi",
"expdate": "2016-07-01",
"write_datetime": "2015-03-13 21:07:59",
"write_ipaddr": "::1",
"mask_number": "xxxx-xxxx-xxxx-1111",
"name": "Jon Doe",
"expdate_month": "7",
"expdate_year": "2016",
"expdate_formatted": "Jul 2016",
"type_name": "Visa",
"customer_id": 1
}
},
"security_token": "Jy5gsouFvDh3ZwS241xpHbPt0K7MkcTW"
}
Credt card updated
This event occurs when a credit card is updated.
Note these fields:
context_str = creditcard
-
context_id = ...
(this is thecreditcard_id
of the credit card) event = update
Credt card updated
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: Jy5gsouFvDh3ZwS241xpHbPt0K7MkcTW
X-Chargeover-Source: example.chargeover.com
{
"context_str": "creditcard",
"context_id": 55,
"event": "update",
"data": {
"creditcard": {
"creditcard_id": 55,
"external_key": null,
"type": "visa",
"token": "3nvojm1967wi",
"expdate": "2016-07-01",
"write_datetime": "2015-03-13 21:07:59",
"write_ipaddr": "::1",
"mask_number": "xxxx-xxxx-xxxx-1111",
"name": "Jon Doe",
"expdate_month": "7",
"expdate_year": "2016",
"expdate_formatted": "Jul 2016",
"type_name": "Visa",
"customer_id": 1
}
},
"security_token": "Jy5gsouFvDh3ZwS241xpHbPt0K7MkcTW"
}
Credit card deleted
This event occurs when a credit card is deleted.
Note these fields:
context_str = creditcard
-
context_id = ...
(this is thecreditcard_id
of the deleted credit card) event = delete
Credit card deleted
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: Jy5gsouFvDh3ZwS241xpHbPt0K7MkcTW
X-Chargeover-Source: example.chargeover.com
{
"context_str": "creditcard",
"context_id": "55",
"event": "delete",
"data": {
"creditcard": {
"creditcard_id": 55,
"external_key": null,
"type": "visa",
"token": "3nvojm1967wi",
"expdate": "2016-07-01",
"write_datetime": "2015-03-13 21:07:59",
"write_ipaddr": "::1",
"mask_number": "xxxx-xxxx-xxxx-1111",
"name": "Jon Doe",
"expdate_month": "7",
"expdate_year": "2016",
"expdate_formatted": "Jul 2016",
"type_name": "Visa",
"customer_id": 1
}
},
"security_token": "Jy5gsouFvDh3ZwS241xpHbPt0K7MkcTW"
}
Credit card is expiring soon
This event occurs when an in-use credit card is expiring soon.
Note these fields:
context_str = creditcard
-
context_id = ...
(this is thecreditcard_id
of the credit card) event = expiring
Credit card is expiring soon
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: 0xeohlHKEOJXAdbDQS5z8Zyafq7kGUR3
X-Chargeover-Source: example.chargeover.com
{
"context_str": "creditcard",
"context_id": "110",
"event": "expiring",
"data": {
"creditcard": {
"creditcard_id": 110,
"external_key": null,
"type": "visa",
"token": "646259fydgq1",
"expdate": "2016-09-01",
"write_datetime": "2016-09-07 09:17:24",
"write_ipaddr": "172.16.16.140",
"mask_number": "x1111",
"name": "",
"expdate_month": "9",
"expdate_year": "2016",
"expdate_formatted": "Sep 2016",
"type_name": "Visa",
"url_updatelink": "http:\/\/dev.chargeover.com\/r\/paymethod\/i\/690gx7105dik",
"address": "",
"city": null,
"postcode": "",
"country": null,
"customer_id": 122
},
"customer": {
"superuser_id": 462,
"external_key": null,
"token": "690gx7105dik",
"company": "Test Stripe YEN",
"bill_addr1": null,
"bill_addr2": null,
"bill_addr3": null,
"bill_city": null,
"bill_state": null,
"bill_postcode": null,
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"terms_id": 2,
"class_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"admin_id": 3,
"campaign_id": null,
"currency_id": 75,
"language_id": 1,
"brand_id": 1,
"no_taxes": false,
"no_dunning": false,
"write_datetime": "2016-09-07 09:15:01",
"write_ipaddr": "172.16.16.140",
"mod_datetime": "2016-09-07 09:15:01",
"mod_ipaddr": "172.16.16.140",
"terms_name": "Net 30",
"terms_days": 30,
"paid": 2000,
"total": 0,
"balance": -2000,
"url_paymethodlink": "http:\/\/dev.chargeover.com\/r\/paymethod\/i\/690gx7105dik",
"admin_name": "Keith Palmer",
"admin_email": "keith@chargeover.com",
"currency_symbol": "\u00a5",
"currency_iso4217": "JPY",
"display_as": "Test Stripe YEN",
"ship_block": "",
"bill_block": "United States",
"superuser_name": "admin",
"superuser_first_name": "admin",
"superuser_last_name": "",
"superuser_phone": "",
"superuser_email": "",
"superuser_token": "n5jc72265yw5",
"customer_id": 122,
"invoice_delivery": "print",
"dunning_delivery": "email",
"customer_status_id": 1,
"customer_status_name": "Current",
"customer_status_str": "active-current",
"customer_status_state": "a",
"superuser_username": "kIcMmVG0zDLw"
},
"user": {
"user_id": 462,
"external_key": null,
"first_name": "admin",
"middle_name_glob": null,
"last_name": "",
"name_suffix": null,
"title": "",
"email": "",
"token": "n5jc72265yw5",
"phone": "",
"user_type_id": 1,
"write_datetime": "2016-09-07 09:15:01",
"mod_datetime": "2016-09-07 09:15:01",
"name": "admin",
"display_as": "admin",
"user_type_name": "Billing",
"username": "kIcMmVG0zDLw"
}
},
"security_token": "0xeohlHKEOJXAdbDQS5z8Zyafq7kGUR3"
}
Credit card has expired
This event occurs when an in-use credit card has expired.
Note these fields:
context_str = creditcard
-
context_id = ...
(this is thecreditcard_id
of the credit card) event = expired
Credit card has expired
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: gqCW7zYaGVsOhBlS3erTxnNm0fLIHMbK
X-Chargeover-Source: example.chargeover.com
{
"context_str": "creditcard",
"context_id": 39,
"event": "expired",
"data": {
"creditcard": {
"creditcard_id": 332,
"external_key": null,
"type": "visa",
"token": "rue045gsphaa",
"expdate": "2023-02-01",
"write_datetime": "2023-03-09 13:28:44",
"write_ipaddr": "172.29.0.1",
"mask_number": "x1111",
"mask_and_bin_number": "411111xxx1111",
"name": "ChargeOver",
"expdate_month": "2",
"expdate_year": "2023",
"expdate_formatted": "Feb 2023",
"type_name": "Visa",
"url_updatelink": "https:\/\/dev1.chargeover.com\/r\/paymethod\/i\/fabqea1zjh5g",
"address": null,
"city": null,
"postcode": null,
"country": "United States",
"customer_id": 919
},
"customer": {
"superuser_id": 350,
"external_key": null,
"token": "fabqea1zjh5g",
"company": "ChargeOver",
"bill_addr1": null,
"bill_addr2": null,
"bill_addr3": null,
"bill_city": null,
"bill_state": null,
"bill_postcode": null,
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": "United States",
"ship_notes": null,
"terms_id": 2,
"class_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"custom_7": null,
"custom_8": null,
"custom_9": null,
"custom_10": null,
"custom_11": null,
"custom_12": null,
"custom_13": null,
"custom_14": null,
"custom_15": null,
"custom_16": null,
"custom_17": null,
"custom_18": null,
"custom_19": null,
"custom_20": null,
"admin_id": null,
"campaign_id": null,
"currency_id": 1,
"language_id": 1,
"brand_id": 1,
"default_paymethod": null,
"default_creditcard_id": null,
"default_ach_id": null,
"tax_ident": "",
"no_taxes": false,
"no_dunning": false,
"no_latefees": false,
"write_datetime": "2023-03-09 13:28:15",
"write_ipaddr": "172.29.0.1",
"mod_datetime": "2023-03-09 13:28:15",
"mod_ipaddr": "172.29.0.1",
"terms_name": "Net 30",
"terms_days": 30,
"class_name": "",
"paid": 0,
"total": 0,
"balance": 0,
"url_statementlink": "https:\/\/dev1.chargeover.com\/r\/statement\/view\/fabqea1zjh5g",
"url_paymethodlink": "https:\/\/dev1.chargeover.com\/r\/paymethod\/i\/fabqea1zjh5g",
"url_self": "https:\/\/dev1.chargeover.com\/admin\/r\/customer\/view\/919",
"admin_name": "",
"admin_email": "",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "ChargeOver",
"ship_block": "United States",
"bill_block": "United States",
"superuser_name": "ChargeOver",
"superuser_first_name": "ChargeOver",
"superuser_last_name": null,
"superuser_phone": null,
"superuser_email": null,
"superuser_token": "uw7eyhnsbz96",
"customer_id": 919,
"parent_customer_id": null,
"invoice_delivery": "email",
"dunning_delivery": "email",
"customer_status_id": 1,
"mrr": 0,
"arr": 0,
"customer_status_name": "Current",
"customer_status_str": "active-current",
"customer_status_state": "a",
"superuser_username": "jkzKvJaqFLU4"
},
"user": {
"user_id": 350,
"external_key": null,
"first_name": "ChargeOver",
"middle_name_glob": null,
"last_name": null,
"name_suffix": null,
"title": null,
"email": null,
"token": "uw7eyhnsbz96",
"phone": null,
"mobile": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"user_type_id": 1,
"write_datetime": "2023-03-09 13:28:18",
"mod_datetime": "2023-03-09 13:28:18",
"name": "ChargeOver",
"display_as": "ChargeOver",
"url_self": "https:\/\/dev1.chargeover.com\/admin\/r\/contact\/view\/350",
"user_type_name": "Billing",
"username": "jkzKvJaqFLU4",
"password": null,
"customer_id": 919
}
},
"security_token": "gqCW7zYaGVsOhBlS3erTxnNm0fLIHMbK"
}
Credit card has been approved for autopay
This event occurs when a creditcard has been opted into autopay.
Note these fields:
context_str = creditcard
-
context_id = ...
(this will be thecreditcard_id
of the credit card that has been approved for autopay) event = autopay
Credit card has been approved for autopay
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: 9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo
X-Chargeover-Source: example.chargeover.com
{
"context_str": "creditcard",
"context_id": "18",
"event": "autopay",
"data": {
"creditcard": {
"creditcard_id": 18,
"external_key": null,
"type": "amex",
"token": "9t8y04h3725r",
"expdate": "2019-03-01",
"write_datetime": "2018-03-01 12:03:05",
"write_ipaddr": "127.0.0.1",
"mask_number": "x0005",
"mask_and_bin_number": "378282xxx0005",
"name": "",
"expdate_month": "3",
"expdate_year": "2019",
"expdate_formatted": "Mar 2019",
"type_name": "American Express",
"url_updatelink": "http:\/\/dev.chargeover.com\/r\/paymethod\/i\/46661z16tliu",
"address": null,
"city": null,
"postcode": null,
"country": "United States",
"customer_id": 37
},
"customer": {
"superuser_id": 386,
"external_key": null,
"token": "46661z16tliu",
"company": "Connecticut Flooring Company",
"bill_addr1": "74 Cowles Avenue",
"bill_addr2": "",
"bill_addr3": "",
"bill_city": "Willington",
"bill_state": "CT",
"bill_postcode": "06279",
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"terms_id": 2,
"class_id": 0,
"custom_1": "",
"custom_2": "",
"custom_3": "",
"custom_4": null,
"custom_5": null,
"custom_6": null,
"admin_id": 4,
"campaign_id": null,
"currency_id": 1,
"language_id": 1,
"brand_id": 1,
"default_paymethod": "crd",
"default_creditcard_id": 18,
"default_ach_id": null,
"tax_ident": "",
"no_taxes": false,
"no_dunning": false,
"write_datetime": "2018-02-22 10:52:51",
"write_ipaddr": "127.0.0.1",
"mod_datetime": "2018-03-01 11:56:38",
"mod_ipaddr": "127.0.0.1",
"terms_name": "Net 30",
"terms_days": 30,
"class_name": "",
"paid": 1210,
"total": 1210,
"balance": 0,
"url_statementlink": "http:\/\/dev.chargeover.com\/r\/statement\/view\/46661z16tliu",
"url_paymethodlink": "http:\/\/dev.chargeover.com\/r\/paymethod\/i\/46661z16tliu",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/customer\/view\/37",
"admin_name": "Dave Tyson",
"admin_email": "dave@example.com",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "Connecticut Flooring Company",
"ship_block": "",
"bill_block": "74 Cowles Avenue\nWillington CT 06279\nUnited States",
"superuser_name": "Karli Marie",
"superuser_first_name": "Karli",
"superuser_last_name": "Marie",
"superuser_phone": "",
"superuser_email": "karli@example.com",
"superuser_token": "gdz737x5ye81",
"customer_id": 37,
"parent_customer_id": null,
"invoice_delivery": "email",
"dunning_delivery": "email",
"customer_status_id": 1,
"customer_status_name": "Current",
"customer_status_str": "active-current",
"customer_status_state": "a",
"superuser_username": "dCMntSrhFQB7"
},
"user": {
"user_id": 386,
"external_key": null,
"first_name": "Karli",
"middle_name_glob": null,
"last_name": "Marie",
"name_suffix": null,
"title": "",
"email": "karli@example.com",
"token": "gdz737x5ye81",
"phone": "",
"user_type_id": 1,
"write_datetime": "2018-02-22 10:52:51",
"mod_datetime": "2018-03-01 11:57:09",
"name": "Karli Marie",
"display_as": "Karli Marie",
"url_self": "http:\/\/dev.chargeover.com\/admin\/r\/contact\/view\/386",
"user_type_name": "Billing",
"username": "dCMntSrhFQB7",
"customer_id": 37
}
},
"security_token": "9aCm8BdvtVT3JzA2GKHFu1fMilwIDXRo"
}
Tokenized Payment Methods
Tokenized
A tokenized payment method is created
This event occurs when a tokenized payment method is created.
Note these fields:
context_str = tokenized
context_id = ...
(this will be thetokenized_id
of the tokenized payment method)event = insert
A tokenized payment method is created
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: a8T3OnpczQwvqgfZumVbrsYhBt0DEeS1
X-Chargeover-Source: example.chargeover.com
{
"context_str": "tokenized",
"context_id": 2,
"event": "insert",
"data": {
"tokenized": {
"tokenized_id": 2,
"type": "",
"name": "Tokenized ACH",
"token": "asldiruesknjfis",
"expdate": null,
"type_hint": null,
"paymethod_hint": null,
"write_datetime": "2023-03-23 12:27:12",
"mod_datetime": "2023-03-23 12:27:12",
"customer_id": 307
},
"customer": {
"superuser_id": 295,
"external_key": null,
"token": "d07dup6w8h61",
"company": "Test Customer",
"bill_addr1": null,
"bill_addr2": null,
"bill_addr3": null,
"bill_city": null,
"bill_state": null,
"bill_postcode": null,
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"terms_id": 2,
"class_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"custom_7": null,
"custom_8": null,
"custom_9": null,
"custom_10": null,
"custom_11": null,
"custom_12": null,
"custom_13": null,
"custom_14": null,
"custom_15": null,
"custom_16": null,
"custom_17": null,
"custom_18": null,
"custom_19": null,
"custom_20": null,
"admin_id": null,
"campaign_id": null,
"currency_id": 1,
"language_id": 1,
"brand_id": 1,
"default_paymethod": null,
"default_creditcard_id": null,
"default_ach_id": null,
"tax_ident": "",
"no_taxes": false,
"no_dunning": false,
"no_latefees": false,
"write_datetime": "2023-03-23 11:48:48",
"write_ipaddr": "172.29.0.1",
"mod_datetime": "2023-03-23 11:48:48",
"mod_ipaddr": "172.29.0.1",
"terms_name": "Net 30",
"terms_days": 30,
"class_name": "",
"paid": 0,
"total": 0,
"balance": 0,
"url_statementlink": "https:\/\/dev1.chargeover.test\/r\/statement\/view\/d07dup6w8h61",
"url_paymethodlink": "https:\/\/dev1.chargeover.test\/r\/paymethod\/i\/d07dup6w8h61",
"url_self": "https:\/\/dev1.chargeover.test\/admin\/r\/customer\/view\/307",
"admin_name": "",
"admin_email": "",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "Test Customer",
"ship_block": "",
"bill_block": "United States",
"superuser_name": "Test Customer",
"superuser_first_name": "Test",
"superuser_last_name": "Customer",
"superuser_phone": null,
"superuser_email": null,
"superuser_token": "x0ka5029tise",
"customer_id": 307,
"parent_customer_id": null,
"invoice_delivery": "email",
"dunning_delivery": "email",
"customer_status_id": 1,
"mrr": 0,
"arr": 0,
"customer_status_name": "Current",
"customer_status_str": "active-current",
"customer_status_state": "a",
"superuser_username": "G2BXWw6NOChK"
},
"user": {
"user_id": 295,
"external_key": null,
"first_name": "Test",
"middle_name_glob": null,
"last_name": "Customer",
"name_suffix": null,
"title": null,
"email": null,
"token": "x0ka5029tise",
"phone": null,
"mobile": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"user_type_id": 1,
"write_datetime": "2023-03-23 11:48:49",
"mod_datetime": "2023-03-23 11:48:49",
"name": "Test Customer",
"display_as": "Test Customer",
"url_self": "https:\/\/dev1.chargeover.test\/admin\/r\/contact\/view\/295",
"user_type_name": "Billing",
"username": "G2BXWw6NOChK",
"password": null,
"customer_id": 307
}
},
"security_token": "a8T3OnpczQwvqgfZumVbrsYhBt0DEeS1"
}
A tokenized payment method is edited
This event occurs when a tokenized payment method is updated.
Note these fields:
context_str = tokenized
context_id = ...
(this will be thetokenized_id
of the tokenized payment method)event = update
A tokenized payment method is edited
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: a8T3OnpczQwvqgfZumVbrsYhBt0DEeS1
X-Chargeover-Source: example.chargeover.com
{
"context_str": "tokenized",
"context_id": "1",
"event": "update",
"data": {
"tokenized": {
"tokenized_id": 1,
"type": "",
"name": "Tokenized CC",
"token": "oaseihsndkrrn12i34ho",
"expdate": null,
"type_hint": null,
"paymethod_hint": "",
"write_datetime": "2023-03-23 11:49:15",
"write_ipaddr": "172.29.0.1",
"mod_datetime": "2023-03-23 12:18:53",
"mod_ipaddr": "172.29.0.1",
"customer_id": 307
},
"customer": {
"superuser_id": 295,
"external_key": null,
"token": "d07dup6w8h61",
"company": "Test Customer",
"bill_addr1": null,
"bill_addr2": null,
"bill_addr3": null,
"bill_city": null,
"bill_state": null,
"bill_postcode": null,
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"terms_id": 2,
"class_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"custom_7": null,
"custom_8": null,
"custom_9": null,
"custom_10": null,
"custom_11": null,
"custom_12": null,
"custom_13": null,
"custom_14": null,
"custom_15": null,
"custom_16": null,
"custom_17": null,
"custom_18": null,
"custom_19": null,
"custom_20": null,
"admin_id": null,
"campaign_id": null,
"currency_id": 1,
"language_id": 1,
"brand_id": 1,
"default_paymethod": null,
"default_creditcard_id": null,
"default_ach_id": null,
"tax_ident": "",
"no_taxes": false,
"no_dunning": false,
"no_latefees": false,
"write_datetime": "2023-03-23 11:48:48",
"write_ipaddr": "172.29.0.1",
"mod_datetime": "2023-03-23 11:48:48",
"mod_ipaddr": "172.29.0.1",
"terms_name": "Net 30",
"terms_days": 30,
"class_name": "",
"paid": 0,
"total": 0,
"balance": 0,
"url_statementlink": "https:\/\/dev1.chargeover.test\/r\/statement\/view\/d07dup6w8h61",
"url_paymethodlink": "https:\/\/dev1.chargeover.test\/r\/paymethod\/i\/d07dup6w8h61",
"url_self": "https:\/\/dev1.chargeover.test\/admin\/r\/customer\/view\/307",
"admin_name": "",
"admin_email": "",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "Test Customer",
"ship_block": "",
"bill_block": "United States",
"superuser_name": "Test Customer",
"superuser_first_name": "Test",
"superuser_last_name": "Customer",
"superuser_phone": null,
"superuser_email": null,
"superuser_token": "x0ka5029tise",
"customer_id": 307,
"parent_customer_id": null,
"invoice_delivery": "email",
"dunning_delivery": "email",
"customer_status_id": 1,
"mrr": 0,
"arr": 0,
"customer_status_name": "Current",
"customer_status_str": "active-current",
"customer_status_state": "a",
"superuser_username": "G2BXWw6NOChK"
},
"user": {
"user_id": 295,
"external_key": null,
"first_name": "Test",
"middle_name_glob": null,
"last_name": "Customer",
"name_suffix": null,
"title": null,
"email": null,
"token": "x0ka5029tise",
"phone": null,
"mobile": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"user_type_id": 1,
"write_datetime": "2023-03-23 11:48:49",
"mod_datetime": "2023-03-23 11:48:49",
"name": "Test Customer",
"display_as": "Test Customer",
"url_self": "https:\/\/dev1.chargeover.test\/admin\/r\/contact\/view\/295",
"user_type_name": "Billing",
"username": "G2BXWw6NOChK",
"password": null,
"customer_id": 307
}
},
"security_token": "a8T3OnpczQwvqgfZumVbrsYhBt0DEeS1"
}
A tokenized payment method is deleted
This event occurs when a tokenized payment method is deleted.
Note these fields:
context_str = tokenized
context_id = ...
(this will be thetokenized_id
of the tokenized payment method)event = delete
A tokenized payment method is deleted
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: a8T3OnpczQwvqgfZumVbrsYhBt0DEeS1
X-Chargeover-Source: example.chargeover.com
{
"context_str": "tokenized",
"context_id": "2",
"event": "delete",
"data": {
"tokenized": {
"tokenized_id": 2,
"type": "",
"name": "Tokenized ACH",
"token": "asldiruesknjfis",
"expdate": null,
"type_hint": null,
"paymethod_hint": null,
"write_datetime": "2023-03-23 12:27:12",
"mod_datetime": "2023-03-23 12:27:12",
"customer_id": 307
},
"customer": {
"superuser_id": 295,
"external_key": null,
"token": "d07dup6w8h61",
"company": "Test Customer",
"bill_addr1": null,
"bill_addr2": null,
"bill_addr3": null,
"bill_city": null,
"bill_state": null,
"bill_postcode": null,
"bill_country": "United States",
"bill_notes": null,
"ship_addr1": null,
"ship_addr2": null,
"ship_addr3": null,
"ship_city": null,
"ship_state": null,
"ship_postcode": null,
"ship_country": null,
"ship_notes": null,
"terms_id": 2,
"class_id": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"custom_6": null,
"custom_7": null,
"custom_8": null,
"custom_9": null,
"custom_10": null,
"custom_11": null,
"custom_12": null,
"custom_13": null,
"custom_14": null,
"custom_15": null,
"custom_16": null,
"custom_17": null,
"custom_18": null,
"custom_19": null,
"custom_20": null,
"admin_id": null,
"campaign_id": null,
"currency_id": 1,
"language_id": 1,
"brand_id": 1,
"default_paymethod": null,
"default_creditcard_id": null,
"default_ach_id": null,
"tax_ident": "",
"no_taxes": false,
"no_dunning": false,
"no_latefees": false,
"write_datetime": "2023-03-23 11:48:48",
"write_ipaddr": "172.29.0.1",
"mod_datetime": "2023-03-23 11:48:48",
"mod_ipaddr": "172.29.0.1",
"terms_name": "Net 30",
"terms_days": 30,
"class_name": "",
"paid": 0,
"total": 0,
"balance": 0,
"url_statementlink": "https:\/\/dev1.chargeover.test\/r\/statement\/view\/d07dup6w8h61",
"url_paymethodlink": "https:\/\/dev1.chargeover.test\/r\/paymethod\/i\/d07dup6w8h61",
"url_self": "https:\/\/dev1.chargeover.test\/admin\/r\/customer\/view\/307",
"admin_name": "",
"admin_email": "",
"currency_symbol": "$",
"currency_iso4217": "USD",
"display_as": "Test Customer",
"ship_block": "",
"bill_block": "United States",
"superuser_name": "Test Customer",
"superuser_first_name": "Test",
"superuser_last_name": "Customer",
"superuser_phone": null,
"superuser_email": null,
"superuser_token": "x0ka5029tise",
"customer_id": 307,
"parent_customer_id": null,
"invoice_delivery": "email",
"dunning_delivery": "email",
"customer_status_id": 1,
"mrr": 0,
"arr": 0,
"customer_status_name": "Current",
"customer_status_str": "active-current",
"customer_status_state": "a",
"superuser_username": "G2BXWw6NOChK"
},
"user": {
"user_id": 295,
"external_key": null,
"first_name": "Test",
"middle_name_glob": null,
"last_name": "Customer",
"name_suffix": null,
"title": null,
"email": null,
"token": "x0ka5029tise",
"phone": null,
"mobile": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"user_type_id": 1,
"write_datetime": "2023-03-23 11:48:49",
"mod_datetime": "2023-03-23 11:48:49",
"name": "Test Customer",
"display_as": "Test Customer",
"url_self": "https:\/\/dev1.chargeover.test\/admin\/r\/contact\/view\/295",
"user_type_name": "Billing",
"username": "G2BXWw6NOChK",
"password": null,
"customer_id": 307
}
},
"security_token": "a8T3OnpczQwvqgfZumVbrsYhBt0DEeS1"
}
Admin Users
Admin
An admin is created
This event occurs when a new admin is created.
Note these fields:
context_str = admin
context_id = ...
(this will be theadmin_id
of the new user)event = insert
An admin is created
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: a8T3OnpczQwvqgfZumVbrsYhBt0DEeS1
X-Chargeover-Source: example.chargeover.com
{
"context_str": "admin",
"context_id": 5,
"event": "insert",
"data": {
"admin": {
"admin_id": 5,
"external_key": null,
"timezone": null,
"first_name": "Test",
"last_name": "Admin",
"initials": null,
"nickname": "TestAdmin",
"email": "test@email.com",
"twitter": null,
"facebook": null,
"linkedin": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"login_count": "0",
"login_datetime": null,
"write_datetime": "2023-03-23 12:54:45",
"mod_datetime": "2023-03-23 12:54:45",
"name": "Test Admin",
"username": "test@email.com"
}
},
"security_token": "a8T3OnpczQwvqgfZumVbrsYhBt0DEeS1"
}
An admin is edited
This event occurs when a new admin is updated.
Note these fields:
context_str = admin
context_id = ...
(this will be theadmin_id
of the new user)event = update
An admin is edited
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: a8T3OnpczQwvqgfZumVbrsYhBt0DEeS1
X-Chargeover-Source: example.chargeover.com
{
"context_str": "admin",
"context_id": 5,
"event": "update",
"data": {
"admin": {
"admin_id": 5,
"external_key": null,
"timezone": "",
"first_name": "Test",
"last_name": "Admin Edit",
"initials": null,
"nickname": "TestAdmin",
"email": "test@email.com",
"twitter": null,
"facebook": null,
"linkedin": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"login_count": "0",
"login_datetime": null,
"write_datetime": "2023-03-23 12:54:45",
"mod_datetime": "2023-03-23 13:01:32",
"name": "Test Admin Edit",
"username": "test@email.com"
}
},
"security_token": "a8T3OnpczQwvqgfZumVbrsYhBt0DEeS1"
}
An admin is deleted
This event occurs when an admin is deleted.
Note these fields:
context_str = admin
context_id = ...
(this will be theadmin_id
of the new user)event = delete
An admin is deleted
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: a8T3OnpczQwvqgfZumVbrsYhBt0DEeS1
X-Chargeover-Source: example.chargeover.com
{
"context_str": "admin",
"context_id": 5,
"event": "delete",
"data": {
"admin": {
"admin_id": 5,
"external_key": null,
"timezone": "",
"first_name": "Test",
"last_name": "Admin Edit",
"initials": null,
"nickname": "TestAdmin",
"email": "test@email.com-1679594810.402",
"twitter": null,
"facebook": null,
"linkedin": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"login_count": "0",
"login_datetime": null,
"write_datetime": "2023-03-23 12:54:45",
"mod_datetime": "2023-03-23 13:01:32",
"name": "Test Admin Edit",
"username": "test@email.com-1679594810.402"
}
},
"security_token": "a8T3OnpczQwvqgfZumVbrsYhBt0DEeS1"
}
An admin logged into ChargeOver
This event occurs when an admin logs into ChargeOver.
Note these fields:
context_str = admin
context_id = ...
(this will be theadmin_id
of the new user)event = login
An admin logged into ChargeOver
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: a8T3OnpczQwvqgfZumVbrsYhBt0DEeS1
X-Chargeover-Source: example.chargeover.com
{
"context_str": "admin",
"context_id": 1,
"event": "login",
"data": {
"admin": {
"admin_id": 1,
"external_key": null,
"timezone": null,
"first_name": "Test",
"last_name": "Admin",
"initials": null,
"nickname": null,
"email": "test@email.com",
"twitter": null,
"facebook": null,
"linkedin": null,
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"login_count": "149",
"login_datetime": "2023-03-23 12:45:45",
"write_datetime": "2022-08-17 09:22:21",
"mod_datetime": "2023-02-27 15:47:07",
"name": "Test Admin",
"username": "test@email.com"
}
},
"security_token": "a8T3OnpczQwvqgfZumVbrsYhBt0DEeS1"
}
Notes
Notes
A note created
This event occurs when a new note is created.
Note these fields:
context_str = note
context_id = ...
(this will be thenote_id
of the new note)event = insert
A note created
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: abcd1234
X-Chargeover-Source: example.chargeover.com
{
"context_str": "note",
"context_id": 9,
"event": "insert",
"data": {
"note": {
"note_id": 9,
"user_id": null,
"admin_id": 1,
"note_is_pinned": false,
"note_datetime": "2024-02-16 08:16:34",
"note": "Customer requested upgrade to PRO plan via email",
"context_str": "customer",
"context_id": 265
}
},
"security_token": "abcd1234"
}
REST Hooks
ChargeOver also supports the REST Hooks <RESTHooks.org> pattern for subscribe/unsubscribe scenarios.
REST Hooks is a pattern designed to make it easy for third-party applications to subscribe to specific events within the ChargeOver platform, and receive webhooks whenever those events occur.
Subscribe
Subscribing is the process of telling ChargeOver to send webhooks to you whenever a specific event occurs (example: "Send http://example.org/my_webhook_url data whenever a customer is created."). Once you've subscribed, each time the event occurs within ChargeOver, ChargeOver will send a HTTP POST
request to your URL with a JSON payload of the event data.
Subscribing happens via a REST API call.
Please see our REST API documentation for an example of this.
Unsubscribe
Unsubscribing is the process of telling ChargeOver to stop sending webhooks to a specific URL.
Unsubscribing happens via a REST API call.
Please see our REST API documentation for an example of this.
Reverse Unsubscribe
Instead of making a REST API call to unsubscribe, your unsubscribe URL can also respond to a webhook with a HTTP 410 Gone
status. This will cause ChargeOver to automatically unsubscribe the URL.
Quick Reference List
Below is a quick reference table for supported events.
Need help or don't see what you're looking for?
Reference List
Customers
Object - context_str
|
Event - event
|
Description | |
---|---|---|---|
customer | insert | A customer is created | 📎 |
customer | update | A customer is updated | 📎 |
customer | join | A contact is added to the customer | 📎 |
customer | super | The main contact is changed | 📎 |
customer | delete | A customer is deleted | 📎 |
customer | nightly | The nightly event occurs | 📎 |
customer | status | A customer status has changed | 📎 |
Users (Contacts)
Object - context_str
|
Event - event
|
Description | |
---|---|---|---|
user | insert | A user is created | 📎 |
user | update | A user is updated | 📎 |
user | delete | A user is deleted | 📎 |
Quotes
Object - context_str
|
Event - event
|
Description | |
---|---|---|---|
quote | status | A quote status has changed | 📎 |
quote | insert | A quote is created | 📎 |
quote | update | A quote is updated | 📎 |
quote | nightly | The nightly event occurs | 📎 |
quote | won | A quote is accepted | 📎 |
quote | lost | A quote is rejected | 📎 |
Invoices
Object - context_str
|
Event - event
|
Description | |
---|---|---|---|
invoice | status | An invoice status has changed | 📎 |
invoice | insert | An invoice is created | 📎 |
invoice | update | An invoice is updated | 📎 |
invoice | void | An invoice is voided | 📎 |
invoice | nightly | The nightly event occurs | 📎 |
Subscriptions (Packages)
Object - context_str
|
Event - event
|
Description | |
---|---|---|---|
package | status | A subscription status has changed | 📎 |
package | insert | A subscription is created | 📎 |
package | update | A subscription is updated | 📎 |
package | generate | A subscription has generated an invoice | 📎 |
package | paycycle | The invoice cycle for a subscription changes | 📎 |
package | paymethod | The pay method for a subscription changes | 📎 |
package | cancel | A subscription is cancelled | 📎 |
package | updowngrade | A subscription is upgraded or downgraded | 📎 |
package | suspend | A subscription is suspended | 📎 |
package | unsuspend | A subscription is unsuspended | 📎 |
package | nightly | The nightly event occurs | 📎 |
package | hold | The subscription invoice generation is delayed | 📎 |
package | uncancel | The cancelled subscription is un-canceled | 📎 |
Items (Products, Discounts)
Object - context_str
|
Event - event
|
Description | |
---|---|---|---|
item | insert | An item is created | 📎 |
item | update | An item is updated | 📎 |
Transactions (Payments, Refunds, Credits)
Object - context_str
|
Event - event
|
Description | |
---|---|---|---|
transaction | status | A transaction status has changed | 📎 |
transaction | insert | A transaction is created | 📎 |
transaction | apply | A transaction is applied to an invoice | 📎 |
transaction | void | A transaction is marked void | 📎 |
ACH/eChecks
Object - context_str
|
Event - event
|
Description | |
---|---|---|---|
ach | insert | An ACH/eCheck account created | 📎 |
ach | update | ACH/eCheck account updated | 📎 |
ach | delete | ACH/eCheck account deleted | 📎 |
ach | autopay | ACH/eCheck account approved for autopay | 📎 |
Credit Cards
Object - context_str
|
Event - event
|
Description | |
---|---|---|---|
creditcard | insert | Credit card created | 📎 |
creditcard | update | Credt card updated | 📎 |
creditcard | delete | Credit card deleted | 📎 |
creditcard | expiring | Credit card is expiring soon | 📎 |
creditcard | expired | Credit card has expired | 📎 |
creditcard | autopay | Credit card has been approved for autopay | 📎 |
Tokenized Payment Methods
Object - context_str
|
Event - event
|
Description | |
---|---|---|---|
tokenized | insert | A tokenized payment method is created | 📎 |
tokenized | update | A tokenized payment method is edited | 📎 |
tokenized | delete | A tokenized payment method is deleted | 📎 |
Admin Users
Object - context_str
|
Event - event
|
Description | |
---|---|---|---|
admin | insert | An admin is created | 📎 |
admin | update | An admin is edited | 📎 |
admin | delete | An admin is deleted | 📎 |
admin | login | An admin logged into ChargeOver | 📎 |
Notes
Object - context_str
|
Event - event
|
Description | |
---|---|---|---|
note | insert | A note created | 📎 |
Best Practices
Verifying / validating webhooks
ChargeOver sends data within webhooks that you can use to verify that the webhook is from ChargeOver rather than from another source.
When you configure webhooks in your ChargeOver account, you will see a field for your "Webhook/callback secret token or encryption key." When you recieve a webhook from ChargeOver, you will be able to see an identical token/key to the one generated in your account in two places within the webhook itself:
- The
security_token
embedded in the JSON request of each webhook - This value is also sent in a HTTP header called
X-ChargeOver-Key
Locating your security key/token in a webhook
The security_token
and the X-ChargeOver-Key
can be seen in the example to the right.
Locating your security key/token in a webhook
Webhook
POST /path/to/your/endpoint HTTP/1.1
Content-Type: application/json
X-ChargeOver-Key: SFkJ3fy02n17YxNXm96tCGUKVTOAIBsH
X-Chargeover-Source: example.chargeover.com
{
"context_str": "user",
"context_id": 370,
"event": "insert",
"data": {
"user": {
"user_id": 770,
"external_key": null,
"first_name": "Denise",
"middle_name_glob": null,
"last_name": "Wong",
"name_suffix": null,
"title": "",
"email": "denise.k.wong@example.com",
"token": "04lukh1f05rp",
"phone": "403-568-4968",
"custom_1": null,
"custom_2": null,
"custom_3": null,
"custom_4": null,
"custom_5": null,
"user_type_id": 1,
"write_datetime": "2017-08-18 13:43:42",
"mod_datetime": "2017-10-05 17:51:22",
"name": "Denise Wong",
"display_as": "Denise Wong",
"url_self": "https:\/\/karlitestaccount.chargeover.com\/admin\/r\/contact\/view\/770",
"user_type_name": "Billing",
"username": "hf20iutg6d9m",
"customer_id": 413
}
},
"security_token": "SFkJ3fy02n17YxNXm96tCGUKVTOAIBsH"
}
Troubleshooting
ChargeOver provides a log of every webhook that is fired. The Webhook Log report contains this information:
For development purposes, you can force ChargeOver to log the full webhook payload by returning a 201 Created response instead of a 200 OK. This will cause ChargeOver to log the full payload, but not retry.
Order of events
ChargeOver tries to send webhooks as quickly as possible, so that your application can be notified of events happening within ChargeOver in near real-time.
However, due to the potential for network errors, retries, etc., it is not guaranteed that you will receive events immediately (there may be a delay between when something happens in ChargeOver, and when you receive the webhook), and it is not guaranteed that you will receive events in exactly the order they were triggered (you may receive events out of order).
Your application should handle events in an idempotent manner, so that events received out of order do not negatively impact your integration.
Network errors, timeouts, etc.
There is a 14 second timeout for webhooks.
If the URL ChargeOver is sending the webhook to takes longer than 14 seconds to respond with a 200 OK
,
ChargeOver will close the HTTP connection and mark the webhook as failed.
If your site takes a long time to process webhooks, consider using a background queue to queue up what needs to be
processed so that you can return a 200 OK
immediately to ChargeOver.